Learn TypeScript in 30 Minutes
Today we're going to take a look at TypeScript, a compile-to-JavaScript language designed for developers who build large and complex apps. It inherits many programming concepts from languages such as C# and Java that add more discipline and order to the otherwise very relaxed and free-typed JavaScript.
This tutorial is aimed at people who are fairly proficient in JavaScript but are still beginners when it comes to TypeScript. We've covered most of the basics and key features while including lots of examples with commented code to help you see the language in action. Let's begin!
The Benefits of Using TypeScript
JavaScript is pretty good as it is and you may wonder Do I really need to learn TypeScript? Technically, you do not need to learn TypeScript to be a good developer, most people do just fine without it. However, working with TypeScript definitely has its benefits:
- Due to the static typing, code written in TypeScript is more predictable, and is generally easier to debug.
- Makes it easier to organize the code base for very large and complicated apps thanks to modules, namespaces and strong OOP support.
- TypeScript has a compilation step to JavaScript that catches all kinds of errors before they reach runtime and break something.
- The upcoming Angular 2 framework is written in TypeScript and it's recommended that developers use the language in their projects as well.
The last point is actually the most important to many people and is the main reason to get them into TypeScript. Angular 2 is one of the hottest frameworks right now and although developers can use regular JavaScript with it, a majority of the tutorials and examples are written in TS. As Angular 2 expands its community, it's natural that more and more people will be picking up TypeScript.
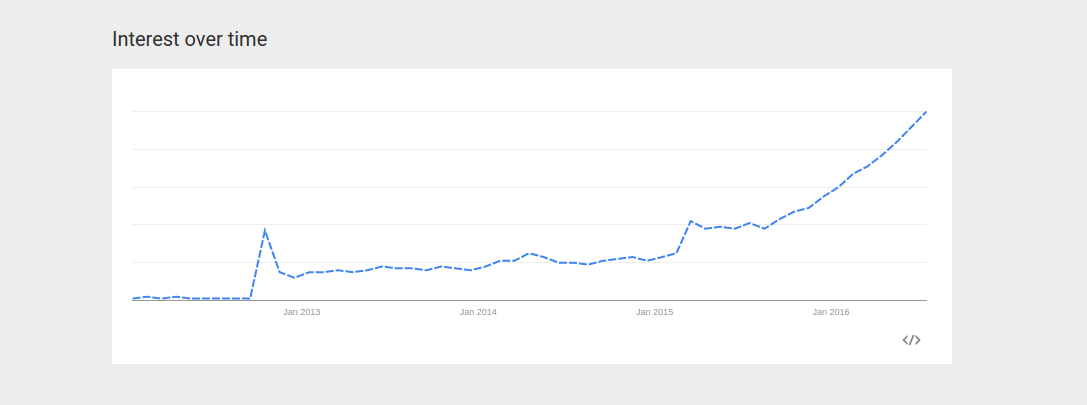
Installing TypeScript
You will need Node.js and Npm for this tutorial. Go here if you don't have them installed.
The easiest way to setup TypeScript is via npm. Using the command below we can install the TypeScript package globally, making the TS compiler available in all of our projects:
npm install -g typescript
Try opening a terminal anywhere and running tsc -v
to see if it has been properly installed.
tsc -v Version 1.8.10
Text Editors With TypeScript Support
TypeScript is an open-source project but is developed and maintained by Microsoft and as such was originally supported only in Microsoft's Visual Studio platform. Nowadays, there are a lot more text editors and IDEs that either natively or through plugins offer support for the TypeScript syntax, auto-complete suggestions, error catching, and even built-in compilers.
- Visual Studio Code - Microsoft's other, lightweight open-source code editor. TypeScript support is built in.
- Official Free Plugin for Sublime Text.
- The latest version of WebStorm comes with built in support.
- More including Vim, Atom, Emacs and others.
Compiling to JavaScript
TypeScript is written in .ts files (or .tsx for JSX), which can't be used directly in the browser and need to be translated to vanilla .js first. This compilation process can be done in a number of different ways:
- In the terminal using the previously mentioned command line tool
tsc
. - Directly in Visual Studio or some of the other IDEs and text editors.
- Using automated task runners such as gulp.
We found the first way to be easiest and most beginner friendly, so that's what we're going to use in our lesson.
The following command takes a TypeScript file named main.ts and translates it into its JavaScript version main.js. If main.js already exists it will be overwritten.
tsc main.ts
We can also compile multiple files at once by listing all of them or by applying wildcards:
# Will result in separate .js files: main.js worker.js. tsc main.ts worker.ts # Compiles all .ts files in the current folder. Does NOT work recursively. tsc *.ts
We can also use the --watch
option to automatically compile a TypeScript file when changes are made:
# Initializes a watcher process that will keep main.js up to date. tsc main.ts --watch
More advanced TypeScript users can also create a tsconfig.json file, consisting of various build settings. A configuration file is very handy when working on large projects with lots of .ts files since it somewhat automates the process. You can read more about tsconfig.json in the TypeScript docs here
Static Typing
A very distinctive feature of TypeScript is the support of static typing. This means that you can declare the types of variables, and the compiler will make sure that they aren't assigned the wrong types of values. If type declarations are omitted, they will be inferred automatically from your code.
Here is an example. Any variable, function argument or return value can have its type defined on initialization:
var burger: string = 'hamburger', // String calories: number = 300, // Numeric tasty: boolean = true; // Boolean // Alternatively, you can omit the type declaration: // var burger = 'hamburger'; // The function expects a string and an integer. // It doesn't return anything so the type of the function itself is void. function speak(food: string, energy: number): void { console.log("Our " + food + " has " + energy + " calories."); } speak(burger, calories);
Because TypeScript is compiled to JavaScript, and the latter has no idea what types are, they are completely removed:
// JavaScript code from the above TS example. var burger = 'hamburger', calories = 300, tasty = true; function speak(food, energy) { console.log("Our " + food + " has " + energy + " calories."); } speak(burger, calories);
However, if we try to do something illegal, on compilation tsc
will warn us that there is an error in our code. For example:
// The given type is boolean, the provided value is a string. var tasty: boolean = "I haven't tried it yet";
main.ts(1,5): error TS2322: Type 'string' is not assignable to type 'boolean'.
It will also warn us if we pass the wrong argument to a function:
function speak(food: string, energy: number): void{ console.log("Our " + food + " has " + energy + " calories."); } // Arguments don't match the function parameters. speak("tripple cheesburger", "a ton of");
main.ts(5,30): error TS2345: Argument of type 'string' is not assignable to parameter of type 'number'.
Here are some of the most commonly used data types:
- Number - All numeric values are represented by the number type, there aren't separate definitions for integers, floats or others.
- String - The text type, just like in vanilla JS strings can be surrounded by 'single quotes' or "double quotes".
- Boolean -
true
orfalse
, using 0 and 1 will cause a compilation error. - Any - A variable with this type can have it's value set to a string, number, or anything else.
- Arrays - Has two possible syntaxes:
my_arr: number[];
ormy_arr: Array<number>
. - Void - Used on function that don't return anything.
To see a list of all of the available types, go to the official TypeScript docs - here.
Interfaces
Interfaces are used to type-check whether an object fits a certain structure. By defining an interface we can name a specific combination of variables, making sure that they will always go together. When translated to JavaScript, interfaces disappear - their only purpose is to help in the development stage.
In the below example we define a simple interface to type-check a function's arguments:
// Here we define our Food interface, its properties, and their types. interface Food { name: string; calories: number; } // We tell our function to expect an object that fulfills the Food interface. // This way we know that the properties we need will always be available. function speak(food: Food): void{ console.log("Our " + food.name + " has " + food.calories + " calories."); } // We define an object that has all of the properties the Food interface expects. // Notice that types will be inferred automatically. var ice_cream = { name: "ice cream", calories: 200 } speak(ice_cream);
The order of the properties does NOT matter. We just need the required properties to be present and to be the right type. If something is missing, has the wrong type, or is named differently, the compiler will warn us.
interface Food { name: string; calories: number; } function speak(food: Food): void{ console.log("Our " + food.name + " has " + food.calories + " grams."); } // We've made a deliberate mistake and name is misspelled as nmae. var ice_cream = { nmae: "ice cream", calories: 200 } speak(ice_cream);
main.ts(16,7): error TS2345: Argument of type '{ nmae: string; calories: number; } is not assignable to parameter of type 'Food'. Property 'name' is missing in type '{ nmae: string; calories: number; }'.
This is a beginners guide so we won't be going into more detail about interfaces. However, there is a lot more to them than what we've mentioned here so we recommend you check out the TypeScript docs - here.
Classes
When building large scale apps, the object oriented style of programming is preferred by many developers, most notably in languages such as Java or C#. TypeScript offers a class system that is very similar to the one in these languages, including inheritance, abstract classes, interface implementations, setters/getters, and more.
It's also fair to mention that since the most recent JavaScript update (ECMAScript 2015), classes are native to vanilla JS and can be used without TypeScript. The two implementation are very similar but have their differences, TypeScript being a bit more strict.
Continuing with the food theme, here is a simple TypeScript class:
class Menu { // Our properties: // By default they are public, but can also be private or protected. items: Array<string>; // The items in the menu, an array of strings. pages: number; // How many pages will the menu be, a number. // A straightforward constructor. constructor(item_list: Array<string>, total_pages: number) { // The this keyword is mandatory. this.items = item_list; this.pages = total_pages; } // Methods list(): void { console.log("Our menu for today:"); for(var i=0; i<this.items.length; i++) { console.log(this.items[i]); } } } // Create a new instance of the Menu class. var sundayMenu = new Menu(["pancakes","waffles","orange juice"], 1); // Call the list method. sundayMenu.list();
Anyone who has written at least a bit of Java or C# should find this syntax comfortably familiar. The same goes for inheritance:
class HappyMeal extends Menu { // Properties are inherited // A new constructor has to be defined. constructor(item_list: Array<string>, total_pages: number) { // In this case we want the exact same constructor as the parent class (Menu), // To automatically copy it we can call super() - a reference to the parent's constructor. super(item_list, total_pages); } // Just like the properties, methods are inherited from the parent. // However, we want to override the list() function so we redefine it. list(): void{ console.log("Our special menu for children:"); for(var i=0; i<this.items.length; i++) { console.log(this.items[i]); } } } // Create a new instance of the HappyMeal class. var menu_for_children = new HappyMeal(["candy","drink","toy"], 1); // This time the log message will begin with the special introduction. menu_for_children.list();
For a more in-depth look at classes in TS you can read the documentation - here.
Generics
Generics are templates that allow the same function to accept arguments of various different types. Creating reusable components using generics is better than using the any
data type, as generics preserve the types of the variables that go in and out of them.
A quick example would be a script that receives an argument and returns an array containing that same argument.
// The <T> after the function name symbolizes that it's a generic function. // When we call the function, every instance of T will be replaced with the actual provided type. // Receives one argument of type T, // Returns an array of type T. function genericFunc<T>(argument: T): T[] { var arrayOfT: T[] = []; // Create empty array of type T. arrayOfT.push(argument); // Push, now arrayOfT = [argument]. return arrayOfT; } var arrayFromString = genericFunc<string>("beep"); console.log(arrayFromString[0]); // "beep" console.log(typeof arrayFromString[0]) // String var arrayFromNumber = genericFunc(42); console.log(arrayFromNumber[0]); // 42 console.log(typeof arrayFromNumber[0]) // number
The first time we called the function we manually set the type to string. This isn't required as the compiler can see what argument has been passed and automatically decide what type suits it best, like in the second call. Although it's not mandatory, providing the type every time is considered good practice as the compiler might fail to guess the right type in more complex scenarios.
The TypeScript docs include a couple of advanced examples including generics classes, combining them with interfaces, and more. You can find them here.
Modules
Another important concept when working on large apps is modularity. Having your code split into many small reusable components helps your project stay organized and understandable, compared to having a single 10000-line file for everything.
TypeScript introduces a syntax for exporting and importing modules, but cannot handle the actual wiring between files. To enable external modules TS relies on third-party libraries: require.js for browser apps and CommonJS for Node.js. Let's take a look at a simple example of TypeScript modules with require.js:
We will have two files. One exports a function, the other imports and calls it.
exporter.ts
var sayHi = function(): void { console.log("Hello!"); } export = sayHi;
importer.ts
import sayHi = require('./exporter'); sayHi();
Now we need to download require.js and include it in a script tag - see how here. The last step is to compile our two .ts files. An extra parameter needs to be added to tell TypeScript that we are building modules for require.js (also referred to as AMD), as opposed to CommonJS ones.
tsc --module amd *.ts
Modules are quite complex and are out of the scope of this tutorial. If you want to continue reading about them head out to the TS docs - here.
Third-party Declaration Files
When using a library that was originally designed for regular JavaScript, we need to apply a declaration file to make that library compatible with TypeScript. A declaration file has the extension .d.ts and contains various information about the library and its API.
TypeScript declaration files are usually written by hand, but there's a high chance that the library you need already has a .d.ts. file created by somebody else. DefinitelyTyped is the biggest public repository, containing files for over a thousand libraries. There is also a popular Node.js module for managing TypeScript definitions called Typings.
If you still need to write a declaration file yourself, this guide will get you started.
Upcoming Features in TypeScript 2.0
TypeScript is still under active development and is evlolving constantly. At the time of the writing of this tutorial the LTS version is 1.8.10, but Microsoft have already released a Beta for TypeScript 2.0. It's available for public testing and you can try it out now:
npm install -g typescript@beta
It introduces some handy new concepts such as:
- Non-nullable types flag which prevents some variables from having their value set to
null
orundefined
. - New improved system for getting declaration files directly with an
npm install
. - Control flow type analysis that catches errors previously missed by the compiler.
- Some innovations in the module export/import syntax.
Another long-awaited feature is the ability to control the flow of asynchronous functions in an async/await
block. This should be available in a future 2.1 update.
Further Reading
The amount of information in the official docs can be a bit overwhelming at first, but the benefits of going through it will be huge. Our tutorial is to be used as an introduction, so we haven't covered all of the chapters from the TypeScript documentation. Here are some of the more useful concepts that we've skipped:
- Namespaces - here.
- Enums - here.
- Advanced Types and Type Guards - here.
- Writing JSX in TypeScript - here.
Conclusion
We hope you enjoyed this tutorial!
Do you have any thoughts on TypeScript and would you consider using it in your projects? Feel free to leave a comment below!
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Comments 27
Awesome tutorial !!!!
Covers everything to get started
No mention of decorator?
Nicely explained. You should submit your tutorial on Hackr.io.
First of all nice article but a frustration is rasied with new syntaxes, why it is not possible to keep the syntax similar to C# if TS is created by Microsoft? Can this request be raised to Microsoft please to keep the syntaxes same?
Really helpful & awesome article ..!
I've just started learning Angular 2 and was having some problems with the typescript syntax. This tutorial just came up as if ordered. The interface section really helped me out a bunch. Thank you.
Really good tutorial, I am new to typescript and this tutorial gave me a broad overview.
Really good article!!! I'm new to typescript. It helped me a lot
The following is a minor error.
In the section on types, in the code (copied and pasted below), a comment says that the function speak expects a string and an integer. But the second parameter, being of type number, does not have to be an integer; it can be any number.
var burger: string = 'hamburger', // String
calories: number = 300, // Numeric
tasty: boolean = true; // Boolean
// Alternatively, you can omit the type declaration:
// var burger = 'hamburger';
// The function expects a string and an integer.
// It doesn't return anything so the type of the function itself is void.
function speak(food: string, energy: number): void {
console.log("Our " + food + " has " + energy + " calories.");
}
speak(burger, calories);
Thank you so much for this post for TypeScript. I'm the beginner of AngularJS 2 :D
Thank you.I am new to typescript, and really its a very good overview for me. Thanks a lot.
It helped a lot, really gave me confidence to go further. Thanks a ton Author.
Great starting tutorial - I've read some books and tutorials on TypeScript - none of 'em have this approach. So - keep up a good work !
Awesome tutorial, good overview.
Well written and the combination of breakdowns with small code snippets make this tutorial better than the others I've been reading.
Thanks, simple, general and useful.
This is very good article for beginners. It gave me a good idea of what typescript is about.
Thank you for author.
Thank you for this tutorial :)
Very very informative as a beginner to TypeScript!
Nice Tutorial. After going through this I felt that people coming from Java background would be more at home using Typescript than vanilla Javascript. Thanks..
I just started exploring Angular 2, but was unaware of typescript. article helped a lot knowing the syntax's.
Best Tutorial! Well Explained. Thanks! :)
Probably the best article about TypeScript, thank you - helped me a lot.
Thanks for the wonderful jumpstart. Much appreciated. God bless you richly
Awesome Tutorial!! quick and crisp
Great tutorial, easy explanation and simple examples :)!
I absolutely hated pure Javascript. I was disgusted by it. TypeScript made the front end programming fun for me.
Thank you to the author of this article .... I've heard many years ago the name "TypeScript" being mentioned at Microsoft Events. Now when I have to work on Node.js, websocket and SPA ... I realized that it is very much C# language-like !
Well done for a very well article write up.