How to Create a jQuery Confirm Dialog Replacement
Continuing on our quest for building customizable alternatives for browser's controls, this week we are building a cross-browser, custom confirm dialog in the form of an easy to use jQuery plugin. You can choose the text, buttons, and actions that will be executed when they are clicked.
HTML
Although we are mainly concentrating on the confirmation dialog, lets first say a few words about the page on which we are going to use it. If you are eager to see the plugin's source code, you can skip this step and scroll down to the jQuery part of the tutorial.
index.php
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>A jQuery Confirm Dialog Replacement with CSS3 | Tutorialzine Demo</title> <!-- Including the Cuprum font with @font-face from Google's webfont API --> <link href='http://fonts.googleapis.com/css?family=Cuprum&subset=latin' rel='stylesheet' type='text/css'> <link rel="stylesheet" type="text/css" href="css/styles.css" /> <link rel="stylesheet" type="text/css" href="jquery.confirm/jquery.confirm.css" /> </head> <body> <div id="page"> <div class="item"> <a href="https://tutorialzine.com/?p=1274"> <img src="https://tutorialzine.com/img/featured/1274.jpg" title="Coding a Rotating Image Slideshow w/ CSS3 and jQuery" alt="Coding a Rotating Image Slideshow w/ CSS3 and jQuery" width="620" height="340" /> </a> <div class="delete"></div> </div> <!-- Other "item" divs --> </div> <!-- Including jQuery and our jQuery Confirm plugin --> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.4/jquery.min.js"></script> <script src="jquery.confirm/jquery.confirm.js"></script> <script src="js/script.js"></script> </body> </html>
In the head section of the document, I am including the Cuprum font from Google's Font Directory, jquery.confirm.css, which is the stylesheet for the confirm dialog, and styles.css, which styles the example page.
At the bottom of the body section, I've included the jQuery library, jquery.confirm.js, which is the main plugin file, and script.js, which listens for the click event on the example page uses fires up the plugin. These last two files are discussed in the final step of this tutorial.
To include the confirm dialog in your website, you need to copy the jquery.confirm folder from the zip download, and include jquery.confirm.css in the head, and jquery.confirm.js before the closing body tag of your pages, along with the jQuery library.
The dialog box itself is nothing more than a few lines of HTML. Bellow you can see the code that is inserted by the plugin to display the confirm window.
Example code for the dialog
<div id="confirmOverlay"> <div id="confirmBox"> <h1>Title of the confirm dialog</h1> <p>Description of what is about to happen</p> <div id="confirmButtons"> <a class="button blue" href="#">Yes<span></span></a> <a class="button gray" href="#">No<span></span></a> </div> </div> </div>
This code is appended to the body of the document. The confirmOverlay is displayed over the rest of the page, preventing any interactions with it while the dialog is visible (modal behavior). The h1, p and the confirmButtons div are populated according to the arguments you pass to the plugin. You will read more on that later on in this article.
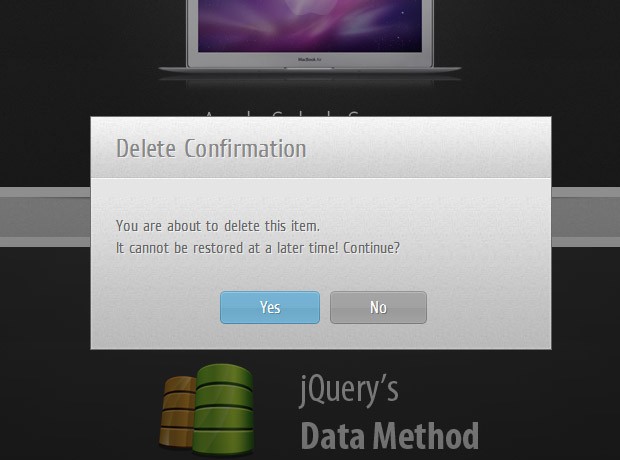
CSS
The styling of the confirm dialog is contained in jquery.confirm.css. This makes it easier to include into an existing project, and it is built in such a way, that you can be sure it will not clash with the rest of your page styles.
jquery.confirm.css
#confirmOverlay{ width:100%; height:100%; position:fixed; top:0; left:0; background:url('ie.png'); background: -moz-linear-gradient(rgba(11,11,11,0.1), rgba(11,11,11,0.6)) repeat-x rgba(11,11,11,0.2); background:-webkit-gradient(linear, 0% 0%, 0% 100%, from(rgba(11,11,11,0.1)), to(rgba(11,11,11,0.6))) repeat-x rgba(11,11,11,0.2); z-index:100000; } #confirmBox{ background:url('body_bg.jpg') repeat-x left bottom #e5e5e5; width:460px; position:fixed; left:50%; top:50%; margin:-130px 0 0 -230px; border: 1px solid rgba(33, 33, 33, 0.6); -moz-box-shadow: 0 0 2px rgba(255, 255, 255, 0.6) inset; -webkit-box-shadow: 0 0 2px rgba(255, 255, 255, 0.6) inset; box-shadow: 0 0 2px rgba(255, 255, 255, 0.6) inset; } #confirmBox h1, #confirmBox p{ font:26px/1 'Cuprum','Lucida Sans Unicode', 'Lucida Grande', sans-serif; background:url('header_bg.jpg') repeat-x left bottom #f5f5f5; padding: 18px 25px; text-shadow: 1px 1px 0 rgba(255, 255, 255, 0.6); color:#666; } #confirmBox h1{ letter-spacing:0.3px; color:#888; } #confirmBox p{ background:none; font-size:16px; line-height:1.4; padding-top: 35px; } #confirmButtons{ padding:15px 0 25px; text-align:center; } #confirmBox .button{ display:inline-block; background:url('buttons.png') no-repeat; color:white; position:relative; height: 33px; font:17px/33px 'Cuprum','Lucida Sans Unicode', 'Lucida Grande', sans-serif; margin-right: 15px; padding: 0 35px 0 40px; text-decoration:none; border:none; } #confirmBox .button:last-child{ margin-right:0;} #confirmBox .button span{ position:absolute; top:0; right:-5px; background:url('buttons.png') no-repeat; width:5px; height:33px } #confirmBox .blue{ background-position:left top;text-shadow:1px 1px 0 #5889a2;} #confirmBox .blue span{ background-position:-195px 0;} #confirmBox .blue:hover{ background-position:left bottom;} #confirmBox .blue:hover span{ background-position:-195px bottom;} #confirmBox .gray{ background-position:-200px top;text-shadow:1px 1px 0 #707070;} #confirmBox .gray span{ background-position:-395px 0;} #confirmBox .gray:hover{ background-position:-200px bottom;} #confirmBox .gray:hover span{ background-position:-395px bottom;}
A handful of CSS3 declarations are used. In the #confirmOverlay definition, we are using CSS3 gradients (only available in Firefox, Safari and Chrome for now), with a transparent PNG fallback for the rest.
Later, in the #confirmBox, which is centered in the middle of the screen, I've added an inset box-shadow, which is in effect a highlight (think of Photoshop's inner glow). Also I've used the Cuprum font, which was included from Google's Font Directory.
Along with some text shadows, you can see the styling of the buttons. They are built using the sliding doors technique. Currently two designs are available - blue and gray. You can add a new button color by specifying additional declarations.
jQuery
Before moving to the source code of the plugin, lets first see what we are after. In script.js you can see how the plugin is called.
script.js
$(document).ready(function(){ $('.item .delete').click(function(){ var elem = $(this).closest('.item'); $.confirm({ 'title' : 'Delete Confirmation', 'message' : 'You are about to delete this item. <br />It cannot be restored at a later time! Continue?', 'buttons' : { 'Yes' : { 'class' : 'blue', 'action': function(){ elem.slideUp(); } }, 'No' : { 'class' : 'gray', 'action': function(){} // Nothing to do in this case. You can as well omit the action property. } } }); }); });
When the .delete div is clicked in our example page, the script is executes the $.confirm function, defined by our plugin. It then passes the title of the dialog, the description, and an object with the buttons. Each button takes the name of a CSS class and an action property. This action is the function that is going to be executed when the button is clicked.
Now lets move to the interesting part. In jquery.confirm.js you can see the source code of our confirm dialog alternative.
jquery.confirm.js
(function($){ $.confirm = function(params){ if($('#confirmOverlay').length){ // A confirm is already shown on the page: return false; } var buttonHTML = ''; $.each(params.buttons,function(name,obj){ // Generating the markup for the buttons: buttonHTML += '<a href="#" class="button '+obj['class']+'">'+name+'<span></span></a>'; if(!obj.action){ obj.action = function(){}; } }); var markup = [ '<div id="confirmOverlay">', '<div id="confirmBox">', '<h1>',params.title,'</h1>', '<p>',params.message,'</p>', '<div id="confirmButtons">', buttonHTML, '</div></div></div>' ].join(''); $(markup).hide().appendTo('body').fadeIn(); var buttons = $('#confirmBox .button'), i = 0; $.each(params.buttons,function(name,obj){ buttons.eq(i++).click(function(){ // Calling the action attribute when a // click occurs, and hiding the confirm. obj.action(); $.confirm.hide(); return false; }); }); } $.confirm.hide = function(){ $('#confirmOverlay').fadeOut(function(){ $(this).remove(); }); } })(jQuery);
Our plugin defines the $.confirm() method. What it does, is read the arguments you've passed, construct the markup, and then add it to the page. As the #confirmOverlay div is assigned a fixed positioning in its CSS declaration, we can leave it to the browser to center it on the screen and move it when the visitor scrolls the page.
After adding the markup, the script assigns event handlers for the click events, executing the action parameter for the respective button.
With this our jQuery-powered Confirm Dialog is complete!
Wrapping it up
You can customize the appearance of the dialog by modifying jquery.confirm.css. As the message attribute of the dialog takes HTML text, you can further customize it by displaying images and icons in the confirm window.
You can even alternatively use this plugin as an alert dialog - you will just need to pass a single button with no action attribute.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
i am loving this..will definitely put it to use. Thank you!
Very well done ! This helped me a lot. Thanks !
Ohh love it . Many thanks for tutorial
Incredible! I loved this tutorial.
As always, such a nice tutorial. Keep up the good work.
Amazing. Really nice work..
This would be most awesome if it overrode calls to Javascript's native confirm dialog, so any call to 'confirm' instead calls this one. That way you don't need to change existing scripts. Otherwise I'd prefer jQuery UI's dialog plugin because it has more options.
Unfortunately this is not possible. When a regular confirm is opened, it blocks the script until the user chooses an option, and then returns true/false.
jQuery UI's dialog is always an option, but sometimes you want something simple and lightweight.
Great work as usually ! Thanks !
Thank you for the comments, folks! Glad you like the script.
hey Martin, Great job!
is there a way to TAB and ESC keys on this confirmation box?
thanks!
great work brother, it was really nice..
hehehe I delete all the demo ... xixixixi
can be like it, its a cool
cheers \m/
Really well done! (code and design)
Loving the design, nice and clean! Thanks for sharing
Really well done! I was looking for something like this for a upcoming project.
Thanks for the Tut + Snippet. Looks really nice.
This is exactly what I've been looking for, thanks!
Looks pretty sweet.
Nice work but your script doesn't support using TAB key :/
Well done !!! Thank you for helpful and useful code...
Code, design are just awesome. (y)
This is awesome! Just what I'm looking for. However, I've applied it to a link which redirects the user away from the site, thus the need of the confirm box to inform the user that they're going to be redirected.
However, before the modal window completely loads (set to fast too), the page redirects to the href that was on the clicked link.
Is there any way to make it perform just like a confirm box, where the page doesn't do anything until a button is clicked?
Thanks for the killer code!
Here is my code that may help some of you...The first is a dialogue box for delete confirmation that fires after the user clicks the delete button. This resides in an array so you see the underscore_ID. A simple yes or no. If yes, sends a variable to the delete php script...The second, is an ajax call that updates the record and then opens the dialogue box for 3 seconds and then closes. Good luck
$(document).ready(function(){
});
$(document).ready(function(){
$(".update").click(function(event){
var price = $('#price').val();
var featureID = $('#featureID_').val();
if(price == "" ){
$("#alert ").slideDown("slow");
}
else{
jQuery.ajax({
type: "POST",
url: "Omitted for security",
data: 'price='+ price + '&featureID='+ featureID,
cache: false,
success: function(html)
{
$('#price').val('');
$("#oldPrice").slideUp("slow");
$("#newprice_").html(html).show();
setTimeout(function(){
$("#confirmOverlay").fadeOut("slow", function () {
$("#confirmOverlay").remove();
});
}, 2000);
$.confirm({
'title' : 'Giddy up! You updated this feature',
'message' : 'Series: - Feature Code: : ',
'buttons' : {
'Close this window' : {
'class' : 'blue',
'action': function(){}
}
}
});
}return true;
});
});
very clean script, thanks a lot
Thanks for that amazing plugin. Just one addition, i need to send some data via ajax and post back results (put results on the Confirm) after confirmation. If data is posted back rapidly, confirm box doesn't show the results bacause of current doesn't removed yet,
if($('#confirmOverlay').length){
// A confirm is already shown on the page:
return false;
}
but i need to show both consecutively, so i changed pugin a bit like that;
buttons.eq(i++).click(function(){
line becomes
buttons.eq(i++).bind('click',function(){
(because i'll unbind below) and
if($('#confirmOverlay').length){
// A confirm is already shown on the page:
return false;
}
bart becomes;
if($('#confirmOverlay').length){
// A confirm is already shown on the page so unbind all click events for preventing from previous clicks and remove for new ones' adding.
$('#confirmBox .button').unbind('click').remove();
}
As a result, when you execute a Confirmbox while previous still on the screen, will toggle from prev to next.
Hi Alper,
I have exactly the same problem. I tried your solution, but it still does not work. Just wondering if you have any other solution for this problem?
Ed
Hi Ed, sorry for late reply..
I've found a solution, it flicks the overlay but working.
replace
$('#confirmBox .button').unbind('click').remove();
part with
Just an idea ;)
It flicks because remove() fireed rapidly.
A long time has passed and I've been using this for quite a while, but I ran into the same race condition today. I decided to fix it just by removing the fadeOut() call. Seems to work robustly. I like this simple and elegant plug-in a lot.
Awesome
Uhm, im kinda new with jQuery and Javascript, but im now using an jqueryUI model window withan Apple like style skin.
I would really like to use this one, but it also has to make a AJAX request to a file so it will delete it from the database or something.
Can someone help my with this? Of give me tips/hints?
Thanx, and really nicely done with the design! It's freaking awsome!
Exactly what i was looking for. Thanks :) Jquery rocks.
Can you please show me some mercy?
I have worked on this all day. I am a very experienced web developer, but I cannot make this even begin to work - it wont show the Delete button.
In the end I copied your example page to my local machine and just changed the path to the various .js and .cc files. I have had all day to quadruple check the paths and they are fine. It is just inert. Dead.
I used the files from your 'Download' link at the top of the page. What could possibly be wrong that it is so completely dead?
If you can help I would be immensely grateful - a day from your life lost is a lot of time after all!
Regards
Destroyed.
P.S.
Also, why does your demo flicker and then displace its dialogue box about 1 picvel up and one to the left as the shaded screen sets in, and the effect reverses if you cancel it. It makes the dialogue box flicker/jump slightly in a very disconcerting way.
Internet Explorer 8
Cheers
Destroyed.
Hi
I don't know if this is adaptable for a more basic purpose, but I just want a text link that says 'Delete' to trigger your confirm dialogue, and collect a return true or not. Nothing to do with deleting (hiding) the image on screen, putting up tool tips and all the extras. Is it a lot of work to make it operate at a more general level like this (stipping out tooltips and so on). I can't figure out where to start. a good clue would be, "what a href would I attach to the 'Delete' text to fire off the Confirm dialogue please?" - knowing that that would be a massive help.
Thank you
David
David,
This is what I use to add the confirmation dialog to a simple <a> link:
You need to use preventDefault() to keep the link from firing until you click the confirmation button. Then use $(this).attr("href") to direct the user's browser to the original link when they confirm.
hello Tom, I was looking at your comment, I have been looking for this for a while. i wanted to ask you; the window.location.href is going to a separate php file? and where exactly would you place the $(this).attr('href'); ? thanks for your help!
This is exactly what I needed thank you.
Is it possible to do the same to validate a form?
Great tutorial, thank you so much!
I have only one question, and maybe somebody here knows how to do it:
I know JS is client side and PHP is server side, but if I wanted a PHP function to be called when the “YES” button is clicked, what would be the best way to get it done?
Thank Again!
Actually, I do have to use this confirm as a part of form validation. It is not just one confirmation rather of multiple confirmation boxes which would to be displayed if the first one is confirmed as YES. But the problem is that I want this to halt the code execution until the user gives the choice YES or NO ffor the first confirm box. If user clicked YES then we may like to present the send Confirm Box based on form data conditions. Basically the same effect of havascript native confirm box but with custom buttons and style.
I do not get a success at all so far. Please advise if that can be possible at all.
Thanks in advance.
This plugin is very nice.
I am trying to use it but I think there is a problem with the 'yes' button.
If I have a regular old JavaScript prompt dialog inside the 'yes' action, the function executes, but the dialog doesn't go away?
Any thoughts on this?
Very nice plugin , solve my problem.
I added the following code to allow dismissal of the dialog box with the ESCAPE button.
AFTER:
$.confirm = function(params){
ADD:
$(document).keyup(function(e) {
var KEYCODE_ESC = 27;
if (e.keyCode == KEYCODE_ESC) { $.confirm.hide(); }
});
This was a huge help. I've incorporated it into a responsive design of mine, but I'm having trouble with it on Android devices (2.2). If you click the link that launches the dialog somewhere down a scrolled page, the overlay and message appear only in the top part of the page. It only fills up a section of the page that's the height of the viewport. You have to scroll up to actually see it. If you don't, you wouldn't even notice there was a dialog box. Any ideas on how to fix this?
Good work Mr. Angelov. Nice, simple and easy (and not too bloated like so many other addons/scripts)
:)
Beatifull, but I would prefer not having to set a function to run after the user press ok, this is not very good from a dont-repeat-yourself perspective, better would just to halt the execution if the user cancels, and if the users confirms let jQuery continue to whatever code is below.
Is it possible to use this method to trigger the execution of a form?
I have a list of users and when I click on the delete box opens and asks me to confirm the suppression of the user. If I click yes, the form must be executed.
Is this feasible?
thank alot
I'm a developer so I don't do much UI work. How can I get the buttons.png for pink and gray instead of blue and gray. We have certain styling guidelines we have to use and I can't use blue. Our pink color has to be #DE1C85. Thanks all the help is appreciated.
good sirr, Thanks for your tutorial
Dear, is there a way to enable the enter key to confirm any of these actions? Congratulations for the excellent code!
I have updated the script to handle Enter key. Just update the $.each function with "buttons[0].focus();"
Sometimes, you want to extend the functionality and add a form or something.
But you want it to be submited when the user clicks a button (ex. ajax).
But at the sime time you have to keep the dialog showing in case of error, to prompt the user.
For that, change line 43 from this:
into this:
If you return false on a function in the button, the dialog won't close!
If you simply want to skip this, don't return false, use return; or return undefined;
Yet another way to handle key codes:
Add this below line 47:
Add this to line 50:
The <enter> key will be "bound" to the 1st button and the <esc> to the LAST button (IF AVAILABLE).