Neon Text Effect With jQuery & CSS
Tutorialzine is the home to some of the best web development tutorials on the net. Our focus has mainly been on the coding side of web development, but often creating a good design is equally important.
This is why today we are going to do the first tutorial on the site, which is both design and code oriented, to bring you the full web development experience. Share your thoughts on what you think about this new format in the comment section below.
Step 1 - Design
Today we are creating a neon glow text effect with CSS and jQuery. The first step to achieving this effect, is to create a background image, which contains two slightly different versions of the text. jQuery fades between those two versions creating a smooth transition effect.
To create the colorful background image, you first need to open a new Photoshop document 650px wide and 300px high, with a background color of #141414. Use your favorite typeface to write your heading. I used Century Gothic with a size of 60px.
After this Ctrl-click the text layer's icon in the layers panel to select it.
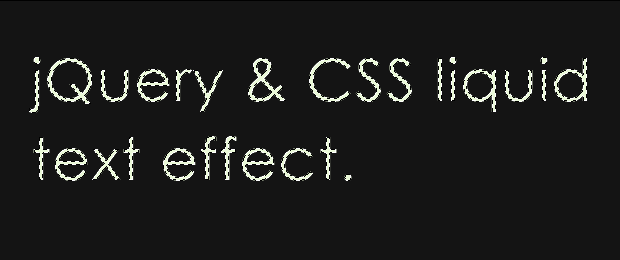
Use the Rectangular Marquee Tool while holding Shift+Alt to limit the selection to a single word of the text.
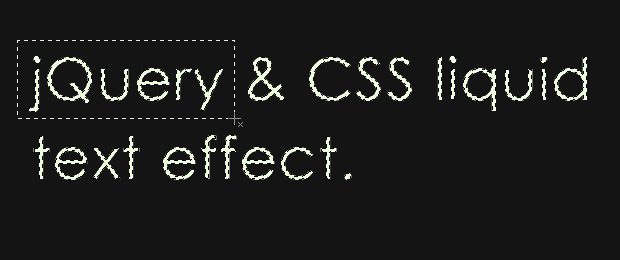
While everything is selected, create a new layer, name it gradients and make it active by clicking it.
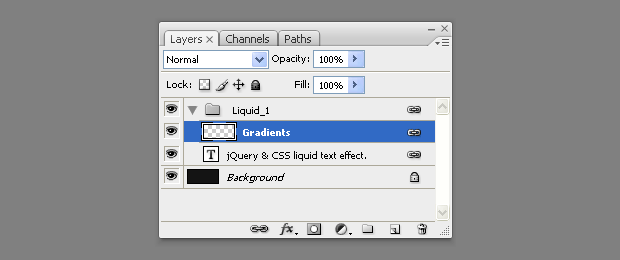
Choose the Gradient tool from the toolbox, and color the words one by one using the technique discussed above to switch the selection between the individual words. These selections limit the effect of the gradient tool and with the "gradients" layer being the currently active one, all changes made by the tool are saved there.
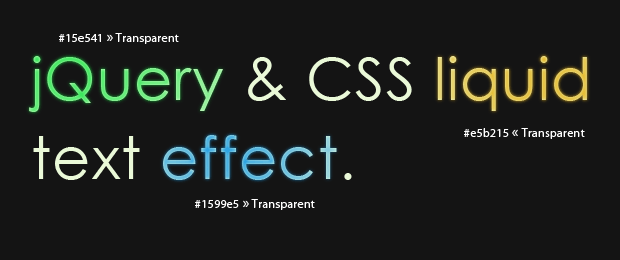
After you finish the heading, duplicate it below the original and apply a different set of gradients. Align the two versions of the colorful heading one above the other, so that it is easy to switch between them by simply providing a different value for the position of the background image in the CSS part.
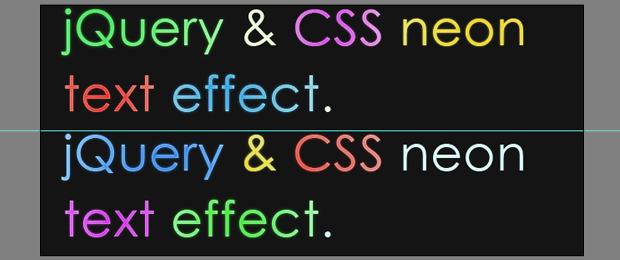
You can find the PSD file that I created for this tutorial in the download archive.
Step 2 - XHTML
The XHTML markup is really simple, you just need a container (the #neonText H1) which holds the two versions of the background.
demo.html
<h1 id="neonText"> Neon Text Effect With jQuery & CSS. <span class="textVersion" id="layer1"></span> <span class="textVersion" id="layer2"></span> </h1>
Layer1 is shown above layer2, and lowering its opacity will create a smooth neon glow effect as the background image of the span below it fades into view.
For SEO reasons, we are also providing the content of the image in plain text. It is neatly hidden from view with a negative text-indent.
Step 3 - CSS
The styles, used by the effect are also pretty straightforward. The two spans share the same background image we did in step one, but by specifying a different background position, we show only the top or the bottom part of the image.
styles.css
/* The two text layers */ #neonText span{ width:700px; height:150px; position:absolute; left:0; top:0; background:url('img/text.jpg') no-repeat left top; } span#layer1{ z-index:100; } span#layer2{ background-position:left bottom; z-index:99; } /* The h1 tag that holds the layers */ #neonText{ height:150px; margin:180px auto 0; position:relative; width:650px; text-indent:-9999px; }
The #neonText container is positioned relatively, so that the absolutely positioned spans inside it are shown exactly one on top of the other. Also notice the negative text-indent, which we are using to hide the contents of the container.
Step 4 - jQuery
The last step involves setting up the transitioning animation. As we are using the jQuery library (which we include in the page with a script tag), a couple of useful methods are made available - fadeIn and fadeOut, which we are using the code below.
script.js
$(document).ready(function(){ setInterval(function(){ // Selecting only the visible layers: var versions = $('.textVersion:visible'); if(versions.length<2){ // If only one layer is visible, show the other $('.textVersion').fadeIn(800); } else{ // Hide the upper layer versions.eq(0).fadeOut(800); } },1000); });
The function inside of the setInterval statement is executed once every second and shows or hides the first span layer.
With this our slick neon glow effect is complete!
Conclusion
You can use this effect to freshen up your designs while still keeping the SEO value of your pages. I am sure that you can think of many interesting uses and modifications of this code. Be sure to share what you do with the community in the comment section below.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Really slick. I can see this having some great potential for logo designs
Very nice :D Gona try this out right now for a new logo on my site ;)
Great stuff!
Would love to read someting about processing.js next week! :-)
My eyes ......
Great tuts but hard to integrate in website ( iThink)... Neon
I use nearly the same "technique" on http://1click.at (the 1CLICK.AT logo)
so sweet, combine plug-in and css...great
thanks
these tuts keep getting awesome and awesome. 10/10
awesome trick thank for great tutorial :)
Again, long live CSS3!!
@Scott Corgan, that is nice only this does not use a single line of CSS3.
Nice !!
Is it possible to do this with just jquery? I have read about using an animate color plugin but have not seen how to implement it yet?
Wooow! Thats really nice! Thanks!
Awesome trick! Nice!
OMG!!!So inspiration design!!
i love it...
Thanks for the great tutorial...
Im having problems selecting each word in photoshop on Mac.
I've selected the layer and selected the Rectangular Marquee Tool while holding Shift+Alt and i cant get it to hilight the text only
Any ideas? Is it different then Shift & Alt on Mac?
The sweetest i've ever seen from jquery's...
@ Ross Canpolat
Sorry, Ross, I haven't tried it on a Mac, but it should be the same key combination. Also make sure that you have the appropriate layer selected in the layers panel.
Great work! I love this bit. I'm really new to design/dev and was messing around with this effect and tried adding a twist to it but ran into some road blocks.
Has anyone combined a link with this animation? I'd love to use it to present some ideas and have a version of this animation kick off the rest of the site.
Just wonderin'
Thanks.
Pretty nice effect here! I think I'll use this in my next design!
Hey, this is great... just in case, you should warn the newbies (like) to put the
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
script...otherwise it won't work locally in a regular PC...
What code need to be change in order to make the neon text effect vertically?i try but it did not really work.Help is much appreciated.