Sponsor Flip Wall With jQuery & CSS
Designing and coding a sponsors page is part of the developer's life (at least the lucky developer's life, if it is about a personal site of theirs). It, however, follows different rules than those for the other pages of the site. You have to find a way to fit a lot of information and organize it clearly, so that the emphasis is put on your sponsors, and not on other elements of your design.
We are using PHP, CSS and jQuery with the jQuery Flip plug-in, to do just that. The resulting code can be used to showcase your sponsors, clients or portfolio projects as well.
Step 1 - XHTML
Most of the markup is generated by PHP for each of the sponsors after looping the main $sponsor array. Below you can see the code that would be generated and outputted for Google:
demo.php
<div title="Click to flip" class="sponsor"> <div class="sponsorFlip"> <img alt="More about google" src="img/sponsors/google.png"> </div> <div class="sponsorData"> <div class="sponsorDescription"> The company that redefined web search. </div> <div class="sponsorURL"> <a href="http://www.google.com/">http://www.google.com/ </a> </div> </div> </div>
The outermost .sponsor div contains two additional div elements. The first - sponsorFlip - contains the company logo. Every click on this element causes the Flip effect to be initiated, as you will see in the jQuery part of the tutorial.
Maybe more interesting is the sponsorData div. It is hidden from view with a display:none CSS rule, but is accessible to jQuery. This way we can pass the description and the URL of the sponsoring company to the front end. After the flipping animation is complete, the contents of this div is dynamically inserted into sponsorFlip.
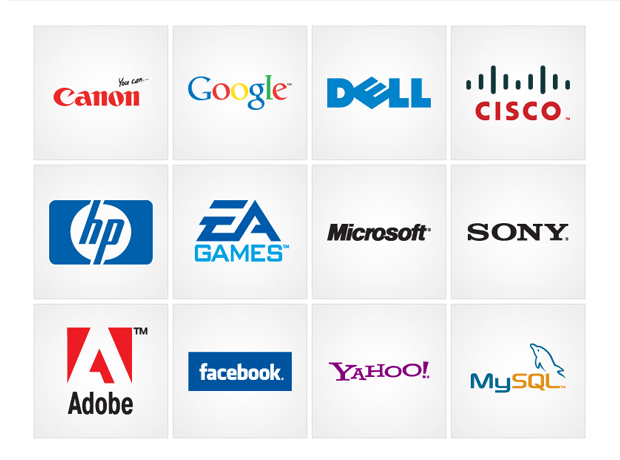
Step 2 - CSS
We can start laying down the styling of the wall, as without it there is no much use of the page. The code is divided in two parts. Some classes are omitted for clarity. You can see all the styles used by the demo in styles.css in the download archive.
styles.css - Part 1
body{ /* Setting default text color, background and a font stack */ font-size:0.825em; color:#666; background-color:#fff; font-family:Arial, Helvetica, sans-serif; } .sponsorListHolder{ margin-bottom:30px; } .sponsor{ width:180px; height:180px; float:left; margin:4px; /* Giving the sponsor div a relative positioning: */ position:relative; cursor:pointer; } .sponsorFlip{ /* The sponsor div will be positioned absolutely with respect to its parent .sponsor div and fill it in entirely */ position:absolute; left:0; top:0; width:100%; height:100%; border:1px solid #ddd; background:url("img/background.jpg") no-repeat center center #f9f9f9; } .sponsorFlip:hover{ border:1px solid #999; /* CSS3 inset shadow: */ -moz-box-shadow:0 0 30px #999 inset; -webkit-box-shadow:0 0 30px #999 inset; box-shadow:0 0 30px #999 inset; }
After styling the sponsor and sponsorFlip divs, we add a :hover state for the latter. We are using CSS3 inset box-shadow to mimic the inner shadow effect you may be familiar with from Photoshop. At the moment of writing inset shadows only work in the latest versions of Firefox, Opera and Chrome, but being primarily a visual enhancement, without it the page is still perfectly usable in all browsers.
styles.css - Part 2
.sponsorFlip img{ /* Centering the logo image in the middle of the .sponsorFlip div */ position:absolute; top:50%; left:50%; margin:-70px 0 0 -70px; } .sponsorData{ /* Hiding the .sponsorData div */ display:none; } .sponsorDescription{ font-size:11px; padding:50px 10px 20px 20px; font-style:italic; } .sponsorURL{ font-size:10px; font-weight:bold; padding-left:20px; } .clear{ /* This class clears the floats */ clear:both; }
As mentioned earlier, the sponsorData div is not meant for viewing, so it is hidden with display:none. Its purpose is to only store the data which is later extracted by jQuery and displayed at the end of the flipping animation.
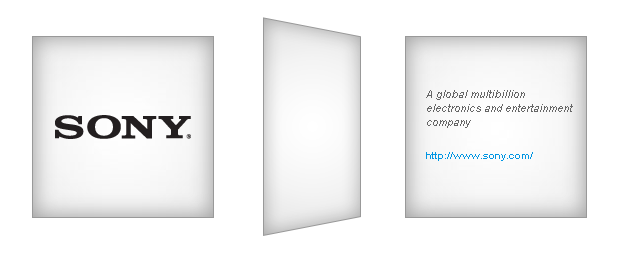
Step 3 - PHP
You have many options in storing your sponsors data - in a MySQL database, XML document or even a plain text file. These all have their benefits and we've used all of them in the previous tutorials (except XML storage, note to self).
However, the sponsor data is not something that changes often. This is why a different approach is needed. For the purposes of the task at hand, we are using a multidimensional array with all the sponsor information inside it. It is easy to update and even easier to implement:
demo.php - Part 1
// Each sponsor is an element of the $sponsors array: $sponsors = array( array('facebook','The biggest social..','http://www.facebook.com/'), array('adobe','The leading software de..','http://www.adobe.com/'), array('microsoft','One of the top software c..','http://www.microsoft.com/'), array('sony','A global multibillion electronics..','http://www.sony.com/'), array('dell','One of the biggest computer develo..','http://www.dell.com/'), array('ebay','The biggest online auction and..','http://www.ebay.com/'), array('digg','One of the most popular web 2.0..','http://www.digg.com/'), array('google','The company that redefined w..','http://www.google.com/'), array('ea','The biggest computer game manufacturer.','http://www.ea.com/'), array('mysql','The most popular open source dat..','http://www.mysql.com/'), array('hp','One of the biggest computer manufacturers.','http://www.hp.com/'), array('yahoo','The most popular network of so..','http://www.yahoo.com/'), array('cisco','The biggest networking and co..','http://www.cisco.com/'), array('vimeo','A popular video-centric social n..','http://www.vimeo.com/'), array('canon','Imaging and optical technology ma..','http://www.canon.com/') ); // Randomizing the order of sponsors: shuffle($sponsors);
The sponsors are grouped into the main $sponsors array. Each sponsor entry is organized as a separate regular array. The first element of that array is the unique key of the sponsor, which corresponds to the file name of the logo. The second element is a description of the sponsor and the last is a link to the sponsor's website.
After defining the array, we use the in-build shuffle() PHP function to randomize the order in which the sponsors are displayed.
demo.php - Part 2
// Looping through the array: foreach($sponsors as $company) { echo' <div class="sponsor" title="Click to flip"> <div class="sponsorFlip"> <img src="img/sponsors/'.$company[0].'.png" alt="More about '.$company[0].'" /> </div> <div class="sponsorData"> <div class="sponsorDescription"> '.$company[1].' </div> <div class="sponsorURL"> <a href="'.$company[2].'">'.$company[2].'</a> </div> </div> </div> '; }
The code above can be found halfway down demo.php. It basically loops through the shuffled $sponsors array and outputs the markup we discussed in step one. Notice how the different elements of the array are inserted into the template.
Step 4 - jQuery
The jQuery Flip plugin requires both the jQuery library and jQuery UI. So, after including those in the page, we can move on with writing the code that will bring our sponsor wall to life.
script.js
$(document).ready(function(){ /* The following code is executed once the DOM is loaded */ $('.sponsorFlip').bind("click",function(){ // $(this) point to the clicked .sponsorFlip element (caching it in elem for speed): var elem = $(this); // data('flipped') is a flag we set when we flip the element: if(elem.data('flipped')) { // If the element has already been flipped, use the revertFlip method // defined by the plug-in to revert to the default state automatically: elem.revertFlip(); // Unsetting the flag: elem.data('flipped',false) } else { // Using the flip method defined by the plugin: elem.flip({ direction:'lr', speed: 350, onBefore: function(){ // Insert the contents of the .sponsorData div (hidden // from view with display:none) into the clicked // .sponsorFlip div before the flipping animation starts: elem.html(elem.siblings('.sponsorData').html()); } }); // Setting the flag: elem.data('flipped',true); } }); });
First we bind a function as a listener for the click event on the .sponsorFlip divs. After a click event occurs, we check whether the flipped flag is set via the jquery data() method. This flag is set individually for each sponsorFlip div and helps us determine whether the div has already been flipped. If this is so, we use the revertFlip() method which is defined by the Flip plugin. It returns the div to its previous state.
If the flag is not present, however, we initiate a flip on the element. As mentioned earlier, the .sponsorData div, which is contained in every sponsor div, contains the description and the URL of the sponsor, and is hidden from view with CSS. Before the flipping starts, the plug-in executes the onBefore function we define in the configuration object that is passed as a parameter (line 29). In it we change the content of the sponsorFlip div to the one of sponsorData div, which replaces the logo image with information about the sponsor.
With this our sponsor flip wall is complete!
Conclusion
Today we used the jQuery Flip plug-in to build a sponsor wall for your site. You can use this example to bring interactivity to your site's pages. And as the data for the wall is read from an array, you can easily modify it to work with any kind of database or storage.
What do you think? How would you modify this code?
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Yah, that plugin is awesome. Nice effect! It's not only good for sponsors list, but also great for trusted services list using. Well done, Martin!
Great tutorial looking forward to use it, thank you, great tutorial!
Cool plugin! Thanks for sharing!
Looks great. FWIW, the hover border style tends to stick in Safari 4.0.5 when you flip a few and hover over several tiles. It could be the ole iMac pitching a fit, but may be something to check. Wish I'd thought to do this first!
Very nice plugin!
Thinking about using it for a references-page - front is a image of the project, description on the back-side...
Wow! Thanks! Great tutorial!
really nice piece of work, i like it.
Interesting, I've retwitted and digged it :)
Can you make a jQuery memory game using this?
wow! awesome tutorial. looks great.
Very nice...reminds me of the Memory game where you'd flip over two cards and try to find a match.
You might want to prevent the flipping action when a user clicks on the company URL...it kinda does this half flip, then goes to the URL. Other than that, works great!
Cool script !
Delicious'ed and RTwitted ! Thanks !
Very cool effect :-) Nicely done!
If it's posssible (which I think it is) this plugin would also be cool with just flipping when you hover over the element.
nice job) take it)
I love it!! Thanks!
Brilliant as always!
Great tutorial!
I'm thinking to use it but i've a question:
is there a way to have only one sponsor flipped at time?
Thank you
Superb plugin. I was actually looking for something like this when I got this article in my email. Thanks so much for sharing.
Thanks for the great comments, folks!
@k1c0, @jtuckr
I hadn't thought of that. It would be interesting to see it turned into a memory game.
@Goatie
It is possible, however, it would be a bit tricky, as during the flipping of the div mouseenter / mouseleave events are constantly being fired. The solution would be to lock the animation from being started in the onBegin function of the plugin, and unlock it in the onEnd (the latter is not currently used in the sponsor wall).
@Higrade
You can add the following code to the bottom of $(document).ready() to have only one sponsor flipped at a time.
This would be perfect (visually and functionality-wise), if it weren't for two major problems (for me anyhow as a developer with a special interest in web accessibility).
I know this uses a iQuery plug-in, so you'd kinda expect it requires javascript to be enabled on the user's browser, but if for whatever reason javascript isn't on, there's no fallback - if you click on the 'tiles' they just disappear off into the ether leaving a blank space. I'm not sure without trying it out on a screenreader, most if not all of which don't support javascript (and unfortauntely I don't have a screenreader handy at the moment to try it out), whether it would be accessible to a screenreader, but I'm guessing that as all the links and alt attributes are in the XHTML, a screenreader user would get at least the alt attributes and the link, though not the description from the 'flipped' tile.
The second concern is lack of accessibility for keyboard navigation users. Those who just use keyboard navigation because it's quicker for them can fairly easily revert to a mouse to click the logos, but for those who are reliant on keyboard navigation if they're unable to use a mouse or other pointing device, it's impossible to tab through the logos to click 'enter' to activate the 'flip' as a keyboard user would commonly do to access a hyperlink. The 'tiles' don't obtain focus through tabbing, so the user's sat there with a wall of icons, unable to access the additional information.
Very useful and just what I was looking for!
Great tuto...
I 'll use it for my gallery presentation.
keep in touch
awsome work man that was really helpfull.
Excelent, Very Good, Congratulation!!!
Good!!Thanks for sharing very much!!
Great effects, great job ! Thumbs up 4u !
Hi Martin!
This one was GREAT!
I have it up and running, inbedded on my site as a "We Like" page:
http://www.ekohyllan.se/press/menu_like.php
(your site of course is one of the sites "We Like") ;)
Since I´m new in the business of coding it toke me a while to get it running inside my own framework, but now its really cool!
I´m getting loads of good response from my friends who have seen it, and that of course makes my happy :)
Birgir
I don't want to make a flip just click and redirect to page. will you please tell me how to do that.
kick ass flips
Very creative! Great tut.
@ Sandeep
To make the tiles link directly to the sponsors' sites, without flipping, you should put them in a hyperlink. Just replace line 8 of demo.php - Part 2 with a hyperlink. Something like this:
After this remove the script tag which includes script.js into the page. You can also remove the jquery library, as it won't be needed.
Very nice effects awesome. Thanks. Its good also for portfolio.
@BlissC
I agree that we developers shouldn't forget about the people that prefer to have javascript disabled, in this case it isn't too difficult to have some nice effect with only CSS when javascript is disabled.
Keyboard navigation? i would say thats too much, i haven't seen many "keyboard friendly" pages (and i don't think it's necessary yet), like pausing a video in youtube with spacebar o a couple of shortcuts in google docs, but those are apps, this is just some nice effect to have for a list.
@Martin Angelov
Indeed as i have experienced, it is tricky thing to work with the mousenter / mouseleave, how about some hoverIntent? ( http://cherne.net/brian/resources/jquery.hoverIntent.html )
Thanks for sharing, cool plug in.
I am attempting to get the links on the flip modules to work with shadowbox, but I haven't be able to do so. I am trying the following:
'.$company[2].'
Thanks,
Awesome! I have a great idea for this.
Can someone tell me how to limit the columns to 3?
I will be using 9 tiles and want 3x3
Thanks! i'll check back here or brianrocks at me dot com
Nevermind... it was a Duh! moment.
just change the size of the Main div
Obviously it goes without saying that this doesn't quite work in IE6... :(
Hello!
Really nice wall, looks amazing!
Can this be integrated in Wordpress in any way?
Thank you!!
see comment below
woooow !!!
can we make it automated flip ??
Great tutorial, One question - the url when a user flips the box - It is possible to create a url e.g.
"Visit Here" instead of the actual url http://www.webpage.comm/
Thanks, Joe
Excellent idea and well crafted design. Brings life to a potentially dead page and could hold the attention slightly longer than normal.
Damn IE6, it would've been perfect... :-)
Hi and well done! this is really amazing, best i've seen in a long long time,
is it possible to have the link on the flip side as an image that would link to another part of the site ? thank very much!
This is excellent - any chance that one could embed video code in the flipped side, so when it flips, it would have the sponsor details, plus an embedded video?
I was thinking along the lines of the Oxford wall of profiles, but having the boxes flip to reveal info about the student, their course, and a playable video instead: http://www.ox.ac.uk/videowall/index.html
...something like this, but with your cool animation feature:
http://geckohub.com/jquery/youtubeplaylist/gallery.html
Article here: http://www.geckonewmedia.com/blog/2009/8/14/jquery-youtube-playlist-plugin---youtubeplaylist
Super sleek wall. Absolutely fantastic.
Just one question - In all browsers works great, however, I encountered a problem in IE7 - the browser shows an error (invalid property value on line 11) and then some of the flip images disappear after clicking on on the 5th or the 6th.
What could cause such a glitch in IE?
Thanks.
cool, thx for share
I think that this is really excellent, and am thinking of adding it into my works website.
However we have to support IE6 and as Magic Paul states above, the demo doesn't really work when viewing in IE6.
Are there any workarounds?
The best way to make it work in IE is probably detecting if the current browser is IE6 in the function that handles the click event (around line 6 in script.js).
If this is the browser in use, just redirect the page with window.location and skip the flipping all together.
Thanks Martin.
I have just started playing around with it and will have to think about IE6 a bit later!
However, I do have another question:
Is there any way that I can change the grey colour that the tiles change to when they flip?
Thanks
Hi, thanks so much for the plugin.
I am having a javascript conflict with a previous accordian javascript on my sit causing my accordian not to work. I think the conflict is with mootools.
Could i be right?
Thanks in advance.
I really like this flip wall idea. Its great for creating a good user experience.
Two questions if you don't mind and have time(?) , i'd be most grateful. Could you please show how to pull data out of an XML file, as i've been asked to pool in the data using an XML file.
Also, one idea that i thought of was that this "Wall" would be really need if it was used as a 'Dashboard' for complex web apps like ERP / CRM systems etc.
Would be really nice if there was a way to get the flipped box to expand to the full size of the canvas and either overlay or compress the other boxes out of the way. I can definitely see myself using it for that purpose even for our own in-house CRM system.
Thank you for a great tutorial nonetheless.
I like this so much and I ported the php code for asp.net using csharp. Here is the link,
http://nazmulweb.com/site4/flip.aspx
Thanks.
Hello Martin,
Neat tutorial. I find it useful and want to use it but have a few problems.
How do I embed this into a Wordpress page? I have tried a couple of times but can't figure out how to get it to work with WP. Please help.
It was a nice, helpful tutorial. Helped me a lot. But I would like to know if is it possible to flip all on a single button click say for eg FLIP ALL . In a loop ?
I wrote like this on my button click event and nothing happened
function flipall()
{
document.getElementsByClassName("sponsorFlip").style.display ='inline';
}
Kindly help
Hello!
It's very great tutor!
When i'm using it without some CMS it's work fine, but when i integrate it in my CMS (i using TextPattern), i get an error in this line:
error:
-> Warning: Invalid argument supplied for foreach() on line 5
how to fix it?
thanks in advance!
@ Becky, I am afraid that is in-build in the flip plugin. You could get an un-minified version from the plugin homepage and change it there.
@ Ziya, the error code you receive suggests that the $sponsors array is not visible to the foreach loop. You could maybe move the array immediately before the foreach. This should fix the problem.
look very nice especially since I am grappling with PHP
This is a very cool script. Thanks a great deal for sharing!
Pretty slick and amazing. I can't believe jQuery is getting further in animations this fast. Nice!
I wonder how to prevent the flip if the user clic on the link ?
Thanks
Hey guys,
I have found it doesn't work in the lastest version of Chrome I am using...6.0.427.0
Everything works, except the sponsor logo's aren't displayed and therefore it's just a blank gray square.
Can this be looked at??
Nick
great tutorial and nice effects.
I found it really good, would like to try it on one of my clients' portfolio site.
Thanks for sharing this us.
Regards
Xcellence IT
can someone help me please and tell me what I am doing wrong as the flipping thing isn't happening anymore.
It appears that revertFlip does not take any parameters like flip() does? It would be nice if it did:
elem.revertFlip({
direction:'rl',
speed: 350,
onBefore: function(){},
onEnd: function(){
//elem.find('.stickyTextArea').hide();
$(sender).siblings('.stickyNoteInfo').hide();
Really Great job and just imaging but not proper working in ie .
Thanks
Found your Post on Drupalcenter.de ... This is a cool Effect! Maybe i will use it in future!
THX for it!
Awesome Share....!! Thanks..!!
Hi, its great :)
Im trying in my website
(http://soutez.rubstudio.cz/index.php?option=com_content&view=article&id=58&Itemid=90)
in chrome working, but in Safari 5.0 dont seeing logos :(
Whats wrong?
Its great, but sometimes a little bit buggy in the Internet Explorer 6, 7 and 8...
Hello and thanks for your job ! Sorry,my english is very bad and i hope you will understand my problem : IE7/8. The flip doesn't work correctly and disapear on the left border. I use the script on my Drupal website and i thinks the problem is with jquery ui. It's possible ?
Thanks.
I have modified using asp.net. Please check the link http://blog.rlambda.com/2010/07/29/client-flip-wall-using-asp-net/.
Please comment if its is interesting for you or the one you are looking for.
hey guys, i need help!
i need to 'shuffle' all the items but output only 3 of them, is that possible?
thanks-)
nice plugin
Thanks for sharing!
WOW awesome plugin!!! Thanks for the tut!
This is a great tutorial! I am having problems seeing the logos on firefox and chrome though, the box is just the gray image, but the flip works. It does work on Safari and IE on the same computer though; any idea what the problem could be?
No answers? Anyone? Really trying to get this to work.
Doesn't work in firefox :-(
I tested under firefox and work perfectly !
Im fan of this website! thanks for all greats tuttos
Not sure if your still having this issue but, AdBlock Plus was preventing it from showing in Firefox, for me. Once I disabled it for this site, it worked fine.
Late, but for anybody else having this issue.
Just rename "Sponsor" to "Client" or something else, some adblocker software looks for the word Sponsor in pages.
I have Firefox & it works like a charm. There's nothing wrong with it.
Hey - I'm normally a huge advocate for Flash but this is sexy! How can I make the flip-side animate over the other tiles? I tried flipping .sponsorListHolder instead of .sponsorFlip but as it reached the end of the turn it becomes invisible. Any advice would be awesome.
Thanks again - long live open source
This is a great tutorial! It works flawlessly.
Very Cool. I use it.
Martin Angelov
Thanks!
@Higrade
You can add the following code to the bottom of $(document).ready() to have only one sponsor flipped at a time.
$(".sponsorFlip").bind("click",function(e,block){
});
This is a very nice effect, and I immediately loved it!
I created an extension for this for Yii users, which I tagged as
mflip
After hours of trial and error I found hoverIntent and used it so far seems to work just fine
http://cherne.net/brian/resources/jquery.hoverIntent.html
$(document).ready(function(){
$(".sponsorFlip").hoverIntent(function() {
var elem = $(this);
elem.flip({
"direction": "lr",
"speed": 350,
"onBefore": function() {
elem.html(elem.siblings(".sponsorData").html());
}
});
}, function() {
$(this).revertFlip();
}
)});
I'm trying to enable the flipping only when a text is clicked, instead clicking the whole panel.
P.S. I changed the term 'sponsor' to 'panel'.
I also moved the panelURL inside the panelFlip, instead of being inside the panelDescription.
Here's what I did:
$('.panelURL').bind("click",function(){
var elem = $(this);
Obviously, only the text/URL will flip because the 'this' now refers to the text/URL.
I tried
$('.panelURL').bind("click",function(){
var elem = $('.panelFlip');
But this leads to all of them flipping when the text is clicked, because they are all under the panelFlip class. Any suggestions?
Fist of all, great tutorial!
I've been trying to combine this flip wall with Quicksand (http://razorjack.net/quicksand/docs-and-demos.html) using the AJAX call method in the demos/examples.
Everything worked fine except that, once i call a filter option, suppose i display only 3 of the sponsors, the flip function doesn't work anymore. Any approach on this?
I'm a newbie on jQuery, so I'm learning using this as references.
Thanks!
solved, I just needed to use live instead of bind. I'm now wondering if there's any way to keep the flipped sponsor if filtered... so, if i flip i.e. dell sponsor, and then filter the results, if dell sponsor is included on those results, it should remain flipped, not reset to it's original state, so it'll be graphically coherent. Can this be done using an array and condidionals?
Hi , I'm trying to use this for a package browser so i have it set up to only allow one sponsor to be turned at one time , However i am finding that if someone clicks several sponsors in a short amount of time that it allows more than one sponspor to be viewed at the one time , apart from changing the time inwhich it takes to turn how could it be done so that only one may be turned ay a given time and should you user click lots in a short space of time it will not break the script
Many Regards Ross
@Becky Commenting on an old thread but thought this might help those who want to get rid of the grey background once the card is flipped.
add this to your flip call:
dontChangeColor: true
Only works after version 0.9.9
Martin,
Thanks for sharing the knowledge. I have a similar requirement could you please throw some light.
I wish to have a image wall (in fact, each image is a front cover of the book - book object)
For every equal interval, one of the book (random) should be flipped automatically, so that user can see a different book.
If i hover mouse, i should get a popup with the details of the book say(price, availability, author etc)
I wish to have this in asp.net, jqueryui
Great effect, but the script JQuery stopped working yesterday 2011/12/28.
Any solution?
Thanks.
It was a mistake of mine, the domain from which JQuery load is blocked on my computer.
Great work dude ....!!! Really amazing :)
Great plugin Martin, many thanks!
Btw, I'm using this sponsorFlip Wall for the references on my webpage.
Because of that, I need to have some appropriate picture for every reference.
So, I want to use prettyPhoto (image plugin) for viewing thoose pictures...
...and, at the bottom:
$("a[rel^='gallery']").prettyPhoto();
prettyPhoto doesn't get fired this way, but the picture is being loaded in a new tab!
prettyPhoto works fine in outside .sponsorFlip class, so I think there is some conflict with .sponsorFlip click() event and prettyPhoto click() event ???
Does anyone know what this could be ?
Thanks !!!
Hi,
I am using your Fantastic!!!!
I was playing around wih it and ran into touble today. I redownloaded the demo to see if it was me and loaded it into ie and firefox. Firefox is fine but if i click randomly away on the boxes eventually i get a yellow warning triangle at the bottom left of ie and one of the boxes disapears. If i click another that disapears to. When i click on the yellow triangle at the bottom left of ie to get the error it says:
Invalid Property value: jquery-ui.min.js : Line10 : Code 0 : Char 81832
http://ajax.googleapis.com/ajax/libs/jqueryui/1.7.2/jquery-ui.min.js
Any idea if this is fixable as it will probably break anyones wall who is using a link to the jquery-ui.min.js file
I look forward to your reply
Regards
Kevin
Most likely a syntax error
I was looking around for a tutorial to mimic Metro UI and this came close. These are some modifications that I made to this 'neat-plugin'.
1 - I used a [$('.sponsorFlip').bind("dblclick",function()] double click command instead of a click, because I am adding a form on the sponsorDescription flip side. this enables users to click within a form element without the flip occuring.
2 - I changed the dimensions (h/w) using CSS for each 'sponsor' using a secondary class name like [class="login sponsor"] then [class="profile sponsor"], this gives me different size boxes on the cover page. Duplicating Microsoft's Metro UI style.
My Road-blocks:
1 - when trying to insert a php echo command within the 'sponsorDescription' class, nothing is written to the page (blank, html works fine though).
2 - How to I center the flip animation when I increase the width/height in the css from 100% to 500%. (example: I am trying to have the one side 150px by 150px but when flipped, 500px by 450px. centered.)
My comments on your tutorial - this is a very cool add-on, and very customizable. Much Thanks!
Anyone able to create a link outside of all this that can flip a specific DIV? this would be similar to the question by @Fontia
If anyone is still looking to add this to Wordpress, we made a plugin:
http://www.atlanticbt.com/blog/wordpress-plugin-sponsor-flipwall-shortcode/
http://wordpress.org/extend/plugins/sponsor-flipwall-shortcode/
Not cross browser compatible. Should've work in IE at least.
hi,nice job!
How can i set the effect from this http://tutorialzine.com/2010/04/simple-banner-rotator-with-php-jquery-mysql/ , to what you have done here? It is possible? i mean it possible, but how can i do?
I do not want to use that version, because I do not want to use a database for the sponsor wall. TNX
hello again .... I have a site made in joomla, the Java code used by the sponsor wall, come in conflict with jquery in joomla. What can I do?
This works great except for ie9. It flips but on clicking URL it flips back but never opens new window/tab. Has anyone figured a workaround?
Thanks
I am creating a web application with this plugin and I need it to flip back to the front side when an external button is clicked. I would also like to hide the animation on rotation back. Any ideas how I can accomplish that?
Finally manage to fix sponsor-flip-wall to work with PrettyPhoto !!!
Firstly, place the rel attributes for <a href>:
<a href="'.$company[2].'" rel=”gallery” title=”‘.$company[3].’”>Gallery</a>
$("a[rel^='gallery']").prettyPhoto();
That is it . . . this way prettyPhoto will be called without problems !!!
:: cheers ::
Hi i want to implement this for wordpress where my all sponsor images are locates in image folder.
i have changed
<img src="img/sponsors/’.$company[0].’.png" alt="More about ‘.$company[0].’" /> lines in demo.php
to
<img src="<?php bloginfo(‘template_url’);?>/images/’.$company[0].’.png" alt="More about ‘.$company[0].’" />
which through error like this :
Parse error: parse error, expecting
’,” or
’;” in C:\xampp\htdocs\wordpress\wp-content\themes\viv\includes\demo.php on line 49please help me
@Martin Angelov - Any chance the IE flip issue was fixed? I was able to get this working on my site just fine in Firefox, but then when I look at it in IE the div flips and when you try to click the url/button it flips back without ever clicking the link. Its the same issue Bob is having above.
@cesalio45g - Can you please tell me a little bit more about using the [$('.sponsorFlip').bind("dblclick",function()] in the script.js file? It sounds like that could fix the issue if it works in IE too?
Hi i want to implement this for wordpress where my all sponsor images are locates in image folder.
i have changed
<img src="img/sponsors/'.$company[0].'.png" alt="More about '.$company[0].'" /> lines in demo.php
to
<img src="<?php bloginfo('template_url');?>/images/'.$company[0].'.png" alt="More about '.$company[0].'" />
which through error like this :
Parse error: parse error, expecting
','' or
';'' in C:\xampp\htdocs\wordpress\wp-content\themes\viv\includes\demo.php on line 49please help me
I had the same issue... try this
<img src="'.get_bloginfo(template_url).'/Images/'.$company[0].'.png" alt="More about '.$company[0].'" />
how do i make images constant when refreshing the page or when opening the page, but not stopping stopping fliping
i have download this flip box, its great tutorial, please could any one know how to change the color of background after the box flip. it shows as a inline color r g b and in element it shows #99999. i would like to change that color to white but i cant the way out, i have looked everywhere but still struggling.... please advise
Many Thanks
You simply just add "color:'#fff'" in the function options
.flip({
direction:'lr',
speed: 350,
color: '#fff', <---- This will change the background color for you
});
Hi i'm trying to show the sponsors in alphabetich order how i can do that instead of using the shuffle <?php shuffle($sponsors);?> ?
sort the array?!?
This is really beautiful! However, I want to use it for our company website, but our servers don't run PHP. Is there any way I can write it all out in HTML and add the data myself in the doc without the arrays? (might be a stupid question... sorry)
I'm not all that spiffy at scripting, but I tried using the HTML that the PHP would generate, and while it somewhat works, it seems all elements in each pane are accompanied by a number ranging from 01-13. I can't figure out where they come from.
Main question though - anyway of making this work without PHP?
Hi Stina,
have you ever managed to make this work with HTML only?
Hi...We are using this effect here: (http://musikmedia.net)
I was wondering if anyone knows a simple way to tie all the flip boxes to one button or link. One link to flip all the boxes, or one of the boxes. Basically a link or button on the same page that does something like...
<a href="script.php?function=dosomething"> ???
Thanks!
Nice tutorial & plugin. I have tried to implement a fallback for users who disabled javascript, my solution seems to work fine in most browsers but I think there must be a better way to solve this. You can check it here at http://adeptios.com
Also wondering if there are any newer JQuery scripts for flipping, the one this is based on from Luca Manno (http://lab.smashup.it/flip) dates back to 2009 if I'm correct.
Would be great to see a 2nd part to this where we put in 'favorite sites' and the script takes a screenshot of those sites and uses them as pictures to flip.
How do I change Sponsor's Website link on the reverse flip to a simple CLICK HERE hyperlink on each flip side? Want to use the wall as menu for a photogallery.
Hi,
In firefox does not display a single sponsor
hi,
great stuff.
I included a second URL for further information about this sponsor.
And i'm trying to revertFlip after a special time rather than clicking...
and trying to set the flip-direction to random.
This feature looks so awesome and I would really like to be able to implement it. Would anyone be so kind as to tell me how I can implement it into my Drupal site that I will be developing?
I used this to make a fun little memory and guessing game.
How did you do it?? Could you please help me??
Window open in new window:
<div class="sponsorURL">
<a href="'.$company[2].'" target="_blank">'.$company[2].'</a>
</div>
Hope this helps.
Thanks , this works for me!
Great work Martin...
How can I make the image bigger? About the size of a business card? and just show in a slide like way.
Thx for any help.
Bummer. It doesn't work in Firefox (currently using 16)
@Steve:
it does work on everything incl. IE7 - you must have messed up implementation
@everyone:
if you need to make square flip on clicking on a button (e.g.), not entire surface use this launcher (replacement for script.js from demo folder):
Hello,
this tutorial is really great. I have a problem with the shadows. My cards should have a shadow, that rotates with the card. Have anyone a solution for me?
Greetings from Germany
(My English is not good, sorry!)
Hey!
Great work Martin!
How can I make that i only have 3*3 Sponsor Images in the center of my page?
Have anyone a solution?
Thx for any help.
And sry 4 my bad english
Joshua
for flipping on mouseover use this code:
Hey Martin, this is very nice! I will download and play with it, but I need two more features. Please tell me if the script can do these things:
1) flip a single panel through several images (using hover)
2) once an image is settled upon, click to save it's id in a form's textbox
Basically, I want to use this as a basis for filling out a form. Can it be done?
Tx,
George
Hi Martin
I really liked your plugin, I added it and works super well but generates conflict with a plugin dropdown menu, the menu stays open all the time. I wonder what is the root of the problem, if you could help me, because before activating your plugin it looked so good.
Thank you!
I would guess you've loaded jquery multiple times. Usually the cause of this.
Is it possible to prevent the flip when you click on the actual text link that brings you to another page? Let me know if that's possible.
Thanks
This is how I got the hover in/out to work:
Hi,
thanks for the hover solution!
Hover if you hover over all elements quickly, some stay flipped. Do you know if there is a solution for this?
Thanks,
Danyo
Thanks a million... Brilliant plugin, and nicely explained! :-D
Our sponsors need to be displayed in categories - is there a way to create groups separated by category headline?
Is it possible to leave a tile flipped when you go onto the page it goes to?
How about an update to the code.....it's been quite a long time and the multiple conflicts I have with other code is making this plug-in obsolete.....too bad because no one else has done this YET. Thanks
Hi,
First of all. Great tutorial. Thanks.
I have implemented your version. Instead of using a div with CSS, I am using an image which is flipped to a background with text on it. Is it possible to take the image over within the flipping effect? So that the background-color when flipping is still the image until completely flipped?
I would highly appreciate some help, since I am very new to this.
Thanks,
Lisa