Google Powered Site Search with jQuery
By far one of the most requested features by Tutorialzine's readers, is building a site-wide search. One way to do it, is to build it yourself from the ground up. That is, to use a server-side language like PHP and run search queries on your database, displaying the results to the user.
Another way is to use the services of the one search engine that already knows everything about everyone. Yep, you guessed it. In this tutorial we are using Google's AJAX Search API, to create a custom search engine, with which you can search for web results, images, video and news items on your site.
Important update (October 2014): Google has canceled the API that this search engine uses, so this script will no longer work. You can use their custom search engine to build a search function for your website.
The HTML
Lets start with the HTML markup. From the new HTML5 doctype, we move on to defining the title of the document and including the stylesheet to the head section of the page.
search.html
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Google Powered Site Search | Tutorialzine Demo</title> <link rel="stylesheet" type="text/css" href="styles.css" /> </head> <body> <div id="page"> <h1>Google Powered Site Search</h1> <form id="searchForm" method="post"> <fieldset> <input id="s" type="text" /> <input type="submit" value="Submit" id="submitButton" /> <div id="searchInContainer"> <input type="radio" name="check" value="site" id="searchSite" checked /> <label for="searchSite" id="siteNameLabel">Search</label> <input type="radio" name="check" value="web" id="searchWeb" /> <label for="searchWeb">Search The Web</label> </div> <ul class="icons"> <li class="web" title="Web Search" data-searchType="web">Web</li> <li class="images" title="Image Search" data-searchType="images">Images</li> <li class="news" title="News Search" data-searchType="news">News</li> <li class="videos" title="Video Search" data-searchType="video">Videos</li> </ul> </fieldset> </form> <div id="resultsDiv"></div> </div> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script> <script src="script.js"></script> </body> </html>
In the body section, we have the main container element - the #page div. The form inside it, acts not only as a search form, but as a container as well. It has CSS3 rounded corners and a darker background color applied to it, which makes it more easily distinguishable from the rest of the page.
Inside the form is the text input box, after which comes the radio group for searching on the current site / the web, and the four search type icons, organized as an unordered list. Lastly we include jQuery and our scripts.js, which is discussed in the last step of this tutorial.
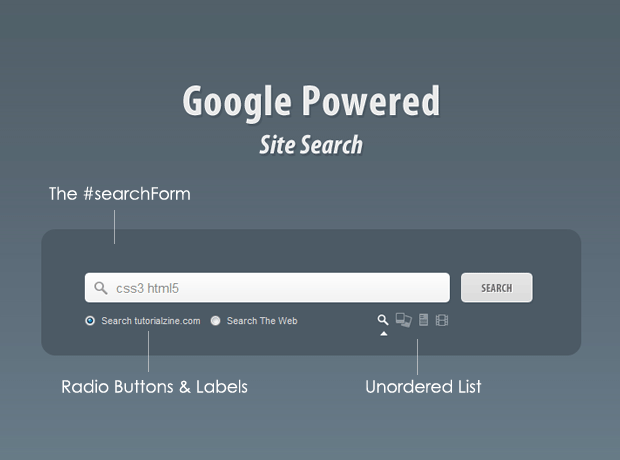
The CSS
The CSS styles reside in styles.css. Only the more interesting parts are included here.
styles.css - Part 1
#searchForm{ /* The search form. */ background-color:#4C5A65; padding:50px 50px 30px; margin:80px 0; position:relative; -moz-border-radius:16px; -webkit-border-radius:16px; border-radius:16px; } fieldset{ border:none; } #s{ /* The search text box. */ border:none; color:#888888; background:url("img/searchBox.png") no-repeat; float:left; font-family:Arial,Helvetica,sans-serif; font-size:15px; height:36px; line-height:36px; margin-right:12px; outline:medium none; padding:0 0 0 35px; text-shadow:1px 1px 0 white; width:385px; }
As mentioned above, the form's functions are not limited to only submitting data, but also to act as a regular container element. This keeps the markup on the page to a minimum, while still providing rich functionality.
The text input box, #s, is styled with a background image and padding, so that the text does not cover the magnifying glass.
styles.css - Part 2
.icons{ list-style:none; margin:10px 0 0 335px; height:19px; position:relative; } .icons li{ background:url("img/icons.png") no-repeat; float:left; height:19px; text-indent:-999px; cursor:pointer; margin-right:5px; } /* Styling each icon */ li.web{ width:15px;} li.web.active, li.web:hover{ background-position:left bottom;} li.images{ width:22px; background-position:-18px 0;} li.images.active, li.images:hover{ background-position:-18px bottom;} li.news{ width:14px; background-position:-44px 0;} li.news.active, li.news:hover{ background-position:-44px bottom;} li.videos{ width:17px; background-position:right 0;} li.videos.active, li.videos:hover{ background-position:right bottom;} span.arrow{ /* The little arrow that moves below the icons */ width:11px; height:6px; margin:21px 0 0 5px; position:absolute; background:url('img/arrow.png') no-repeat; left:0; } /* The submit button */ #submitButton{ background:url('img/buttons.png') no-repeat; width:83px; height:36px; text-indent:-9999px; overflow:hidden; text-transform:uppercase; border:none; cursor:pointer; } #submitButton:hover{ background-position:left bottom; }
In the fragment above, you can see that the search type icons all share a single background image. It is offset with background position so the appropriate part of it is shown, for both the default and the hover state.
The same technique is used for the submit button. Its text is hidden with a negative text-indent, and buttons.png is shown as its background, with the top part of the image visible by default and the bottom on hover.
styles.css - Part 3
/* Web & news results */ .webResult{ text-shadow:1px 1px 0 #586a75;margin-bottom:50px;} .webResult h2{ background-color:#5D6F7B; font-size:18px; font-weight:normal; padding:8px 20px; /* Applying CSS3 rounded corners */ -moz-border-radius:18px; -webkit-border-radius:18px; border-radius:18px; } .webResult h2 b{ color:#fff; } .webResult h2 a{ color:#eee;border:none;} .webResult p{ line-height:1.5;padding:15px 20px;} .webResult p b{ color:white;} .webResult > a{ margin-left:20px;} /* Image & video search results */ .imageResult{ float:left; height:170px; margin:0 0 20px 40px; text-align:center; width:150px; } .imageResult img{ display:block;border:none;} .imageResult a.pic{ border:1px solid #fff; outline:1px solid #777; display:block; margin:0 auto 15px; } /* The show more button */ #more{ width:83px; height:24px; background:url('img/more.png') no-repeat; cursor:pointer; margin:40px auto; } #more:hover{ background-position:left bottom; }
In the last fragment, we style the results. Although we show four types of search results - web, news, images and video, these are only styled by the two classes above - .webResult and .imageResult. Lastly, we style the #more button, which is dynamically added to the page by jQuery depending on the results returned by Google.
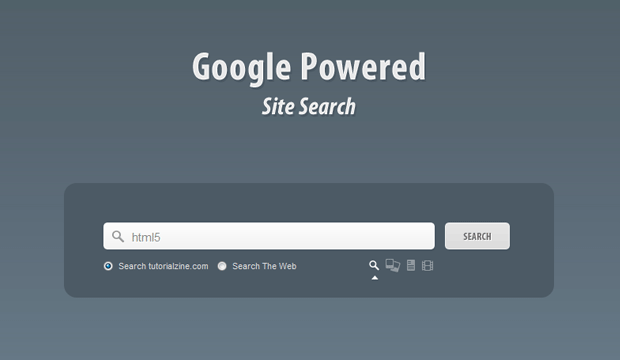
The jQuery
As mentioned in the beginning, this app uses Google's AJAX Search API. Google do provide their own JavaScript library, but if you choose to use it, you are constrained with their UI. While functional, it may not be what you want to offer your visitors. This is why, in this tutorial, we are using the "naked version" by issuing JSONp calls with jQuery directly to their API.
Before we start discussing the jQuery code, lets have a glimpse at what data Google makes available to us, after we run a search with the API.
Sample Result From Google's API
{ "GsearchResultClass": "GwebSearch", "unescapedUrl": "https://tutorialzine.com/2010/02/html5-css3-website-template/", "url": "https://tutorialzine.com/2010/02/html5-css3-website-template/", "visibleUrl": "tutorialzine.com", "cacheUrl": "http://www.google.com/search?q=cache:_NSLxH-cQMAJ:tutorialzine.com", "title": "Coding a <b>CSS3</b> & <b>HTML5</b> One-Page Website Template | Tutorialzine", "titleNoFormatting": "Coding a CSS3 & HTML5 One-Page Website Template | Tutorialzine", "content": "Feb 16, 2010 <b>...</b> Here we are using the new version of HTML.." }
A search run through their API would return the same set of result that you'd normally get directly from their site. The difference is that here we get a JavaScript array populated with objects like the one above. Each of these objects holds the type of search, a title, a URL, and text from the page that contains the terms we are searching for.
Using the GsearchResultClass property, we can determine how to display the information, as you will see in a moment. This search app supports only web, image, news and video searches but you can see a complete list of the available types of searches in Google's AJAX search documentation.
script.js - Part 1
$(document).ready(function(){ var config = { siteURL : 'tutorialzine.com', // Change this to your site searchSite : true, type : 'web', append : false, perPage : 8, // A maximum of 8 is allowed by Google page : 0 // The start page } // The small arrow that marks the active search icon: var arrow = $('<span>',{className:'arrow'}).appendTo('ul.icons'); $('ul.icons li').click(function(){ var el = $(this); if(el.hasClass('active')){ // The icon is already active, exit return false; } el.siblings().removeClass('active'); el.addClass('active'); // Move the arrow below this icon arrow.stop().animate({ left : el.position().left, marginLeft : (el.width()/2)-4 }); // Set the search type config.type = el.attr('data-searchType'); $('#more').fadeOut(); }); // Adding the site domain as a label for the first radio button: $('#siteNameLabel').append(' '+config.siteURL); // Marking the Search tutorialzine.com radio as active: $('#searchSite').click(); // Marking the web search icon as active: $('li.web').click(); // Focusing the input text box: $('#s').focus(); $('#searchForm').submit(function(){ googleSearch(); return false; }); $('#searchSite,#searchWeb').change(function(){ // Listening for a click on one of the radio buttons. // config.searchSite is either true or false. config.searchSite = this.id == 'searchSite'; });
The config object holds general configuration options, such as the site URL, a start page (used in the pagination), and the default type of search (a web search). Google only allows us to select 8 results at a time, which is enough for web searches, but not so for images. Lets hope that Google will raise this limit in the future.
When the form is submitted, jQuery calls our googleSearch() function, which you can see below.
To integrate the search into your website, just replace the siteURL property of the config object, with that of your own site URL.
script.js - Part 2
function googleSearch(settings){ // If no parameters are supplied to the function, // it takes its defaults from the config object above: settings = $.extend({},config,settings); settings.term = settings.term || $('#s').val(); if(settings.searchSite){ // Using the Google site:example.com to limit the search to a // specific domain: settings.term = 'site:'+settings.siteURL+' '+settings.term; } // URL of Google's AJAX search API var apiURL = 'http://ajax.googleapis.com/ajax/services/search/'+settings.type+ '?v=1.0&callback=?'; var resultsDiv = $('#resultsDiv'); $.getJSON(apiURL,{ q : settings.term, rsz : settings.perPage, start : settings.page*settings.perPage },function(r){ var results = r.responseData.results; $('#more').remove(); if(results.length){ // If results were returned, add them to a pageContainer div, // after which append them to the #resultsDiv: var pageContainer = $('<div>',{className:'pageContainer'}); for(var i=0;i<results.length;i++){ // Creating a new result object and firing its toString method: pageContainer.append(new result(results[i]) + ''); } if(!settings.append){ // This is executed when running a new search, // instead of clicking on the More button: resultsDiv.empty(); } pageContainer.append('<div class="clear"></div>') .hide().appendTo(resultsDiv) .fadeIn('slow'); var cursor = r.responseData.cursor; // Checking if there are more pages with results, // and deciding whether to show the More button: if( +cursor.estimatedResultCount > (settings.page+1)*settings.perPage){ $('<div>',{id:'more'}).appendTo(resultsDiv).click(function(){ googleSearch({append:true,page:settings.page+1}); $(this).fadeOut(); }); } } else { // No results were found for this search. resultsDiv.empty(); $('<p>',{ className : 'notFound', html : 'No Results Were Found!' }).hide().appendTo(resultsDiv).fadeIn(); } }); }
The googleSearch() function sends a JSONp request to Google's API, generates the markup of the results, and inserts it into the #resultsDiv div. It can either empty that div beforehand (if we are making a fresh search) or append the results (this happens when we click the "More" button).
Both paths follow the same logic - a new .pageContainer div is created for each set of results (this div has a bottom border, so it is easier to distinguish one page of results from the next) and an object of the result class (you can see this class below), is initialized and its markup is appended to the pageContainer.
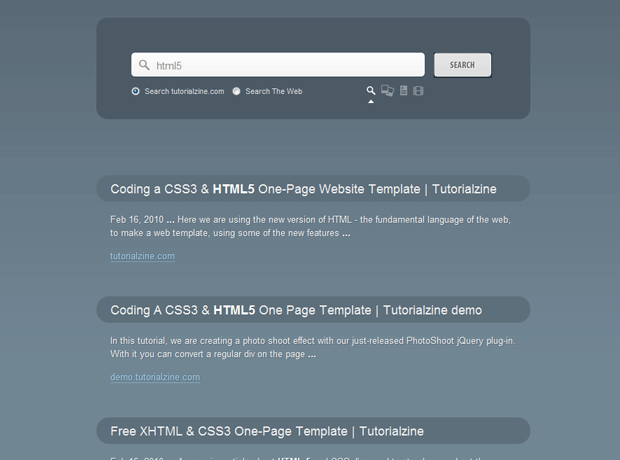
script.js - Part 3
function result(r){ // This is class definition. Object of this class are created for // each result. The markup is generated by the .toString() method. var arr = []; // GsearchResultClass is passed by the google API switch(r.GsearchResultClass){ case 'GwebSearch': arr = [ '<div class="webResult">', '<h2><a href="',r.url,'">',r.title,'</a></h2>', '<p>',r.content,'</p>', '<a href="',r.url,'">',r.visibleUrl,'</a>', '</div>' ]; break; case 'GimageSearch': arr = [ '<div class="imageResult">', '<a href="',r.url,'" title="',r.titleNoFormatting, '" class="pic" style="width:',r.tbWidth,'px;height:',r.tbHeight,'px;">', '<img src="',r.tbUrl,'" width="',r.tbWidth,'" height="', r.tbHeight,'" /></a>','<div class="clear"></div>', '<a href="',r.originalContextUrl,'">',r.visibleUrl,'</a>', '</div>' ]; break; case 'GvideoSearch': arr = [ '<div class="imageResult">', '<a href="',r.url,'" title="',r.titleNoFormatting,' " class="pic" style="width:150px;height:auto;">', '<img src="',r.tbUrl,'" width="100%" /></a>', '<div class="clear"></div>','<a href="', r.originalContextUrl,'">',r.publisher,'</a>', '</div>' ]; break; case 'GnewsSearch': arr = [ '<div class="webResult">', '<h2><a href="',r.unescapedUrl,'">',r.title,'</a></h2>', '<p>',r.content,'</p>', '<a href="',r.unescapedUrl,'">',r.publisher,'</a>', '</div>' ]; break; } // The toString method. this.toString = function(){ return arr.join(''); } } });
This function acts as the constructor of the result class. It takes the object which was returned from Google's API (which you saw at the beginning of the jQuery step) and initializes arr according to the value of GsearchResultClass. Notice that arr is assigned an array instead of a string. This is a bit faster than multiple concatenations of a string together.
At the bottom of the class, we have the toString() method. It basically calls the array's internal join method, turning it into a string. toString() is a magical method, which is implicitly called on line 38 of script.js - Part 2.
With this our own Google Powered Search Engine is complete!
Conclusion
Configuring this app to search your site is really simple. Just change the siteURL property of the config object in script.js. There are many ways how you can improve this example. Not all the data that comes from Google is currently displayed. You could also use the filetype: search modifier to look for specific types of files.
Share all the interesting ideas that you come up with in the comment section.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Awesome stuff! :D
Woah, very nice tutorial and outcome! Thanks for sharing.
Thank you guys! Glad you like it :)
Awesome tutorial!
as always it looks great! good job, martin!
good style, good functionality :)
Wow! Exactly what I needed. Great job and thanks for sharing.
Following tzine from Mexico!
Siempre intente hacer algo similar y no lo habia conseguido.
¡Muy bueno! +10!
Are you really allowed to display results from Google without informing the user that the search result is from Google? I have looked at Google search for my site a few times but all I have found has required me to display the Google logo.
Maybe you are right. It didn't occur to me that they had additional requirements for their API usage (it isn't mentioned in the developer docs either).
Currently, the design features a huge "Google Powered" heading, so I think that the example is compliant to their terms (I am pretty sure they don't need a backlink).
But if you plan to remove the heading and put something like "Search example.com" you'd probably have to put a small google logo somewhere.
Это великолепно (It's Great) You tutorial's are best of the best :)
u have no idea how i wait for new tuts.. thanks
Hello, excellent work :)
I woke up this morning thinking I need to make a search for my site; so this was perfect timing :) Thankyou, Tutorialzine; I love your tutorials :)
Thank you, glad that you find the tutorial useful :)
Anybody knows how to do a search in two or more sites using this great tutorial?
Thank you!
Great! thanks alot =D I can't wait for the new tuts D:
Is it possible to customize this code so that rather than simply searching my site, the API searches a select group of other URL's?
This search supports anything that you'd type in the search box on Google's home page. For example, in the demo I used the site: modifier to limit the search to the current site only.
However, I couldn't find a way to limit the search to a group of domains. I don't think it is supported by Google in general. I also tried to use their OR modifier and include more than one site: clause, but at no avail.
You could always use Google CSE for that... http://www.google.com/cse/
WOW!!! A Great Tutorial thanks
Very very nice tut! thanks you so much ^^!
Impressive turtorial as always, thanks for sharing!
How can I intergrate my google CSE?
SUPERB!
Very nice, not only is the code great but the design is very clean.
Looking forward to your next post
Awesome tut!
Thank You for Sharing! Can it also get the actual dimension of the images on Image Search? - WebDev from Philippines
coooooolll... I needed it for client..
This is my output:
%3Fpage%3Dnews%26id%3D97
instead of
?page=news&id=97
Anyone can help me???
I set the example to use the unescapedUrl property of the google response, instead of just url. It should work now. Try to re-download it. Only script.js is changed.
Awesome Tutorial..!!
Super cool script. Thanks so much for sharing.
very very nice :)
just that we should make it to output 9 images and videos instead of 8
and thanks for yet another beautiful tutorial
Unfortunately it is not possible to output 9 images. 8 is the maximum. I personally would like to be able to display several times that amount, but it is still better than nothing.
Isn't it possible to do multiple request for more images?
Ok great it's working now tnx
great tutorial...nice work
Wow! Congratulations with the visitor boost, Martin. :-D Maybe you could write up an article again like you did a while ago? Where your visitors are coming from etc.
Awesome tutorial!
just wondering, which iconset did these icons come from?
The icons are from http://www.iconsweets.com/
Damn, it's great!
I would love to incorporate this in a site i'm developing for a client, but i was wondering if there was a way to make it so that the search results weren't displayed on a the same page, but instead either a new window, or the page redirects to a page designed to the display the results. I want to place the search box in the footer and having the results display there wouldn't work well.
You can place a generic search form (only a input box and a button) in the footer, and make the demo available on the site, at say http://www.example.com/search.php
When the form in the footer is submitted, it'll to send the contents of the search box to http://www.example.com/search.php (preferably via POST).
You should rename search.html from this example to search.php and change this code:
To this
This will automatically populate the text field with the terms that were entered in the footer form. Now all that is left is to add this line to the bottom of script.js (inside document.ready):
The whole idea is to populate the form and fire a search when the page loads.
Hi Martin, great code man...
I'm looking to do the same as J Roberts, where the search list comes up on a separate page. I was trying to follow the directions you gave, but a bit lost. Can you break it down a little more? I have the text field and submit bttn on my main page, and I switched the search.html to search.php. I also made the mods to the .css and .js files.
I'm just not sure how to make the main page communicate with the search.php page. How do I tell it - when you click search bttn go to search.php and display content.
A million thanks in advance.
-S
Hi Martin, I have tried the suggestion you gave above, but when the I hit submit and the main re-directs, it does NOT fill the text fill automatically. The .js search happens automatically, no problem, but the input text field in the new page does not automatically fill with the word/s typed on the previous page.
Can you please let me know what i may be overlooking.
A million thanks,
Serge
Serge,
I am trying to do the same. Have the search form to submit the results to another page. Did you have any luck?
Very nice tutorial and design for the demo. I really adore this kind of content.
This tut is bookmarked and will be used some day in my new projects. Thanks
This is GREAT!
For long time I was searching for exactly this way of search - nice, clean and robust at the same time. Thanks for sharing!
Keep up with good tutorials Tzine!
Yet another great tutorial. Thanks and hope to see more of them in the future.
Absolutely brilliant tutorial as usual. One question, is there a way to change the Google API bit to UK instead of US? Thanks in advance and keep up the great tutorials =)
Very good tutorial again.. I'm gonna use it in my projects probably, thank you!
Great tutorial.
Having some issues though. Think they're with Google though as this tutorial works with every site except the site I need it to. The site I want to be able to search shows up in Google search results, but this search never returns anything.
Any ideas?
You can try running the query
on Google's main page. The script should return the same results that you get there. If the results differ, this could be something specific for the API. Unfortunately there is not much that can be done in this case.
I was worried about that. When I google something like "site:mysite.com search terms" I get the expected results, but the script doesn't return those results.
It's really strange, could be due to the fact this site is only 2 weeks old I guess. I'll re-submit the sitemap to webmaster tools to see if that helps any.
Either way, thanks for the tutorial Martin!
Just an update here Martin.
The problem I'm experiencing is apparently a known problem. I made a google groups thread about it here:
http://groups.google.com/group/google-ajax-search-api/browse_thread/thread/57edee1bcd923287#
Apparently the workaround is to setup a custom search engine and restrict the search API to that custom search engine.
Now to figure out how to accomplish that.
How could you go about using a lightbox on the image results?
very nice tut :)
i like it :)
Also I am noticing inconsistencies between stuff on Google and stuff being displayed here. I am doing searches that are showing no results in my engine but in google that same search shows up. Any ideas?
Chris, see this comment:
http://tutorialzine.com/2010/09/google-powered-site-search-ajax-jquery/#comment-10979
Great tutorial!
i can't believe google search can adapt with jQuery ajax...
its a rock
Really WOW! very nice
bloody amazing!
Awesome. Timely. Thank you.
What a great tutorioal...thank you very much. I did all as in the docomentation...but unfortunately it is not working...
Hope, you don't mind my asking but would you please check, where I did the mistake??? Otherwise no chance to find out...
Thanks for your time. Regards from Switzerland.
Christa
This is a fantastic tutorial. Thank you for sharing it.
What i´ve to do if the keyword should be in a normal font and not highlighted in bold (in the results)? Thanks ...
http://demo.tutorialzine.com/2010/09/google-powered-site-search-ajax-jquery/search.html
is this working for you ?
i see no results whatever i try
Try searching the web. The first option searches only within Tutorialzine.com.
Hi,
A great tutorial and very useful, is there a way I can add this to Mediawiki 1.15.x or 1.16.x ?
Will be very useful with mediawiki and also with wordpress.
Any tutorial or steps on that would be highly appreciated.
Kind Regards
Unfortunately I haven't worked with Mediawiki.
It should, however be the same everywhere. Just upload it somewhere on your site and replace the siteURL property of the config object in script.js.
You should be able to edit a template and change the destination of the default search form on your wiki. If you can, follow the instructions I gave J Roberts a few comments back in this thread.
Martin, thank you for this great and usefull tutorial, it is style-ish and simple yet incredible useful.
I already made Google API implementation in PHP but this is much faster and better lookig.
One question: how come google allows Ajax API without a API Key ?? Is it optional as my implementation is not working without the key.
Option to use MORE button can be replaced by page numbers + next/previous buttons ? I am JS learner now but just a hint will be much appreciated!
All the best, keep up the good work!
U.
The API key is optional, but the number of requests is limited. It is OK in this version, as it will count the limits towards the individual visitors' IP.
As for the pagination, the result set you are able to fetch from Google is also limited, so in fact only the first pages would return results.
Great, this is what I wanted to make for a phpbb forum.
I think I'll try now, because I found your tutorial.
This is real schnazzy. I always love your tutorials!
Final a search that is understandable and I can make it work. Thanks
Really great tutorial! Thank you!
This is really superb, I will make some changes in the code & design & host it on my website & use it as my default search engine its just so awesome
Keep in mind that -unfortunately- Google announced on Nov 1st that their web ajax api was deprecated so this code won't work in the near future. More info: http://news.ycombinator.com/item?id=1864625
It's amazing tutorial. Thanks alot!
Hi. I wanted to ask if this search engine works with static sites built entirely in html without php.
Yes, it will work. This is JavaScript powered so there wouldn't be any problems.
I don't understant how I set a "input type" in my home page and the result of the search in an other page. Can you help me?
Excellent script .. one question can this be used with adsense custom search engine? anyone here please advice .. thank you so much
I've used the above google powered site search with jquery, but it doesn't give me any results...it gives "No results found". I've assigned the siteURL to my website url in the scripts.js file.
Can anyone help...
Thanks in advance
Regards
Since Google web ajax api will deprecated is there a way to use this script with Google Custom Search Engine? Probably won't be an easy upgrade but something worth looking into. Anyways, amazing search script!
i have same problem is.........
i can't find google coad add my website to looking like this
"
{
"GsearchResultClass": "GwebSearch",
"unescapedUrl": "http://tutorialzine.com/2010/02/html5-css3-website-template/",
"url": "http://tutorialzine.com/2010/02/html5-css3-website-template/",
"visibleUrl": "tutorialzine.com",
"cacheUrl": "http://www.google.com/search?q=cache:_NSLxH-cQMAJ:tutorialzine.com",
"title": "Coding a CSS3 & HTML5 One-Page Website Template | Tutorialzine",
"titleNoFormatting": "Coding a CSS3 & HTML5 One-Page Website Template | Tutorialzine",
"content": "Feb 16, 2010 ... Here we are using the new version of HTML.."
}
"
PLEASE !!!!!!!
EXPLAIN TO ME HOW ADD FOR THIS SCRIPTS MY WEB SITE
It’s amazing. Many many thanks!
great tutorial. bookmarked!
Nice stuff!
How can I use only a specific TLD? (searching on google.ro not on google.com)
I see this tutorial is using the deprecated search api. Can we still use this, without a limited request per day?
How to embed Google Custom search into this ?
I have been looking for a tutorial like this. Can we also apply it on Joomla! type of sites? By the way, thanks for this post!
Just wanted to drop a note. This is using the deprecated API and it will no longer work. There is a new API however it is limited to 100/queries per day ($5/1000 after that if you have billing set up). And alternative is to use the CSE solution. It isn't as robust, but I don't think there is a query limit, and you should be able to override most styles.
Hello Martin,
Thanks for the tutorial, I've compiled it on a blog and slightly modify it, and it worked perfectly.
» http://assadsearch.blogspot.com/
You Rock Martin!!! ^_^
only support jQuery 1.4.2 for arrow effect...
ty ty ty
deuh assadotcom was landing here :P
how to use filetype: pdf, I need to do research in pdf format
// Adding the site domain as a label for the first radio button:
$('#siteNameLabel').append(' '+config.siteURL);
NO FOUND
How can i search only web and delete all radio button :)
type="hidden"
Replace line 24 with
<div id="searchInContainer" style="display: none">
and line 32 with
<ul class="icons" style="display: none" >
Note radio button for web must be checked like this
<input name="check" type="radio" id="searchWeb" value="web" checked />
and unchecked for searching www.yoursite.com
<input name="check" type="radio" id="searchSite" value="site" />
This was a really helpful tutorial for implementing a search Option within a limited span of time. Am very grateful that it was shared to us all the needful. Thanks a ton.
Does not work, api is depreciated
Thank you Martin, great help.. i have one question..is it possible to add code for
1.Show how mane results found
2.I turned on from googleCSEpanel autocomplete but it didnt work here...do have any idea that can help..to add autocomplete to this.
Thanks you,,waiting for your reply Martin
Thanks! You save my time.
I realize this is a slightly older post but this is why I'm asking...is there was way to integrate this with a custom search? Any thoughts or ideas would be appreciated. I want to use the custom search to define a universe of sites / pages to search against. But I'd like to have more control over the input page, as well as the results page.
I wonder if that system and a base of google.com / cse why I found it pretty cool the more I use cse advertising and wonder if it is to implement the system so I keep winning?
Hello Martin,
I'm thinking to to add ads in search results. Not any banner ads which depends on js and display on iframe. Those ads which google show in their custom search result page. I have adsense account. Can i integrate with the script?
Best regards and thanks for this great one.
Take care.
I am totally stumped. I thought I knew little bit about jQuery but I guess I was wrong.
I am trying to display <div id="resultsDiv"></div> on a separate page as the search result showing below the search box does not fit my website layout.
I thought if I change script.js line 78 to
var resultsDiv = $('result.html #resultsDiv');
this would show the search result on result.html. No dice. Totally stumped.
Can anyone help?
Thanks.
Trying to do the same thing... I ask but no reply...
I am thinking to add one line before line 78 that would open a new page and then append results...
Will try later today.
Yanik
How can I make to results be on a new page? Thanks for this awsome tutorial...
I figured it out ! You need to change this :<input id="s" type="text" /> INTO THIS <input id="s" name="terms" type="text" size="15" value="<?php echo htmlspecialchars( isset( $_POST['terms'] ) ? $_POST['terms'] : null); ?>" /></span>
into your search form frm your site , don't forget to redirect to the search results page
This will automatically populate the text field with the terms that were entered in the footer form. Now all that is left is to add this line to the bottom of script.js (inside document.ready):
$('#searchForm').submit();
The whole idea is to populate the form and fire a search when the page loads.
I am trying to accomplish the same thing, but am stuck on how to redirect the search results to search.php. A little guide please. Thanks.
I am trying to write all the steps that I've made. Now it works fine!
<form method="post" action="search.php">
<input type="text" name="terms" value=""/>
<input type="submit" name="action" value="search">
</form>
Then you have to change (into the search.php (the renamed search.html) ) this:
<input id="s" type="text" />
into this:
<input id="s" name="terms" type="text" size="15" value="<?php echo ( isset( $_POST['terms'] ) ? $_POST['terms'] : ""); ?>" />
You also have to put al the beginning of the search.php page this:
<?php
$terms = ( isset($_POST['terms']) ? $_POST['terms'] : (isset($_GET['terms']) ? $_GET['terms'] : "") );
?>
$('#searchForm').submit();
That's all!
Thank you so much for the tutorial! :)
I am trying to add this to my clients website, and I need a mini search bar in the header and the result page like this tutorial displays on a separate page, how do i do this?
can you give a demo based on google cse?thx.
hi good day, is a great script, but I wonder if it is possible to cast the results in a new page??? .. greetings and thanks
Great tutorial, thanks a lot :D
Fantastic tut.!!!! Thank you, thank you, thank you!!!! And thank you to all the people who commented.. you helped me solve the "how to enter a search from one page and display results on another page".
Cheers!
How did you do it? I am still trying to figure this out.
Martin Angelov can i ask you if you can add the autocomplete that google has to your plugin.
Great tutorial, congrats!
Is it possible to search both website and web with one input and display results on same page, website search results on the right, web search results on the left etc. ?
Hi there,
If I search the text "index" it shows my entire directory listing, althrough I blocked it through .htaccess.
Need help.
Regards.
Thanks a lot .. saved a lot of my time. (Y)
thanks for your efforts, I really like it.
I need your help,
I created an application for a company, and it is hosted on the company server and accessed from the intranet (offline).
by adding the Google search component, I found it doesn't work because it is not hosted on the internet.
How can I make it work?
thanks,
taraman
thanks :D
Hello,
Great tutorial and great webpage!
Just I have one problem, very big.
I tested the search plugin in a demo page into my site as subdomain (demo.mysite.com).
Now I delete the subdomain and I changed at all my new website (I uploaded a new page), but the plugin still show me results from the subdomain and from the old page. It likes it couldn't delete cache or cookies. I thought that this situation will be regulated in some weeks, but still continues the problem.
Please, can you help me?
Thanks
This does not work with URL's that contain Hyphens.
I Don't think it working fine
Just enter OR youtube.com
And see the result
Hi!
Excellent job, was exactly what I was searching (!) for. Cool design by the way.
So, thanks!!
Cheers
Here's the result (tweaked), just to show that we can do so many things with it, very easily!
Cheers!
Hey great tutorial martin!!!!
How do you make the web search the default search ???
Thank you !!
Hi, great work!
Can anybody tell me please how can I limit the search only for the main domain and not for subdomains?
Thabk you!
Does this work for non-database driven websites as well?
Martin Angelov google's web search api is going to not work anymore. Do you have another api that will work? Please Answer.
Note: The Google Web Search API was officially deprecated as of November 1, 2010. Per our deprecation policy it has operated for more than three years past its deprecation date. Its last day of operation will be September 29, 2014.
script.js:82 Error
Message : "Uncaught TypeError: Cannot read property 'results' of null script.js:82(anonymous function) script.js:82b jquery.min.js:124A.(anonymous function) jquery.min.js:125(anonymous function)"
It is working again. Is there any way to make this work with multiple sites?
Search works but the Images / News & Videos.
Search not working now i think google updated api for search. kindly check api code and update this code.
Search not working anymore i think problem in the API .