Animated Sharing Bar With jQuery & CSS
Social networks can make a big difference on the popularity of a blog. Sites that communicate better and understand social media are usually the most popular.
A move towards this goal would be to find a way to encourage your visitors to share your content on the networks they are most active on.
Today we are doing just that, by using pure JavaScript with the jQuery framework, to make an animated sharing bar, which will enable your website visitors to share posts on a number of social networks.
Go ahead and download the demo files and continue with step one of this tut.
Step 1 - XHTML
As usual, we start off with the XHTML markup. The sharing area is constructed by using three main div containers:
- #share - this element acts as a container which holds the other two. It also has some CSS3 properties applied, such as rounded corners;
- #stage - this is where the animation takes place. On page load jQuery detects the horizontal and vertical centers and uses them to position the rotating divs. It is also floated to the left;
- #share-label - this has the "share the love" image as a background. It is also floated to the left and thus positioned right next to the #stage div.
But lets have a detailed look:
demo.html
<div id="share"> <div id="stage"> <div class="btn digg"><div class="bcontent"><a class="DiggThisButton"></a><script src="http://digg.com/tools/diggthis.js" type="text/javascript"></script></div></div> <div class="btn tweetmeme"><div class="bcontent"><script type="text/javascript" src="http://tweetmeme.com/i/scripts/button.js"></script></div></div> <div class="btn dzone"><div class="bcontent"><script language="javascript" src="http://widgets.dzone.com/links/widgets/zoneit.js"></script></div></div> <div class="btn reddit"><div class="bcontent"><script type="text/javascript" src="http://www.reddit.com/button.js?t=2"></script></div></div> <div class="btn facebook"><div class="bcontent"><a name="fb_share" type="box_count" href="http://www.facebook.com/sharer.php">Share</a><script src="http://static.ak.fbcdn.net/connect.php/js/FB.Share" type="text/javascript"></script></div></div> </div> <div id="share-label"> <!-- This is where the share some love image appears --> </div> </div>
The code above is all you need to add to your page (in addition to the js and css files), so you can show the animated bar below your articles.
Each button has a generic btn class and an individual one (digg, tweetmeme, etc). This makes it possible to gather the common rules shared by the buttons in a single class and make it easier to customize at a later time.
Also notice that inside of each of the button divs, there is an individual JavaScript file that actually generates the button on the page. Those are provided by the social networks and usually display the button exactly where the script tag is inserted.
Now lets see how these elements are styled.
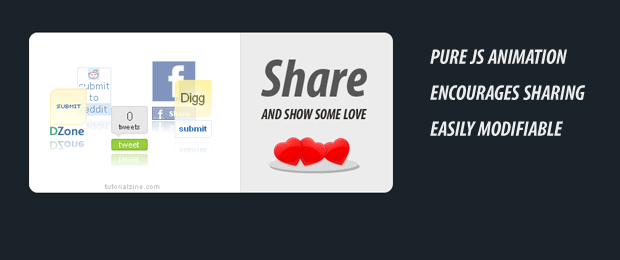
Step 2 - CSS
I've divided the CSS code into two parts.
demo.css - Part 1
#share{ /* The share box container */ width:500px; background:#ececec; height:220px; margin:60px auto; overflow:hidden; -moz-border-radius:12px; -webkit-border-radius:12px; border-radius:12px; } #share-label{ /* The image on the right */ background:url(img/share.png) no-repeat 50% 50%; float:left; height:220px; width:200px; } #stage{ /* This is where the animation takes place */ position:relative; border-right:1px solid #DDDDDD; width:290px; height:220px; background:white; float:left; border-bottom-left-radius:12px; border-top-left-radius:12px; -moz-border-radius-bottomleft:12px; -moz-border-radius-topleft:12px; -webkit-border-bottom-left-radius:12px; -webkit-border-top-left-radius:12px; } .btn{ /* This class is assigned to every share button */ background-color:white; height:90px; left:0; top:0; width:60px; position:relative; margin:20px 0 0 10px; float:left; } .bcontent{ /* Positioned inside the .btn container */ position:absolute; top:auto; bottom:20px; left:0; }
Maybe at this stage you are wondering how the actual buttons are animated in a circular movement on the page.
A major part of the technique takes place inside the #stage CSS class above. The stage itself is relatively positioned, which enables the buttons (which have absolute positioning assigned by jQuery below, and are direct descendants) to be positioned accordingly.
This means that setting top and left to zero on the buttons, positions them in the top-left corner of the stage.
You'll see the rest of the technique in step 3.
demo.css - Part 2
/* Individual rules for every share button */ .digg{ background:url(img/digg_reflection.png) no-repeat -4px bottom;} .reddit{ background:url(img/reddit_reflection.png) no-repeat -4px bottom;} .facebook{ background:url(img/facebook_reflection.png) no-repeat bottom center;} .tweetmeme{ background:url(img/twit_reflection.png) no-repeat -5px bottom;} .dzone{ background:url(img/dzone_reflection.png) no-repeat -7px bottom;} .thanksto{ position:absolute; bottom:2px; right:4px; font-size:10px; } .thanksto a,.thanksto a:visited{ color:#BBB; } /* Customizing the facebook share button */ span.fb_share_no_count { display:block; } span.fb_share_count_top.fb_share_no_count { line-height:54px; } span.fb_share_count_nub_top.fb_share_no_count{ display:none; } span.fb_share_no_count span.fb_share_count_inner { background:#3B5998 url(http://static.fbshare.me/f_only.png) no-repeat scroll 20px 5px; display:block; }
In the second part of the style sheet, we assign individual rules to each button which define a unique background image with the reflection. After this we customize the facebook button, so that its styling matches the rest of the buttons.
With this we can continue with step 3.
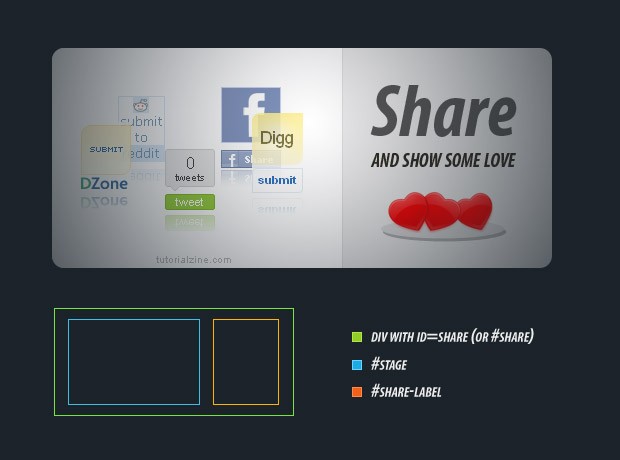
Step 3 - jQuery
With the speed with which modern browsers render JavaScript, it gets easier to make eye-catching animations, previously reserved only for technologies as Adobe Flash.
But before getting down to work, we need to include two script files in the head of our page:
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js"></script> <script type="text/javascript" src="script.js"></script>
The first one includes the jQuery library from Google's CDN, and the second line includes our own script.js file, covered in detail below:
script.js
$(document).ready(function(){ /* This code is run on page load */ var deg=0; var dif=-3; /* Assigning the buttons to a variable for speed: */ var arr = $('.btn'); /* Caching the length of the array in a vаriable: */ var len = arr.length; /* Finding the centers of the animation container: */ var centerX = $('#stage').width()/2 - 40; var centerY = $('#stage').height()/2 - 60; /* Applying relative positioning to the buttons: */ arr.css('position','absolute'); /* The function inside the interval is run 25 times per second */ setInterval(function(){ /* This forms an area with no activity on mouse move in the middle of the stage */ if(Math.abs(dif)<0.5) return false; /* Increment the angle: */ deg+=dif; /* Loop through all the buttons: */ $.each(arr,function(i){ /* Calculate the sine and cosine with the new angle */ var eSin = Math.sin(((360/len)*i+deg)*Math.PI/180); var eCos = Math.cos(((360/len)*i+deg)*Math.PI/180); /* Setting the css properties */ $(this).css({ top:centerY+25*eSin, left:centerX+90*eCos, opacity:0.8+eSin*0.2, zIndex:Math.round(80+eSin*20) }); }) },40); /* Detecting the movements of the mouse and speeding up or reversing the rotation accordingly: */ var over=false; $("#stage").mousemove(function(e){ if(!this.leftOffset) { /* This if section is only run the first time the function is executed. */ this.leftOffset = $(this).offset().left; this.width = $(this).width(); } /* If the mouse is over a button, set dif to 0, which halts the animation */ if(over) dif=0; else dif = -5+(10*((e.pageX-this.leftOffset)/this.width)); /* In the other case calculate the speed according to the X position of the mouse */ }); /* Detecting whether the mouse is positioned above a share button: */ $(".bcontent").hover( function(){over=true;dif=0;}, function(){over=false;} ); });
The main idea here, is that we use setInterval to set up a function to be run every 40 milliseconds. This means that it is run 25 times a second, or if you compare it with a movie screen, this is 25 frames per second. In other words more than enough to display a smooth animation (providing that the rendering speed of the browser is fast enough, and that there are no other scripts interfering).
As this is not a computation-heavy animation, it runs pretty smoothly on all browser versions (even as old as IE6). However, the smoothest animation I've observed is in Safari and Chrome (it would run nicely in Firefox too, providing that you don't have a lot of add-ons or opened tabs).
You can see throughout the code above, that I tend to assign the results of jQuery selectors or other functions to variables. This is done to improve the speed of the script, otherwise all those functions and methods would be calculated on every frame (for a total of 25 times per second) which would ultimately bring down the performance and smoothness of the animation.
With this our animated sharing bar is complete!
Conclusion
In this tutorial, we created an animated social sharing bar which encourages visitors to share articles on a number of social networks with an eye-catching animation.
To include new services, in addition to the five currently active, you just have to include a new btn element in the stage div with an appropriate script that would generate the button.
What do you think? How would you improve this script?
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Take that flash, we have no use for you anymore.
This is really awesome. Almighty jQuery! Thanks for sharing this great tutorial.
Really cool tutorial. I personally wouldn't use this for my site, but it just shows us that the possibilities are limitless with jquery. Thanks for pushing the limit and coming up with one incredible tutorial after another.
You've been busy.
Keep up the good work sir.
thanks goood
I'm speechless :)
You are my new hero :D
Just keep them comming
That's really nice. Just wanted to drop by and say thanks - I really enjoy your tutorials. =] Keep up the good work.
Amazing, and as said, the beginning of the end for flash on websites, lol.
Not sure how practical or how much people would use it, but its great to see something like this in use, and it could be adapted for things like banners etc.
Very nice, but why XHMTL? This can be done just with HTML (3.2, 4.01, 5). I do not see any reasons to use XHTML (with this and other scripts, and… at all). BTW: so why "demo.html" and not "demo.xht"?
Great work, thanks for sharing :)
Thank you for this great Tut!
I think a non-animated sharing bar is much more user friendly: it doesn't distract so much and it goes quicker to ckick on a static icon. Users should easily find the bar, but it shouldn't disturb the reading process.
Anyway the jQuery and tutorial are very interesting and a good job has been done on it - thanks for it. But, as said Design Informer, this animated sharing bar shouldn't be used. It's a pitty to work hard for little use; perhaps the same code used for another feature would have more future :-)
Thanks for the great comments folks! Really appreciate it!
@ TomkOx
XHTML is the standard for contemporary web development, and as this is a tutorial, it is best that the readers are presented with it.
However, you are free to modify the demo and rewrite it in any spec you'd like.
@ Regine Lambrecht
The aim of the tutorial is to teach people and show them what is possible to be done with pure JS alone. The example that is build is a proof of concept that complements the tut, rather than a standalone script.
So as long as the sharing bar draws interest to the matter of JS animations, it is a job done.
thank for share. very good
Excellent detailed tutorial. Thanks for sharing
Nice post... thanks for sharing.
-Deepu
Another great tutorial! This site is way better than nettuts
I was using this from flash...........but now i have no need of flash.........
Thank's a lotttttttttttttttttttttttttttttttttt sir.
Cool! another great tutorial, thanks
Great tutorial! thanks for sharing
hei, it's realy cool.. great job froend...
Not as user friendly as a static version, but still a great effort in demonstrating another reason for not needing Flash.
Keep up the good work.
nice work :D
That was pretty creative, thanks
Fantas-Tik
Too bad you need so much code to do this little thing. In flash it takes a few lines of code to tdo the same and it runs much more smoothly :) with that amount of code, I could add a motion blur effect, 3D, and multi-touch
Hello Martin,
this is similar to one of my image shows, check out if you want: http://uglydesign.freehostia.com/software/wheelgal/wheelgal.php
However these experiments taught me what "oldish CPU" means
:-)
cheers
ivan
amazin work...keep it up...thx alot
very nice concept..
this is a very clever way of a Animated sharing bar with Jquery. I will try and implement this
Excellent post. How can i increase the radius of the circle. I want the items to have more distance between them.