Making an Apple-style Splash Screen
The Beatles are on iTunes! Or, if you are like me and don't care that much about it, you've probably noticed the awesome splash screen that Apple used to promote the event. Risking to start a trend, in this tutorial we are going to create a simple jQuery plugin that will display fancy Apple-style splash screens for us.
The HTML
Encapsulating the splash screen functionality into a jQuery plugin makes it portable and easy to incorporate into an existing website. But before starting work on the plugin files, we first need to lay down the HTML markup of a simple demonstration page, where the plugin is going to be used.
index.html
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Apple-style Splash Screen | Tutorialzine Demo</title> <link rel="stylesheet" type="text/css" href="css/styles.css" /> <link rel="stylesheet" type="text/css" href="css/splashscreen.css" /> <script src="http://ajax.microsoft.com/ajax/jQuery/jquery-1.4.4.min.js"></script> <script src="js/jquery.splashscreen.js"></script> <script src="js/script.js"></script> </head> <body> <div id="page"> <div id="topBar"></div> <div id="promoIMG"> <img src="img/available_now.png" alt="Available Now" /> </div> <div id="contentArea"> <p>... The textual content ...</p> </div> </div> </body> </html>
Notice that I've placed the script tags in the head section. Usually it is best to include them in the body, after all the other content, as otherwise the page rendering blocks while the js files are being downloaded and our visitor is left staring at a blank screen. This time, however, this is a necessary evil, as we need to be sure that nothing of the page is going to be shown to the user before we show the splash screen.
The code of the splash screen plugin is contained in jquery.splashscreen.js, which we will be discussing in the last section. The plugin depends on splashcreen.css, which defines some useful styles. You can see the stylesheet in the next section of the tutorial.
The #promoIMG div has the MacBook illustration set as its background. This is what our plugin is going to be looking for, when we call it. It will then create a splash screen div and set the illustration as its background, offsetting it so it aligns with the #promoIMG div below it. Finally, when the splash screen is hidden, we are left with the impression that the page around it slowly fades in.
The CSS
We have two distinct stylesheets - styles.css and splashscreen.css. The first one is pretty straightforward, and as it is only used to style the underlying page, is of no interest to us and will not be discussed here. The styles that define the looks of the splash screen are separated in their own file - splashcreen.css, which you can see below.
splashscreen.css
#splashScreen img{ margin:0 auto; } #splashScreen{ position:absolute; top:0; left:0; height:100%; width:100%; background-color:#252525; background-repeat:no-repeat; text-align:center; }
In the fragment above, we are basically saying that the stylesheet div is positioned absolutely with relation to the document window and that it should take the full width and height of the page. The background color is the same as the one of the MacBook illustration, which we are using as a background image, as you will see in a moment.
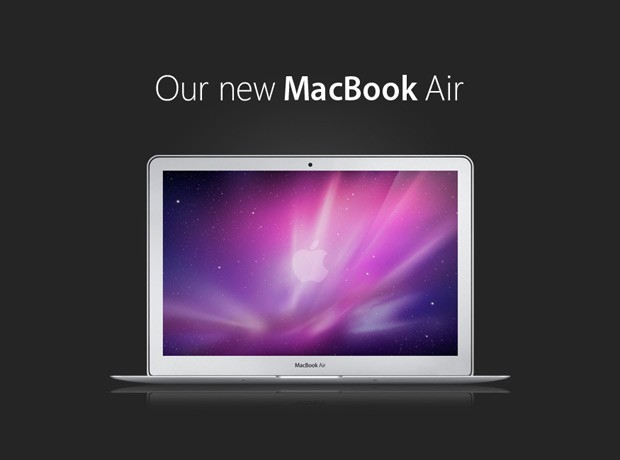
The jQuery
All of the plugin code resides in jquery.splashscreen.js. As I mentioned in the first section of the tutorial, it is important that the script is loaded before any content is shown to the user, otherwise they will witness a somewhat unpleasant flicker when the splashscreen is shown.
jquery.splashscreen.js
// A self-executing anonymous function, // standard technique for developing jQuery plugins. (function($){ $.fn.splashScreen = function(settings){ // Providing default options: settings = $.extend({ textLayers : [], textShowTime : 1500, textTopOffset : 80 },settings); var promoIMG = this; // Creating the splashScreen div. // The rest of the styling is in splashscreen.css var splashScreen = $('<div>',{ id : 'splashScreen', css:{ backgroundImage : promoIMG.css('backgroundImage'), backgroundPosition : 'center '+promoIMG.offset().top+'px', height : $(document).height() } }); $('body').append(splashScreen); splashScreen.click(function(){ splashScreen.fadeOut('slow'); }); // Binding a custom event for changing the current visible text according // to the contents of the textLayers array (passed as a parameter) splashScreen.bind('changeText',function(e,newID){ // If the image that we want to show is // within the boundaries of the array: if(settings.textLayers[newID]){ showText(newID); } else { splashScreen.click(); } }); splashScreen.trigger('changeText',0); // Extracting the functionality into a // separate function for convenience. function showText(id){ var text = $('<img>',{ src:settings.textLayers[id], css: { marginTop : promoIMG.offset().top+settings.textTopOffset } }).hide(); text.load(function(){ text.fadeIn('slow').delay(settings.textShowTime).fadeOut('slow',function(){ text.remove(); splashScreen.trigger('changeText',[id+1]); }); }); splashScreen.append(text); } return this; } })(jQuery);
The plugin is passed a settings object. The only property of this object that is required is textLayer. This property should be an array with the URLs of all the images with promo phrases that fade in and out above the MacBook illustration. You can make this array arbitrary long, and even put any kind of images there, not just containing text. You could even turn it into a stylish slideshow.
The this of the function corresponds to the promoIMG div. Notice how we take the offset of the image from the top of the page with the offset() method on line 25, and set it as the offset of the background image on the splash screen. This gives us perfect alignment and in the same time frees us from having to pass the offset as a parameter, making the plugin more robust.
You can see how the plugin is used in the fragment below:
script.js
$(document).ready(function(){ // Calling our splashScreen plugin and // passing an array with images to be shown $('#promoIMG').splashScreen({ textLayers : [ 'img/thinner.png', 'img/more_elegant.png', 'img/our_new.png' ] }); });
What is left is to only call the splash screen plugin. The document ready event is perfect for the task, as it is called before the page is visible to the user and we can safely display the screen straight away.
With this our Apple-like Splash Screen Plugin is complete!
Parting words
You can use the plugin to easily create your own stylish intro splash screens. You only need to remember to limit it only to one of your pages, as it will become annoying to the user if they have to sit through a 30 second intro on every page view. Also, using the textLayers parameter, you can turn it into a slide show with different product screenshots, which would be an interesting effect to see.
Be sure to share your ideas for this script in the comment section below.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Me too Martin,
Love that splash screen when I saw the Beatles page in Apple...
Awesome in jQuery.
Thanks
Perfect timing! I was just searching for a tutorial on this.
Just what I was looking for. Thanks.
Very good script
Nice Job!
Again.
awesome script and I am gonna try it with some different contents
Fantastic tutorial!
Love the effect! Great tutorial!
wow YOU DID IT AGAIN and I have to say this and I mean it, You are ahead of any other sites were quality tutorials are posted and by miles...
: )
Thank you.
I just saw the splash on apple and thought this is great, but this is greater :)
Great work! But can you humour a newbie: How can I implement this on a Wordpress installation?
For some reason it doesn't work in Chrome for me.
wa,it's good!
Super Cool Stuffs, Thanks a lot!
Very nice! Thank you.
Pretty awesome!
Perfect timing, was just looking for the 'Beatles' splash page script for some web-shops. Thanks!
Fantastic keep up the good work!
Cool stuff, but you should be use
"splashScreen.bind('splashScreen.changeText',...)"
instead of
"splashScreen.bind('changeText',...")
in order to avoid collision w/ user defined events.
Okay, it's been official for a while but this seals the deal, I'm a tutorialzine groupie!
Great stuff. I've been working on something similar but I went a completely different route and used jquerytools expose plugin to achieve the layover effect. It was handy because it allowed easily for a background image to be used when overlaying the site instead of a solid color, but I'm sure you could work that in on this example as well.
It might be good to add to the code some jQuery cookie (sessional would be best) action to allow for showing the animation only once per session so as to not annoy users on smaller sites where the homepage might be reloaded more often (blogs with featured posts come to mind.
Great work! Thanks for putting this out there for the community.
This is very cool! But how can I ensure that this screen only 1 time per visitor is displayed?
Thanks :)
A quick temporary fix would be to create a duplicate index page that doesn't contain the intro, "index2" that all other pages link to, rather than the actual index.
Simple great...!!!
Great... but why jQuery from Microsoft... just curious :)
hihihi :D
cool cool \m/
Very Nice.... :)
I love this technique, but my concern would be accessibility and usability issues. I like how you can click the intro screen to make it jump to the content, would this be enough for users though?
I would love to hear others thoughts on this. Thanks!
Very cool! :)
But how can I ensure that this screen only 1 time per visitor is displayed?
You set a Cookie an check for it on every page load. If set or equals to a certain value, like 1, you display the splash screen, else, you don't.
nice. I like apple
Excellent Tutorial, I like this effect, Apple always has good ideas.
I think maybe setting up a cookie for checking if the user saw it once per browser session?
Referring to another tutorial here, http://tutorialzine.com/2010/03/microtut-getting-and-setting-cookies-with-jquery-php/. Shouldn't be that hard.
wonderful its really cool and easy to do thanks
Great script! Just wondering if it's possible to redirect to a specified URL upon load after the animation has completed?
Hello there :)
Just wondering: there's a way of using a swf for the splash screen instead of a background image?
There shouldn't be a problem with using an SWF. You just have to edit jquery.splashscreen.js and instead of adding a background image at line 24, add the HTML code for embedding the swf.
var splashScreen = $('',{
id : 'splashScreen',
css:{
paddingTop :promoIMG.offset().top,
height : $(document).height()
}, html:''
});
Is it possible to have the splash screen just fade out and reveal the normal page bellow?
And has anyone sorted out having it only play once per visitor?
Thanks
Anyone have issues with this in IE? I am using a slightly modified version of this to cycle through 4 small gif's, and was having issues with the text.load() not being called when the page is refreshed (F5). It seems to be an issue with IE caching the images, and I was able to solve it using the advice here: http://css-tricks.com/snippets/jquery/fixing-load-in-ie-for-cached-images/
Funny part it, I haven't experienced the issue with the demo here, so wondering why it is...anyone else having a similar issue, or maybe my code isn't up to par?
Actually, scratch my last comment about not having the problem with the demo code here - I was able to replicate it on IE7. Problem is, once the images have been cached by IE (as with a page refresh), it skips over the .load() code. Only workaround seems to be to attach a random query to the img src, which forces IE not to cache.
Trying to figure out the cookie thing. It is a great effect if it only plays once. If you have to run through the splash screen every time i can become annoying.
Anyone else had any luck with this?
No luck here. Would love it to play once only.
This is awesome! thanks for posting!
Anyone wondering how to make sure it only plays once per session use the jQuery cookie plugin and just go...
// if cookie is not there, show splash
if ($.cookie('splash') != 'shown'){
//set splash cookie for one session
$.cookie('splash', 'shown');
// .spashscreen code here...
} else{
// do nothing
}
God good thank you.. I've been trying to work with cookies and a plugin for splash-screens in wordpress and it was a mess!! Tweaked this along with the jquery cookie plugin and now it works like a frickin charm! :D
Good Work I'm an newbie in jquery, and this is all I was looking for!!!!!!!
Greetz from Colombia!!!
How do you change the position of the text layers? I want to position them in the lower right section but can't find a css rule that seems to have an effect on them and I'm not that familiar with jQuery.
Hello,
Awesome job on the splash-screen.
Can you advise how I can use this plug-in with an existing Wordpress theme?
Can you provide instructions on how I can load splash-screen css and js in the header section without interfering with other theme or plugin script? Thanks.
hi there,
i think you can use js cookies,let this effect appear once,not everytime.otherwise when user refresh their browser,screen will repeat this splash effect.
Hi Martin
Great plugin. I was wondering if there is a way to put two images on the splash plugin and only having a colored background. Like remove the mac-book image and leave the changing text and below it have a picture of logo or something.
Thanks in advance.
Hi Martin
I am having a problem with the plugin. I modified your code so the text and image of the macbook are in separate div elements when the text changes the image of the macbook flickers or something like it i don't know if i am using the right word. So if you could take a look at the code an tell me what might be wrong.
Modified code from jquery.splashscreen.js
// A self-executing anonymous function,
// standard technique for developing jQuery plugins.
(function($){
})(jQuery);
and
Modified code from splashscreen.css
splashScreen img{
}
nam{
}
text{
}
splashScreen{
}
Heya I'd like to do 2 simple things please. (not so simple for me, I'm a beginner.
I'd like to make the splash page static so that the user has to click an 'Enter Here' button.
Your help would be much appreciated, thanks a million for our work so far....cheers!
WOW! I can't believe how amazing this is! You are so cool to be able to create things like this! I hope I can brush up on my skills to create an apple style anything! Ha! Thanks for the tut!
How can I get this to work with a cookie? I only want it to show up on the first time a visitor enters my site...
LOVE this! I want to modify the code so that the splash screen goes away, ONLY if I click somewhere. Please advice!!
Thanks for sharing! Great work but how do you redirect to a specified URL upon load after the animation has completed?