A Quick Guide to Web Storage
This is a guest post by Baback Rashtizadeh, a freelance UI/UX designer and a front-end developer with passion for coding and writing.
Almost every desktop or mobile application needs to store user data somewhere. But how about a website? In the past, we used cookies for the purpose, but they have serious limitations. HTML5 gives us better tools to solve this problem. The first is IndexedDB, which is an overkill as a cookie replacement, and Web Storage, which is a combination of two very simple APIs and which I will be showing to you today.
What is the Web Storage?
Generally speaking, Web Storage (also known as Dom Storage) refers to a set of APIs that attempt to provide a simple way to store client side data in the browser. It is more secure and faster than cookies, not to mention more powerful. Data is stored in the user's browser and is not transferred across the network like cookies are. It is also possible to store larger amounts of data than cookies without affecting your website's performance.
Web Storage provides two awesome objects for storing data:
- localStorage: By using this object, you will store data without an expiration date, which means the data will be stored in the user's local storage forever;
- sessionStorage: By using this object, the data that you stored will be there until the visitor closes their browser (not the tab). A good use case for this is to save temporary data like the contents of a form the user is filling, in case they close the tab or reload the page accidentally.
Getting Started
So now that we know what Web Storage is, it is time to get our hands on it.
localStorage
It is very simple to save data to localStorage
- you only need to assign it as a property . Reading it is exactly as simple, as you can see in the example below:
localStorage.myText = 'This is some text which have been stored with localStorage object'; document.getElementById("text").innerHTML= localStorage.myText;
sessionStorage
Storing and retrieving data from sessionStorage
is done in the same way:
sessionStorage.myText = 'This is some text which have been stored with sessionStorage object'; document.getElementById("text").innerHTML= sessionStorage.myText;
Both objects have setItem()
, getItem()
and removeItem()
methods which you can also use:
localStorage.setItem('username','Johnny'); console.log(localStorage.getItem('username')); localStorage.removeItem('username'); // Johnny is no more!
You can also iterate them like regular objects, and check their length:
console.log(localStorage.length); for(var i in localStorage){ console.log(localStorage[i]);}
These are only the basics for using Web Storage, but they will be enough for you to implement the API in your web apps. There are more awesome things you can do with Web Storage, as you will see in in a moment.
Browser Support
As is usually the case with awesome HTML5 features, you have to look at the list of supported browsers before making use of it. Web Storage is supported by almost all the modern browsers including IE8+, so it is ready for use. Unfortunately IE7 and earlier versions of Internet Explorer don't support the APIs, so you will have to use one of the fallbacks mentioned below, if you want to support them.
Javascript Libraries for Web Storage
Here are some cool JavaScript libraries that take Web Storage to the next level:
basket.js
A simple (proof-of-concept) script loader that caches scripts with localStorage.
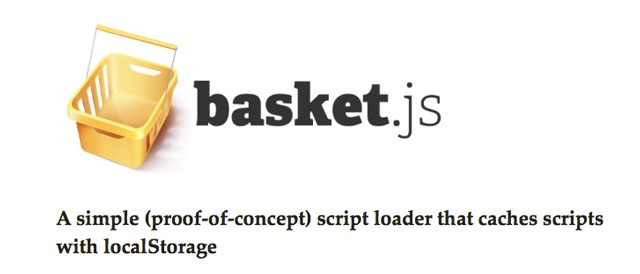
Kizzy
A light-weight, cross-browser, JavaScript local storage utility.
LocalDB.js
A tool that maps the structure of the databases in objects using the localStorage API.
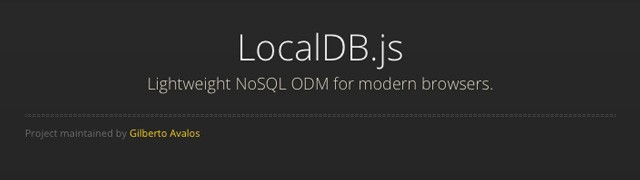
Rockstage.js
The JavaScript library for using localStorage and sessionStorage more easy.
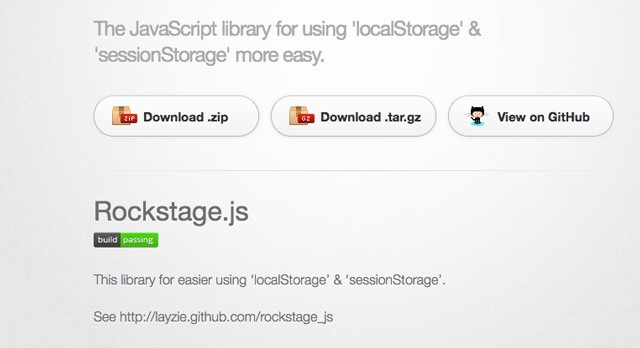
store.js
store.js exposes a simple API for cross-browser local storage.
Conclusion
Web Storage is a cool HTML5 feature and although it's been a while since its introduction, now we have lots of great libraries for it. Remember that this is just a quick guide and and there are still more things to learn about Web Storage, but I hope that this article gets you started on the right track!
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Thank you, article is excellent! I wonder something, if you storage a lot of data with this method, is the user's browser will be slow down?
Glad you like it, Haven't seen this issue yet! But I don't think using this method can be cause of slowing down the browser. Not sure BTW
@Yusuf : normally not :
"It is also possible to store larger amounts of data than cookies without affecting your website’s performance." Normally Browsers will have to find a way to carry the "huge" amount of data you want to use in your website, not you.
Nice article... thanks.
Can you use dot notation instead of getItem and setItem?
Thank you, article is excellent! :)
از اینکه دیدم یک هم وطن همچین مقاله ای اینجا نوشته بسیار خوشحال شدم.
موفق باشید
You can also use setItem on localStorage and sessionStorage.
Here is an example:
Thank you, this is a good example and a beautiful tutorial.