Timeline Portfolio
Timeline is a jQuery plugin specialized in showing a chronological series of events. You can embed all kinds of media including tweets, videos and maps, and associate them with a date. With some design tweaks, this will make it perfect for a portfolio in which you showcase your work and interests.
The HTML
Timeline comes with a light colored theme by default. It is perfectly usable and in most cases would be exactly what you need. However, for our portfolio, a dark design would be a better fit, so we will customize it to our liking.
First, let's look at the basic layout of the page:
index.html
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>Timeline Portfolio | Tutorialzine Demo</title> <!-- The default timeline stylesheet --> <link rel="stylesheet" href="assets/css/timeline.css" /> <!-- Our customizations to the theme --> <link rel="stylesheet" href="assets/css/styles.css" /> <!-- Google Fonts --> <link rel="stylesheet" href="http://fonts.googleapis.com/css?family=Dancing+Script|Antic+Slab" /> <!--[if lt IE 9]> <script src="http://html5shiv.googlecode.com/svn/trunk/html5.js"></script> <![endif]--> </head> <body> <div id="timeline"> <!-- Timeline will generate additional markup here --> </div> <!-- JavaScript includes - jQuery, turn.js and our own script.js --> <script src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script src="assets/js/timeline-min.js"></script> <script src="assets/js/script.js"></script> </body> </html>
In the head section, we have the plugin's stylesheet - timeline.css, and styles.css, which will hold our customizations. In the footer we have the jQuery library, timeline plugin and script.js which initializes it.
When we call the plugin, it will search for a div on your page with the ID of timeline. Inside it, it will inserts all the markup it needs to present the timeline:
<div class="container main" id="timeline"> <div class="feature slider" style="overflow-y: hidden;"> <div class="slider-container-mask slider-container slider-item-container"> <!-- The divs below are the events of the timeline --> <div class="slider-item content"> <div class="text container"> <h2 class="start">Johnny B Goode</h2> <p><em><span class="c1">Designer</span> & <span class= "c2">Developer</span></em></p> </div> <div class="media media-wrapper media-container"> <!-- Images or other media go here --> </div> </div> <div class="slider-item content content-container"> <div class="text container"> <h2 class="date">March 2009</h2> <h3>My first experiment in time-lapse photography</h3> <p>Nature at its finest in this video.</p> </div> <div class="media media-wrapper media-container"> <!-- Images or other media go here --> </div> </div> <!-- More items go here --> </div> <!-- Next arrow --> <div class="nav-next nav-container"> <div class="icon"></div> <div class="date">March 2010</div> <div class="title">Logo Design for a pet shop</div> </div> <!-- Previous arrow --> <div class="nav-previous nav-container"> <div class="icon"></div> <div class="date">July 2009</div> <div class="title">Another time-lapse experiment</div> </div> </div> <div class="navigation"> <!-- The navigation items go here (the tooltips in the bottom) one for each of the events --> <div class="time"> <!-- The timeline numbers go here --> </div> </div> <div class="timenav-background"> <div class="timenav-line" style="left: 633px;"></div> <div class="timenav-interval-background top-highlight"></div> </div> <div class="toolbar" style="top: 27px;"> <div class="back-home icon" title="Return to Title"></div> <div class="zoom-in icon" title="Expand Timeline"></div> <div class="zoom-out icon" data-original-title="Contract Timeline"></div> </div> </div> </div>
As we will be modifying the CSS of the timeline, the fragment above will give you a better idea of the customizations. Note that we won't be recreating the plugin's stylesheet from scratch, we will only be overriding some of the rules in our own css file. This has the benefit of making future updates to the plugin straightforward, not to mention that it will be much easier.
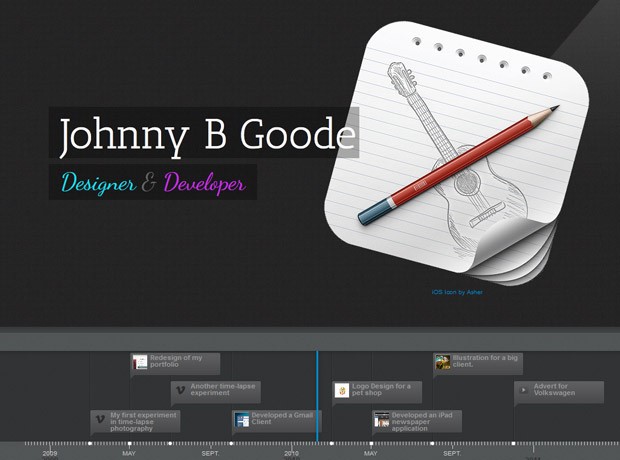
Writing the CSS by looking at the markup alone would be a tough undertaking, given that our rules must have precedence over the ones used in timeline.css. Fortunately, there is a much easier way, as you will see in the CSS section of this tutorial.
The jQuery
To initialize the plugin, we need to call the VMM.Timeline() method on document ready:
$(function(){ var timeline = new VMM.Timeline(); timeline.init("data.json"); });
The init method takes single argument - the data source. It can either be a json file like above, or a Google spreadsheet (reminiscent of our Spredsheet Powered FAQ Tutorial).
For more information on the supported data sources, see the documentation on the plugin's site, or browse the data.json file in the zip download for this tutorial.
The CSS
I used Firebug's HTML Inspector to get the right selectors for the elements that we are about to customize. In the HTML tab, it is easy to see what rules have been applied to each element by timeline.css. To override them, I copied the same selectors to styles.css which is where our modifications will take place. On several occurrences, however, I have used the !important flag to make my work easier.
All the customizations you see below override only a handful of CSS styles. The rest are inherited by the default stylesheet. Let's begin!
The first thing we will do in styles.css, after styling the page itself, is to change the backgrounds of the timeline:
#timeline{ background:none; } /* The individual events in the slider */ .slider .slider-container-mask .slider-container{ background:none; } /* Setting a custom background image */ #timeline div.navigation{ background: url('../img/timeline_bg.jpg') repeat; border-top:none; }
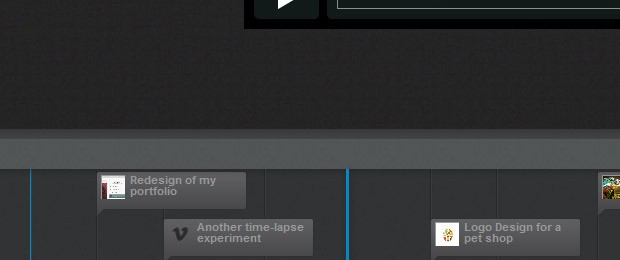
To create the 3D effect of the timeline navigation, we will need to use additional elements. But the Timeline plugin doesn't include such in its markup. An easy solution is to use :before / :after pseudo elements. The :after element is the darker top part and it uses a linear gradient to enhance the effect.
#timeline div.navigation:before{ position:absolute; content:''; height:40px; width:100%; left:0; top:-40px; background: url('../img/timeline_bg.jpg') repeat; } #timeline div.navigation:after{ position:absolute; content:''; height:10px; width:100%; left:0; top:-40px; background:repeat-x; background-image: linear-gradient(bottom, #434446 0%, #363839 100%); background-image: -o-linear-gradient(bottom, #434446 0%, #363839 100%); background-image: -moz-linear-gradient(bottom, #434446 0%, #363839 100%); background-image: -webkit-linear-gradient(bottom, #434446 0%, #363839 100%); background-image: -ms-linear-gradient(bottom, #434446 0%, #363839 100%); }
Then we add a dark background to the timeline navigation (the section with the small clickable tooltips that represent the events):
#timeline div.timenav-background{ background-color:rgba(0,0,0,0.4) !important; } #timeline .navigation .timenav-background .timenav-interval-background{ background:none; } #timeline .top-highlight{ background-color:transparent !important; }
Later we style the zoom-in / zoom-out buttons and toolbar:
#timeline .toolbar{ border:none !important; background-color: #202222 !important; } #timeline .toolbar div{ border:none !important; }
The numeric scale at the bottom comes next:
#timeline .navigation .timenav .time .time-interval-minor .minor{ margin-left:-1px; } #timeline .navigation .timenav .time .time-interval div{ color: #CCCCCC; }
The previous and next arrows:
.slider .nav-previous .icon { background: url("timeline.png") no-repeat scroll 0 -293px transparent; } .slider .nav-previous,.slider .nav-next{ font-family:'Segoe UI',sans-serif; } .slider .nav-next .icon { background: url("timeline.png") no-repeat scroll 72px -221px transparent; width: 70px !important; } .slider .nav-next:hover .icon{ position:relative; right:-5px; } .slider .nav-previous:hover, .slider .nav-next:hover { color: #666; cursor: pointer; } #timeline .thumbnail { border: medium none; }
The loading screen:
#timeline .feedback { background-color: #222222; box-shadow: 0 0 30px rgba(0, 0, 0, 0.2) inset; border:none; } #timeline .feedback div{ color: #AAAAAA; font-size: 14px !important; font-weight: normal; }
Then we move on to the slides:
#timeline .slider-item h2, #timeline .slider-item h3{ font-family:'Antic Slab','Segoe UI',sans-serif; } #timeline .slider-item h2{ color:#fff; } #timeline .slider-item p{ font-family:'Segoe UI',sans-serif; } #timeline .slider-item img, #timeline .slider-item iframe{ border:none; }
Finally, we will customize the appearance of the front page. I am using nth-child(1) to target only the first slider-item, which contains the name and description of the timeline which have been defined in the JSON data source.
/* Customizing the first slide - the cover */ #timeline .slider-item:nth-child(1) h2{ font:normal 70px/1 'Antic Slab','Segoe UI',sans-serif; background:rgba(0,0,0,0.3); white-space: nowrap; padding:10px 5px 5px 20px; position:relative; right:-60px; z-index:10; } #timeline .slider-item:nth-child(1) p i{ font:normal normal 40px 'Dancing Script','Segoe UI',sans-serif; background:rgba(0,0,0,0.3); white-space: nowrap; padding:5px 20px; position:relative; right:-60px; z-index:10; } #timeline .slider-item:nth-child(1) p .c1{ color:#1bdff0; } #timeline .slider-item:nth-child(1) p .c2{ color:#c92fe6; } #timeline .slider-item:nth-child(1) .media-container { left: -30px; position: relative; z-index: 1; } #timeline .slider-item:nth-child(1) .credit{ text-align: center; }
The only thing left is to open up timeline.psd that is bundled with the download of the plugin, and change the color of some of the icons in photoshop. I have included the necessary files in the zip download for this tutorial. With this our timeline portfolio is complete!
Done!
You can use this portfolio to display not only your recent projects, but also interests and important moments of your career. Share your thoughts and suggestions in the comment section.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
This is just awesome!!!
Daaaaaaaaaaaaaaaaaamn!
It look's great! You are pro Martin!
That's funny, I was searching for a tutorial to create a timeline a few minutes ago ;-)
Wow, I hope I can find a use for this :)
Martin, you have surpassed yourself on this one. Everything about this tutorial is truly stunning.
Doesn't work if I have more than 3 elements starting at the same date, it only displays 3 of them.
Is is possible to add a vertical scroll if the height of the timeline is to big?
Thanks,
This is the default timeline behavior, but the previous / next arrows still work so it is usable.
This is amazing bro
If this could be turning into a Wordpress plugin it would be GOLD!!
Matt, if you haven't found it yet there is a timeline for WordPress: http://wordpress.org/extend/plugins/wp-simile-timeline/. I've used it to create a timeline for a client here: http://www.davidminch.com/new-york-architect-timeline. It's not easy to make it useable for a short duration of time though it's a starting point. Every time I upgrade the plugin the layout breaks... I have some cleaning up to do on it.
Did see the library today and had an idea for making a tutorial.. Funny to see it here now ;)
Nice article
Awesome tutorial. Great job mate.
Hi found this plugin the other day this is a great demo of it thanks a lot.
This is aWesome idea + aWesome work...
Hi is there a way to display from the newer events to the older??
Regards
Modify data.json and replace "startDate":"2009,1" on line 7 with a year and month after your last event. This should make the timeline show the newest events first.
Hi Martin, thank you for the tutorial, works a treat and looks great.
I am also trying to get the items to appear in reverse and followed the suggestion:
> Modify data.json and replace "startDate":"2009,1" on line 7
> with a year and month after your last event.
I have modified line 7 to:
"startDate":"2019,1"
This moved the opening page to the end of the sequence and then started the timeline at the next item:
"February 2009
My first experiment in time-lapse photography"
and then the later events after this, just as before the modification.
The title page also shows in the timeline then when you reach it.
Have I misunderstood something here?
Thank you for your help
ice70
I dont know if its me or what but I download the demo and when I run it only says Loading Timeline... Why?
Regards
Make sure you are running the demo through localhost, and not opening it from the folder where you've extracted it. You will need to run XAMPP / MAMP for this, otherwise you will face a security exception for accessing a local file.
lol I was wondering about this too =/
This is awesome, I actually had a lot of fun reading through the timeline and almost forgot about the tutorial.
Hou can i start the timeline a little more expanded ?
Expanding the timeline on load isn't mentioned on the site. It also doesn't look like this is supported from the unminified source code.
Your best bet would be to simulate a click on the enlarge icon after the plugin is initialized with the .click() method.
How would using .click() work? Rather, I know just enough to back myself in a corner, and not enough to actually figure out what and where to use it to solve my current dilemma.
Any response would be appreciated, even if it's just a point in the right direction
You can set the following in your configuration while passing to CreateStoryJS() method
start_zoom_adjust: '-10'
Hi Sethupathi, could you please explain in little more detail how to implement this in timeline portfolio code?
Hi Vitor
Did you have a chance to sort this one? I would like to simulate at least 3 clicks... but no idea where to start...
I too would like to expand the timeline by default, is this possible to explain how to do so?
Thank you!
J
Can you change the timeline to start in reverse chronological order from left to right? In other words, can the timeline start on the left side (as it already does) with the current date and end with the earliest date?
I know you can check the box in the Embedded Generator to "Start at the End" but that just moves the timeline to work from right to left which seem cumbersome since we are accustom to working left to right.
Master piece!
This is amazing!! And it works great!
But I've got a problem with the Google spreadsheet, if i add the url to:
timeline.init("myurl");
When I run it only says Loading Timeline...
Do you have any idea?
This Problem is only with IE9, is there a solution?
I haven't tested it with a spreadsheet. I haven't touched anything on the plugin so you are seeing default behavior. Opening up your browser's console might reveal useful debug messages.
This incredible! Can't wait to start playing with this!
I love !
You'r the best man !
Good one, Martin !
Very very innovative approach. I have been researching a new portfolio layout recently, and this may have just won out over isotope/quicksand.
Cheers!
Fantastic work!!
Awesome tutorial...
You are amazing , this is awesome!.
I think it would be nice to add a feature that stops the video from playing if they click to the next timeline, other than that it's awesome as always!!! Thanks for the post!
Excellent work again ;)
Hello, i have a question, i want use this timeline for do a timeline but based on 2 hour only, so i have try date as that :
2012,1,06,12,01
2012,1,06,12,05
2012,1,06,12,06
2012,1,06,12,10
2012,1,06,12,30
ect but i found a problem , the bar in bottom is very compressed hour on hour
How i can this work extended into minutes in 2hour ?
Thanks it s a very good script
And then how i can put hour in french format ? thanks
Awesome one!! Perhaps this indicates that you are indeed one of the finest web designers we have....Thanks for the tutorial
The script is awesome!
I have two questions though.
Can I start showing events from the last one in the timeline to the first? like 2012 events show on load and then move back on the timeline to see others? Or, change the direction of time, like reverse the timeline?
Second one, can you change the default zoom in level? Cause I have 10 events in 2010 and one event in 2000 so it looks weird!
Both of these questions were answered above.
start_zoom_adjust: '-10', I have explained above
Thank you for the awesome comments!
hey,
great job. Can you also do this for Joomla 1.5 & 2.5 it would totaly fits in my website.
Thanks a lot. You can also answer on my email adress.
I love this! thank you for sharing your knowledge!
Martin: thanks for putting this delightful demo together. I wonder, have you thought about how it could be modified for mobile/tablet browsers? If you shrink the screen window on the demo currently, the timeline disappears completely, the graphics do not shrink to fit, and the next/back nav also disappears.
kind regards,
AndyBean
Hi, great timeline plugin, best I'v seen so far! Looks and work great on Ipad, the jquery transitions are not jaggy wow! My question is how to translate the displayed dates to another language like french? Thanks and really looking for the next updates.
the timeline is awesome but funny enough its NOT LOADING on Google Chrome
Hi Martin,
Is it possible to pay you for customization?
Brilliant tut!
A question, though:
what's the name of the font you use in the "Timeline Portfolio" and "Johnny B Goode"?
Thank you!!
its out of the world. superb.
Perfect! ThankU so much!
Great tutorial and impressive portfolio.
I do have some questions that I hope Martin or someone from the comments can answer.
I would like to have the date displayed in the way it's displayed in many countries besides the US and Canada: How do I change it from displaying i.e. November 8, 2000 to 8 November, 2000?
Also, how do I translate the months? For countries where the months are spelled differently).
I also need the date in "day - month - year". However, excellent tutorial!
Yes, I need to translate it too to day-month-year. I LOVE this tut and the TimelineJS from VeriteCo <3
wow :D
I appreciate this a lot, I've been looking for a good timeline for ages, and this is perfect! Is there any way to enlarge the space between events? Some of them can't be read because they're too close.
I'm impressed, this timeline style is so fucking AWESOME
Thank you!
Hi,
Is it possible to display images in timeline from sql database?
if yes, plz explain me..
Hi there
On my PC downloaded demo works fine. But when I've uploaded it onto web:
Can you help, please?
Love the Theme! Really Wana Use it! Just wandering is there was a way to implement a thumbnail selection for images on one of the slides, so each slide represented a set of images. I'm just trying to think of wait to show my Photography work without having dozens of slides. Thanks for the Theme!
Hi, thanks for this awesome tutorial. I just have one question if you could help :
I will like to put some HTML instead of images,videos...in the media section. Could you tell me how could I do ? Because I tried but it's not working.
Thanks
Hey this is awesome!
Do you know if it's possible to add markup inside the 'media' tag? I'm trying to embed a video instead of linking to it
Cheers
This Timeline portfolio is way better than the ones I've seen lately. Thank you very much for this great tutorial. Although I have some questions in my mind:
Best regards, Anthony!
Good Job!! It's possible link to single slide?! Tanks..
I just saw something similar the other day but this is much nicer for full width as well as a little better in design. Great implementation!
Perfect -----
Could you please help me.
I can't seem to figure this out. The page keeps saying Loading Timeline.
You can see it in http://ecom.freshweb.com.pt/timeline/
Hello Abel... i've just checked your link and it's working... how did you fix the problem?
How to create a schedule from the earliest date to the oldest?
Timeline:
> 2012 ,05
> 2012 ,04
> 2011 ,09
> 2011 ,06
having trouble with Google Spreadsheet data soure
won't load timeline in any browser.
Looking at the console:
VMM.Timeline.TimeNav
timeline-min.js:1init
timeline-min.js:1DATA SOURCE: GOOGLE SPREADSHEET
timeline-min.js:1["key", "output"]
timeline-min.js:1Uncaught TypeError: Cannot read property 'length' of undefined
ideas?
Same here...
Uncaught TypeError: Cannot read property '$t' of undefined
Its a Great Plugin. I thought of Presenting my CV ina Timeline format for which i thought of creating my own timeline.
But you made me totally upset(just kidding). This is what i was planning to do.
But you Won!.
Great Job and Looks very neat.
how I can change the language of the time?
Hi joenhe,
ok, i found
In order to change the language of the date/time, it's necessary to modify the file timeline-min.js
You can change the structure .. otherwise you can also translate each word in your favorite language
Go to http://timeline.verite.co and download the package.
There is one example of this.
Finally!! This is what I've been looking for! Thanks Martin for this great plugin.
About the reverse mode, in the full javascript file (not minifield), there is a option in config var, "start_at_end". Change for true, then the timeline goes to the end.
Bye guys!
Hello, I would like to know how to change the months of the Spanish language.
thank you very much
What a fantastic effort. Very cool.
Couple of things though: can we make the time line in reverse chronology? Latest first..
Secondly, it would be great if this were "responsive". To be displayed on all devices, especially tablets!
Hi,
Can I implement this to my tumblr? Please email me. Thank you. :)
this is just awesome. Thank for making such a great portfolio example out of timeline. I was searching for exactly the same stuff.
U guys are the best! I needed time line on my website but I opened your website to look what you guys have published new.. and voila.. I found the thing that I was looking for the most.
How do I change the date format for day, month and year?
Wow,It's perfect
My timeline is not loading there's only the text "LOADING TIMELINE" but nothing is happening.
Ok, I found the error, I had at the end one comma.
Great script!
I've plotted several events that span over a period of 6 months - the events looked squished in the timeline, but great if I manually zoom in.
Can I set the zoom to be in further?
Steve
This site is amazing.. Thanks a lot
Just wondering, any thoughts on how you can default the timeline to todays date (or nearest event in the time line to today)?
Fantastic site and full of excellent examples and resources! Great job.
This is a great library, I appreciate your wonderful work! I am wondering if it's possible to show the timeline in just half the window size? I seems to me that the video thumnails have a minimum height (To me, it's like 402px). Is there a why to get over this?
Thanks.
Couple of things though: can we make the time line in reverse chronology? Latest first..
Hi Martin,
I've tried to reverse the chronology of the timeline by following your instructions:
"Modify data.json and replace "startDate":"2009,1" on line 7 with a year and month after your last event. This should make the timeline show the newest events first."
It didn't actually work. It would be good to keep the Johnny B Goode intro page and have the timeline be from most current on the left to oldest to the right. I tried reversing the years under the startdates as in latest first, but that totally messed up the timeline.
Please advise what I need to do to reverse it. It seems others have the same question as well?
Coolest thing you did, wish I was a genius like you!
Thanks!
About the reverse mode, in the full javascript file (not minifield), there is a option in config var, "start_at_end". Change for true, then the timeline goes to the end.
Bye guys!
Hi SA.
I'm not really the sharpest javascript coder, so I can't really seem to locate the full JavaScript file which is needed to change the "start_at_end" config var.
Could you instruct me as to how I can find this full javascript file?
(Sorry for the rookie question)
just add this : start_at_end: true
Hi,
Ive downloaded the demo and uploded to my server, but it doesn't work. Just shows loading timeline.
Any idea why this happens?
regards,
Sasee
First, thanks so much for making this tutorial.
I'm having some issues (probably just a lack of knowledge) but if anyone can offer up some help I'd appreciate it.
1) I basically want to wrap the entire timeline with my own graphical border. I have tile-able images that I basically want to have as a border for the timeline. I cannot seem to figure out how to have them display ontop of the timeline. When I refresh the page, the top border displays, but then the timeline over takes it. I have the z-index set at 1000, but still no go.
2) My timeline needs to display clock time. I add the time to my json file, and it's displaying it fine it seems, but it doesn't show 30min increments. Just 1hr. It also doesn't seem to do AM and PM. So, 1pm is shown at the beginning of the timeline before 3AM (both are the same date).
Got it all figured out. Thanks
Hey man could you share your solution? I would like to display clock time also and it would be great to have some info before i start. Thanks in advance
Sure.
I'm using JSON as my data.
It was merely changing the "startDate":"1862,3,8,14,20", format.
The format is: year, month, day, hour, minute
Hi,
How do you display current date & time on json file?
Thanks
Fantastic design and great that you shared it with your users. This is something unique concept I've ever seen. More than just awesome!
impressive !
It dose work in Chrome (YES Chrome) but I noticed it has to be on-line to work, but for testing and building off line, you can use Netscape... I mean Firefox, LOL.
This is not a golden answer, I just noticed once I uploaded it; it started working in Chrome, so this tells me the files I downloaded DO WORK!. :)
So build locally offline in Firefox, OR build your example on-line Remotely; so as your changers are saved it uploads the copy to you server, and tests it in Chrome all in one shot.
PS. you can use EI for offline testing (it works) but why would even turn that Browser on? and you are building off line grab all the Remote files and change all the links (in index) to be local...
"startDate":"2013,1,10", yes we can add day of month also
Thank U
Martin,
Very nice work. I'm not a graphic or web designer, but this plug-in holds promise for presenting events in a chronological fashion. I work in the legal community presenting evidence inside the courtroom and at mediation. Is there any way to format the date in the plugin so that it could display specific dates (i.e., October 6, 2009 instead of October 2009)?
Great work ! Martin!!
This is the very new and fresh idea and design to me.
Thx for the information=)
Great tutorial for a great plugin, picking up on other comments, it would be fantastic to see a solution (as in a snippet) to set the expand level on load.
Hello,
Thank you for this awesome tutorial, I would like to change the name of the author "Johnny B Goode" it is in h2 of the the HTML, but when I open my index.html file, there is not h2 at all, how can I change it please ?
Thanks for reply.
on the data.json
Amazing tutorial martin
Thanks for the tutorial
I have a question though . I see that the order of events are in ascending from 2009 - 2010 , how do i make it in descending order
Hi
Great work man.I just need a little help from you with this timeline.
I want to display more than one image for the same event.How to do it??
I really need this work to be sorted out as soon as possible.
Thnx in advance
Is there a way to include multiple images per date? Like have a small showcase or open a query gallery of sorts?
Hi Martin.
Thanks for the tutorial, great job!
I have a question, how can I control content container box width of the particular event at timenav part in sync with event-line, in other words if the event lasts 6 months, I want to show the box width is extended across 6 months.
thanks a lot in advance
George
Hi everyone!
I'm quite new in CSS and all this world. I'm workin' on a website because at last our webmaster had something else to do (don't know what) so i'm trying to fix a website. I really like this tutorial because it's perfect for what i have to do. Just some problems: i tried to modify the html code in order to update text and images/video, but it doesn't work. Anyone can tell me where the problem is?
Thank you so much for reading!
A fantastic timeline solution... And some great development by end users here. Love the darker theme Martin and downloaded it as a starting place for my timeline. Thanks for sharing.
I wish to use the timeline and 'package' the end product onto a data CD/DVD to send to non computer literate relatives overseas so that they can simply open the index.html in their browser regardless of Internet connectivity i.e. run it completely offline
Can readers offer advice whether this is possible with the timeline data held in a json file and associated images and videos saved on the same disc? Will the browser deal with the js content or does it need server side processing of the json data?
I'm not a developer but an enthusiastic user of Dreamweaver 5.5 so can follow some degree of technical advice. Only ever done static HTML and flash before.
On a related note, I'm currently struggling to run it in local host as wampserver 2.2 freshly installed doesn't want to work. Though I can see my timeline building in the Live view window of Dreamweaver I can't see the finished article previewed in Chrome as I would like. I will persevere, it appears that apache doesn't want to start or my local host settings are wrong! Can anyone recommend the best way to run a local server in Vista.
Exciting stuff guys, keep sharing! Well done Martin!
Darren
I love your timeline ;)
Hello Martin,
Thanks for your wonderful tutorial. It is very well conceptualized and written and found it very useful.
I have the same question similar to others, how to do the timeline in reverse order. The answer you have suggested dint help. Might be we have misunderstood. Can you share us the demo for the same. "start_at_end" will still have it in the same order but just zooms to the last event. Modifying "startDate" dint seem to help.
Also, assume the timespan is for 12-15 years, as we zoom into the year, the timeline lables doesn't show month labels. Any idea how we do this? Your help is much appreciated as it helps most of the people who have posted similar comments.
Thanks,
Krishna
Hi Martin,
Awesome work martin but if we have less then or equal to three events in our timeline then it will break. Can you please tell me solution of this problem?
Thanks.
How would I be able to put this timeline function within a page on any of my sites current pages?
Hey its a very good control and very usefull for my application.
But in my application I want to display the timeline scale i.e. "Navigation DIV" only means I want to hide the slider above that timeline scale i.e. "feature" DIV.
For hiding that I have written the following code :
timeline .feature{width:100%;display:none;visibility:collapse;width:0px;height:0px}
By putting this css the feature div got collapsed BUT the Problem is that the "MOUSE DRAG GOT STOP" in the Navigation Div Timeline scale.
Please help me out!! Is m doing something worng or I have to update some more css to achieve the same.
Thanks in Advance!!
Hey Can I get the full version of TimelineViewer.js coz in download zip there is minified version (timeline-min.js) of js file.
hey everybody - the FIRST WORD at the top of this page is Timeline - the link to the timeline code and all sorts of info - can nobody read?
Timeline (http://timeline.verite.co/)
is a jQuery plugin specialized in showing a chronological series of events. You can embed all kinds of media including tweets, videos and maps, and associate them with a date. With some design tweaks, this will make it perfect for a portfolio in which you showcase your work and interests.
http://timeline.verite.co/
Hello All, I am using this timeline viewer in my application and I am facing some issue with that "Month and day labels are not shown when the time difference is comparatively higher".
for example, the day label is visible if the time period is in the range like: start date 2011,12,25 end date 2012,1,26 but if the difference if large for example
start date 2011,6,25 end date 2012,1,26 the label for the days is not visible when we zoom in the timeline to the maximum extent.
Same is the case when the year gap is large the months label are not visible.
Please help me out!!!
Thanks & Regards,
Abhishek Goel
Thanks got only one question. This works for years and month. But I want to make one time line that only gos for 2 months so I want to work like: 2012, july, 23. Can I do this on this script? pls help and thanks
Awesome demonstration of using this timeline control.
Is it possible to dynamically add events using javascript? I am asynchronously fetching data from several sources and need to load these as they arrive to my timeline.
Any pointers on this really appreciated!
Hi! the timeline work on my mac, but online it dosent work i just change images but when i upload it to the server the web page just say it´s loading. What i can do?
Hello!!
do you have the solution?
I have the same problem.
Would you help me?, please
thanks
Thank you SO much for this!! I've seen a Wordpress theme some while ago that uses this in a drawing/art portfolio theme, I was wondering how it was done and here's the answer! Thank you so much! I just had my web developer's "ah-ha!" moment! =)) Knowledge is amazing!
Can we have items set by day?
If I am not using timeline-min.js, and using un-packed version ie, timeline.js... its not working! :(
please someone help me
how combine the timelineJs with a database? thanks for the answer :)
How do you add scrolling just to the text container that is on the left side of the image area. I tried adding overflow: auto and scroll to various classes int he style and timeline css and it did not work.
awesome !!!! pure creativity and beautiful implementation !!!
Love the idea, would like to use it for my own portfolio timeline. I am having trouble with the dates. When I change the date.json file to start in 2006 the bottom dark numbers don't change and the hash marks don't move to 2006. I looked at the file in firefox with firebug and I can see the "class="time-interval-major" in firebug but where is it in the html file? I am using Dreamweaver to make my edits.
Hi is there any way to load data from a JSON object or a web server json response?
Is there a way to set the width and height of this timeline, so you can include your site design's headers and footers?
i'm having the same problem
Freaking Awesome!!!
Thanks a lot bro always wanted to create a timeline using Dojo but this is superb gonna convert it to a Zend Application, my aim is to create an anti-police website with a timeline of people killed by the police...
Hi,
I know you mentioned to use XAMP/MAMP...however, when I post on our IIS server and ping it through the browser, I'm still seeing Loading Timeline.
I'm planning on using this on our Intranet site, so should I tell our network admin to flick a switch, or can I do something on my end?
Thanks, and great work!
Peter
It's amazing! You are great Martin!
..but what can I do if I need the translation to Spanish?
and what happend if I need that it works with Google's spreadsheet, what is the code and where I have to put this code??
Thank you very much!!!!
Great work Martin, Great Design and Great Functionality.
I have a question though. The timeline is working perfectly and i have added my data into it. I would like to know if the heading and the text in each slide can be made to a hyperlink. So that it will link to the orignal source?
How would i put a pdf file or text as the content instead of a video or image?
Could anyone tell me how I would change the size of the timeline? I want to put it inside a box. I tried creating a separate div and putting the code in there but it doesn't work. Any help would be appreciated. Thanks!
just shocked again, u rocks man!!!, it's really awesome.... tnx a million
Thanks a lot
Hi,
Great work Martin,
your code works correctly on a small file (data.json) but it displays loading timeline when the file is large (136k). Have you a solution so that I can display the timeline if the file data.json is large because it contains a large text ("text":" ",)
Thanks.
Okay, I've got the dates translated. Now I have to set the order from Augustus 25, 2012 to 25 Augustus 2012... Sniffin' around in the mess of timline-min.js ... ...
I see several request about zoom level at start. The answers however are very strange. Not one is pointing where to put what code. Example: Configuration file??? where it is? Of course easy if you know where to put it in what code format, but please.... give us a good example!
Hi
I m New to PHP and I want create a website that search a product from a different website (choice of client) But I have no Idea plz Help me
Thanks in Advance
One more question, I want to post some entries in the January month but it doesn't appear. What lines should I change?
Hello Martin,
This is a great tutorial! But i'm working with google docs and import my google docs sheet into the timeline. (Otherwise it wouldn't fit on my page) but i wanted to color it black. Can you do this?
Because if i use your tutorial it doesn't fit and it crashes :(
Cheers,
M. Willems
hi, i need to use accentuation in texts and use other special chars. in mine texts are 'disformattig', strange chars. any suggestion? i already try o tchange utf-8 to iso, but have no sucess. thanks. cool job!
Hi
how can I set the timeline to 2000 b. c.
is that possible? or is not.
please help me
make the date negative. for example, -2000 should be 2000 b.c
hi, i make some changes and in IE and chrome are ok, but in FF only 'loading...'. any idea? tks.
Hi Martin.
Realizing that you wrote this tutorial quite a while ago, by any chance, do you know how to change where the media shows up on the timeline? You have it in various places on yours (left, right, below) so I thought perhaps you could explain what you did to achieve that. On mine, it only shows up on the left side. (for example: http://johnsonandbell.com/timeline-test/#11) and if I can only have it one way, I'd really like it to be on the right side. Is the bottom placement only available when you don't have body copy?
I'd appreciate any help you can provide. I've posted to the GoogleGroup for TimelineJS (VeriteCo) but to no avail.
Hi, saw that in your demo the timeline has dynamic height. In the original implementation it doesn't, where I can find that piece of code, is it css or jQuery?
hi
martin,
this is awesome timeline example. i had use this in my website. but i have a some problem. its working fine when its online. but when i published it on server its not working. just display "loading time"....any body can guide me please?
thank you in advance.
Excellent work man.
I have downloaded it and reviewed it on Google Chrome but unfortunately its not work for me. I was missed something? Please help me on this. Its working on all other browsers except Chrome.
Hi,
Thanks! it is very use full for me. I have some problem while running it with Google Chrome. It only says "LOADING TIMELINE', while I opened an error console it says:
"XMLHttpRequest cannot load file:///C:/Users/GGPatel/Downloads/timeline-portfolio/data.json. Origin null is not allowed by Access-Control-Allow-Origin."
Can you please suggested why this is happening.
Note: In all other browser than Chrome it works fine.
Thank you,
Gautam.
I am having the same issue, have you figured out how to get yours to work?
This does not load in chrome. has anyone found the solution?
Thanks
I want integrate it with: https://github.com/hidabe/portmagic
Hello, I have problem with the
"startDate":"2010,2,10",
its outputting to March 10, 2010 instead of February 10 2010. It only does it after line 268. If I change the start date before that line to February it works. Is there something you help me.
thanks,
Marie
Hi.
Thanks for the great tutorial. I am using it on www.festivalabierto.com/timelineFA/
I noticed a bug though. When I added youtube content the timeline freezes when I try to go forward to a future date or backwards. The entire page freezes up with non of the clicks taking me anywhere. Is this a bug? How can I fix it? Has anyone had issues?
I can't get make timelineJS work in remote server. Only local. Local is all ok, but when i put in remote server stay ever with the loading timeline and nothing happens. Anyone can help?
Hi Martin,
Great tutorial, but I have a rather earthly question for those non-techies like myself: <b>how do I implement this in a WordPress environment? </b>. I've tried using several adapted WP plugins for VéritéCo's timeline (such as timeline-verite-shortcode.0.9.7, even Vérité's own TimelineJS-Wordpress-Plugin-master) but they're all full of bugs which require -as per their forum responses- too many tweaks that I am not capable of doing (I don't know JS or PHP, simply a bit of HTML).
<b>So if I wanted to add your code to a WP page, how would I proceed?<b> -please use an idiot-proof response :)
I know the Google spreadsheet solution for data is very convenient, but if necessary I don't mind editing a raw data.json file.
Any hint/tips will be greatly appreciated!
has anyone actually got this working in inverse order. (newest first) i've tried everything on the comments and the plugin site doesn't really have much of config.
Not sure what I'm doing wrong
Please advice THanks
I had the "loading timeline" problem as well.
In my case I was able to find out that the page did not find the data.json file because my web server (I was running IIS locally) didn't know the ".json" extension/MIME Type.
So I added it as "application/x-javascript" and all was solved!
Hope this helps!
Hi Paulo,
Can I ask where you added "application/x-javascript"? I think I'm having the same issue as you were, I'm new to web developing so not really good at understanding a lot of the terms being used.
Thanks heaps, Ben.
It is easy.
"application/x-javascript"
IIS doesn't recognize file of type .json
Solution
Add a MIME type to IIS (at server level)
To allow it to serve that type of file.
Open IIS Manager and select server properties
Click the "MIME Types" icon
Click "New"
Enter Extension = "JSON" and MIME type = "application/json"
thats it and it works seamlessly
Nice tutorial .... I used this in my site
Very well done mate, very impressive and I'm definitely going to use this!
It seems Timeline.js does not appear to work with versions of jQuery > 1.8.3. Took a bit to diagnose this issue as we are using jQuery 2.1.1 with some other libraries. This causes the "Loading Timeline" message without actually loading. Haven't determined a workaround.
Awesome ! I need a Wordpress theme with this plugin ! <3
If anyone is having trouble with using this in chrome, it is because chrome is unable to access localhost for the data.json. This could be easily fixed by either hosting your website on a web- server, or by hosting the .json file itself.
Basically, go to myjson.com and paste the data.json contents into it. The website will host the .json file and give you a link to access it. Afterwards, go into your script.js, and change it to
timeline.init("link to .json file");
Hope it helps :)
Hi
I really want to use this timeline, it is exactly what I need.
I see that the post was published in 2012. what's the compatibility rate now and in the future ?