Quick Tip: Convert The Service Chooser App From Backbone.js to AngularJS
This is a guest post by Kevin P. a web developer and a reader of Tutorialzine, who suggested a better approach to our Backbone.js form tut from last week, using AngularJS. The resulting code was so much simpler and shorter, that I invited Kevin to share it with you.
The Backbone.js app tutorial from last week shows how to build a dynamic form with JavaScript, jQuery and the Backbone library. It works perfectly well, but there is more than one way to make that form, and here I will show you how to build it with AngularJS. The JS code is only 15 lines, compared to the original 123! And what is more, no additional libraries besides AngularJS are needed!
A few words about AngularJS
AngularJS is a library by Google that aims to clearly separate the HTML rendering from your data. With it, you can declare bindings in your HTML that describe how it should be rendered and to which data model your markup corresponds. If you need to modify any aspect of your page, do it by changing your HTML and CSS; the JS contains your data and the logic behind it - in our example the model are the different services, and the logic is the calculation of the total price.
With AngularJS your code becomes short and easy to understand. This is important as when the code is concise, you can spot where to make changes quickly and is more maintainable.
The HTML
index.html
<!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>Your First AngularJS App</title> <!-- Google web fonts --> <link href="http://fonts.googleapis.com/css?family=PT+Sans:400,700" rel='stylesheet' /> <!-- The main CSS file --> <link href="assets/css/style.css" rel="stylesheet" /> </head> <body> <form id="main" ng-app ng-controller="myservices"> <h1>My Services</h1> <ul id="services"> <!-- The services will be inserted here --> <li ng-repeat="service in services"> <label> <input type="checkbox" ng-model="service.selected" value="1" name="{{service.title}}" /> {{service.title}}<span>${{service.price}}</span> </label> </li> </ul> <p id="total">total: <span>${{total()}}</span></p> <input type="submit" id="order" value="Order"/> </form> <!-- The single AngularJS include --> <script src="http://cdnjs.cloudflare.com/ajax/libs/angular.js/1.0.5/angular.min.js"></script> <script src="assets/js/script.js"></script> </body> </html>
It looks similar to the original with important differences:
- The whole HTML is here. No '<input type="checkbox"...' in the JavaScript;
- New attributes describe what to do with the data. 'ng-repeat' declares the template to use for each service (a <li> with an <input>);
- ng-model="service.selected" associates the checkbox state to a 'selected' variable of each service (initially undefined, meaning false);
- Placeholders indicate where to insert a value from our model. {{service.price}} puts the price;
- Function calls are needed when logic is applied to data. With total() we sum up the price of the selected services;
- The jQuery library is not needed!
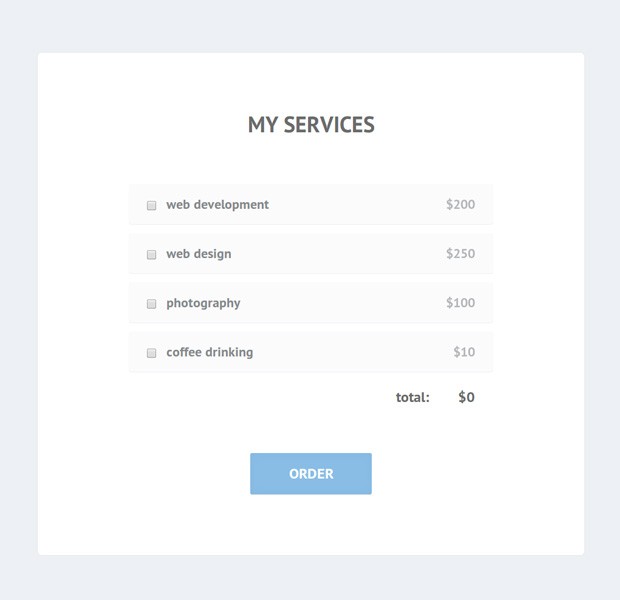
The JavaScript
The JavaScript is as short as it could be:
assets/js/script.js
function myservices($scope){ $scope.services=[ { title: 'web development', price: 200}, { title: 'web design', price: 250}, { title: 'photography', price: 100}, { title: 'coffee drinking', price: 10}]; $scope.total=function(){ var t = 0; angular.forEach($scope.services, function(s){ if(s.selected) t+=s.price; }); return t; }; }
- The function "myservices" matches the ng-controller attribute on our form. AngularJS will act only inside our form;
- Our data model must be stored into the $scope variable to be accessible from the HTML. We first declare our list of services;
- The total() function loops through the services, adding the prices of the selected ones. 'angular.forEach' is a convenient function, a standard 'for' could have been used.
You don't have to worry when your data is read or the total() function called. AngularJS does it for you. Just update the data of the model in JavaScript, and the library will update the HTML.
Go further
AngularJS and Backbone.js are similar in their purpose but solve problems differently. They both give you tools to build highly dynamic web applications. But the usual shortcomings apply - because the application is dependent on JavaScript, these libraries are not good from a SEO point of view.
A good next step is to read the official AngularJS tutorial which explains all the basics, or watch some videos. For a great overview of all the major client-side JS frameworks, you can visit http://todomvc.com/ that implements the same To Do application in each of them.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Excellent! Do you have more tutorials on Angular JS like this. In particular, using it with a backend like Rails or Node/Express?
Thanks.
Bharat
The backend can be any web service.
Angular has the integrated $resource service intended to interact with a RESTful data source.
You can also use jQuery $.ajax() calls if you're more comfortable with them. In this case, the extra step in the AJAX callback is to call
$scope.$apply(function(){ ...your code here... });
so Angular knows that changes happened to the data model.