What You Need To Know About The HTML5 Slider Element
HTML5 brought a lot of new tags and new rules on how the old ones should be used. One of them is the range input element, or the slider. This control has been available in operating systems for decades, but is only now that it has found its way into the browser.
The reason for this delay is probably that it is easy to emulate the functionality with JavaScript alone. The jQuery UI library includes a pretty capable version that is also easy to style. But having it built into the browser and ready to go is much more convenient, at least for the browsers that support it.
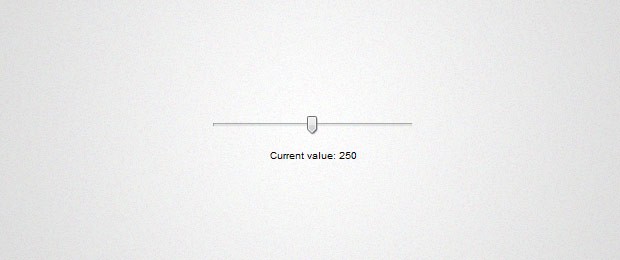
Browser support
All modern browsers support this element with the notable exception of Firefox, but it can be easily recreated with html5slider.js. Of course IE also does not support the range input (no surprise here), for which there are no easy fixes. This means that to use it cross-browser you will still need to include a separate enabling library like jQuery UI (more on that later on). The good thing is that even if the browser does not support the range element, it will fall back to a textbox.
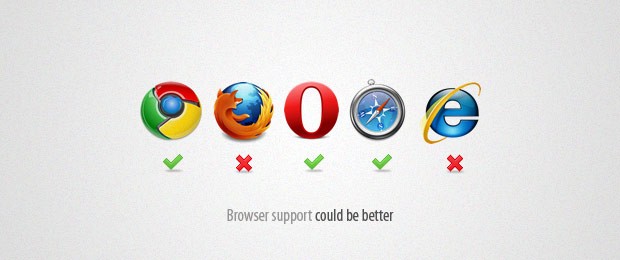
How it works
I referred to the slider as "range input" for a reason. It is a type of input element, rather than a separate tag - <input type="range" />
is all you need to display it in your page. It supports the regular value attribute shared by all input elements, along with min and max, which limit the range, and step which sets how much the value changes on every movement (default is 1).
value | number | not set | The default position of the slider. |
min | number | 0 | The bottom limit of the range. This is the value of the input when the slider is on the leftmost position. |
max | number | 100 | The top limit of the range. This is the value of the input when the slider is on the rightmost position. |
step | number | 1 | The amount with which the value changes on each movement of the slider. |
You can change these attributes with JavaScript/jQuery as you would with any regular input element. You can also use the onchange event to listen for changes. This is what the code looks like:
Extracted from index.html
<input id="defaultSlider" type="range" min="0" max="500" /> <p class="note">Current value: <span id="currentValue">0</span></p>
And this is the jQuery code that listens for the change event:
assets/js/slider-default.js
$(function(){ var currentValue = $('#currentValue'); $('#defaultSlider').change(function(){ currentValue.html(this.value); }); // Trigger the event on load, so // the value field is populated: $('#defaultSlider').change(); });
Of course this code will only work if your browser supports the slider element. Otherwise you will be presented with a textbox.
Lets level the field
As more than two thirds of the internet population won't be able to see our pretty slider, we need to take a different path. Lets build a quick and dirty version of the slider using jQuery UI, a library of interface components that sits on top of jQuery.
Extracted from slider-jqueryui.html
<div id="slider"></div> <p class="note">Current value: <span id="currentValue">0</span></p>
You can see the code for the jQuery UI Slider control below (you need to include jQuery UI alongside jQuery in your page).
assets/js/slider-jqueryui.js
$(function(){ var currentValue = $('#currentValue'); $("#slider").slider({ max: 500, min: 0, slide: function(event, ui) { currentValue.html(ui.value); } }); });
The code is pretty straightforward. It simply uses the slider method and the library does the rest.
The fun part
Here comes a realization - as we are already presenting our own custom version of the slider, we can as well present an entirely different control. Lets use the KnobKnob plugin from last week.
We will have the range input control on the page, but it will be hidden. KnobKnob will then create a rotating control that will have the same limits as the original slider. On every change we will update the value of the hidden slider:
slider-knob.html
<div id="container"> <div id="control"></div> </div> <!-- The range input is hidden and updated on each rotation of the knob --> <input type="range" id="slider" min="0" max="500" value="25" /> <p class="note">Current value: <span id="currentValue">0</span></p>
And for the jQuery part:
assets/js/slider-knob.js
$(function(){ var slider = $('#slider'), min = slider.attr('min'), max = slider.attr('max'), currentValue = $('#currentValue'); // Hiding the slider: slider.hide(); $('#control').knobKnob({ snap : 10, value: 250, turn : function(ratio){ // Changing the value of the hidden slider slider.val(Math.round(ratio*(max-min) + min)); // Updating the current value text currentValue.html(slider.val()); } }); });
The code above takes the min and max attributes of the range input and uses it to calculate the corresponding value of the slider.
Conclusion
The slider control is useful for giving users the ability to conveniently modify a value without having to explicitly type it in a text box. Although there is much to be desired in terms of browser support, you can use progressive enhancement to display an alternative control using jQuery.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Usefull information, thanks.
I prefer to use js plugins, becouse as you said not all browsers support it.
interressantissimo, você é o cara, otima aula,
Up, Tutorialzine :D
Thanks for sharing My friend !! .. i love that working thing!! <3
IE is always a pain in but. I can't believe that Microsoft with all of its power can't make a modern browser. They've spend thousands of hours of creating the concept of Hardware-Accelerated Internet, while IE10 still doesn't support
<input type='range'>
.@Saeed, I completely agree. It feels criminal at times how much money the international business community has been forced to invest to overcome the obvious weaknesses of a piece of software created by a company that has made its billions from that very community. #badBusiness
Ideally you should use an <output> element (see http://wufoo.com/html5/elements/3-output.html) to display (and calculate) the output, with a JS fallback for non HTML5 user agents.
Note taken!
Excellent resource! Thanks for sharing!
Hi, It worked in Firefox here... =)
xxoxoxox
How would I change where 0 is? I would like to make a knob that has the range of an audio knob, with 0 at about 7 o'clock and max at about 5 o'clock.
Nice tutorial. Hard to support new features like this without IE or Firefox though :(
Ahhhhhh like 20 years and we still don't have a dumb stupid slider supported cross-browser :((((
SO SO happy I'm not web developer. such a broken tech!
thank you so much. clear, working, well documented. If only all articles could be just like this one, internet search will be much more enjoyable.