A Fancy AJAX Contact Form
Introduction
Providing a simple and reliable means of feedback from site visitors is a crucial part of any web presence. The most simple and common feedback channel are contact forms.
In this tutorial we are going to make an AJAX contact form which leverages modern web development techniques.
We are using PHP, CSS and jQuery with the help of the formValidator plugin for form validation and the JQTransform plugin, which will style all the input fields and buttons of the form. In addition we are using the PHPMailer class to send out the contact form emails.
For the page background we are using a fantastic dark wood texture by Matt Hamm.
The form degrades gracefully, which means it is perfectly usable even with JavaScript turned off.
*Edit: Also make sure you are running PHP 5. If this is not the case, you can switch the PHP version from your hosting control panel.
So lets start with the tutorial.
Step 1 - XHTML
First, we are going to take a look at the XHTML markup behind the form.
demo.php
<div id="main-container"> <!-- The main container element --> <div id="form-container"> <!-- The form container --> <h1>Fancy Contact Form</h1> <!-- Headings --> <h2>Drop us a line and we will get back to you</h2> <form id="contact-form" name="contact-form" method="post" action="submit.php"> <!-- The form, sent to submit.php --> <table width="100%" border="0" cellspacing="0" cellpadding="5"> <tr> <td width="18%"><label for="name">Name</label></td> <!-- Text label for the input field --> <td width="45%"><input type="text" class="validate[required,custom[onlyLetter]]" name="name" id="name" value="<?=$_SESSION['post']['name']?>" /></td> <!-- We are using session to prevent losing data between page redirects --> <td width="37%" id="errOffset"> </td> </tr> <tr> <td><label for="email">Email</label></td> <td><input type="text" class="validate[required,custom[email]]" name="email" id="email" value="<?=$_SESSION['post']['email']?>" /></td> <td> </td> </tr> <tr> <td><label for="subject">Subject</label></td> <!-- This select is being replaced entirely by the jqtransorm plugin --> <td><select name="subject" id="subject"> <option value="" selected="selected"> - Choose -</option> <option value="Question">Question</option> <option value="Business proposal">Business proposal</option> <option value="Advertisement">Advertising</option> <option value="Complaint">Complaint</option> </select> </td> <td> </td> </tr> <tr> <td valign="top"><label for="message">Message</label></td> <td><textarea name="message" id="message" class="validate[required]" cols="35" rows="5"><?=$_SESSION['post']['message']?></textarea></td> <td valign="top"> </td> </tr> <tr> <td><label for="captcha"><?=$_SESSION['n1']?> + <?=$_SESSION['n2']?> =</label></td> <!-- A simple captcha math problem --> <td><input type="text" class="validate[required,custom[onlyNumber]]" name="captcha" id="captcha" /></td> <td valign="top"> </td> </tr> <tr> <td valign="top"> </td> <!-- These input buttons are being replaced with button elements --> <td colspan="2"><input type="submit" name="button" id="button" value="Submit" /> <input type="reset" name="button2" id="button2" value="Reset" /> <?=$str?> <!-- $str contains the error string if the form is used with JS disabled --> <img id="loading" src="img/ajax-load.gif" width="16" height="16" alt="loading" /> <!-- the rotating gif animation, hidden by default --> </td></tr> </table> </form> <?=$success?> <!-- The $success variable contains the message that is shown if JS is disabled and the form is submitted successfully --> </div> </div> <!-- closing the containers -->
As you can see from line 8, we are submitting our form to submit.php. We are using this file to handle both the regular form submit (for visitors with JS disabled) and the AJAX form submit. This allows the code to be updated easily with no need to merge changes between files.
Later you can see that we use the $_SESSION array to populate the values of the input fields. This is done to insure that the data is not lost during page redirects, which occur when the form is submitted to submit.php during the regular form submit.
Another important aspect are the classes assigned to the input fields - classs="validate[required,custom[onlyLetter]]". These classes are used by the validation plug-in to define the validation rules for every input field, or text area. We are basically saying that the field is required, and only letters are allowed.
There is a number of available validation rules. You can see them at the plug-in's homepage.
Now lets see how the plain form is fancyfied with the use of the JQtransform plugin.
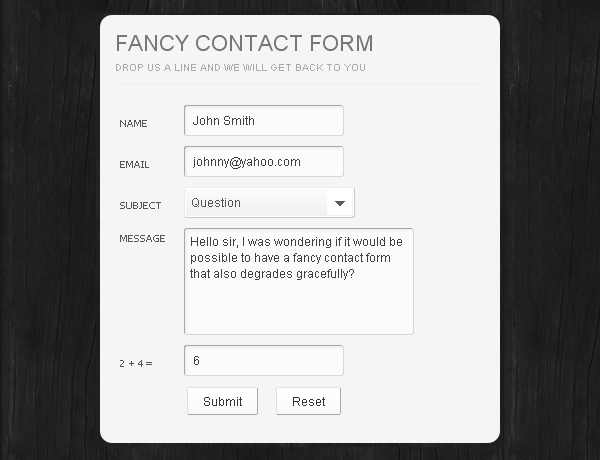
Step 2 - jQuery
We are using two jQuery plugins - JQtransform for styling all the form elements, and formValidator, which will help us validate all the input fields on the client side.
It is important to remember to always validate the input data on the server side in addition to client side validation.
First we need to include all the required libraries.
demo.php
<link rel="stylesheet" type="text/css" href="jqtransformplugin/jqtransform.css" /> <link rel="stylesheet" type="text/css" href="formValidator/validationEngine.jquery.css" /> <link rel="stylesheet" type="text/css" href="demo.css" /> <?=$css?> <!-- Special CSS rules, created by PHP --> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js"></script> <script type="text/javascript" src="jqtransformplugin/jquery.jqtransform.js"></script> <script type="text/javascript" src="formValidator/jquery.validationEngine.js"></script> <script type="text/javascript" src="script.js"></script>
The above code is from the head section of demo.php. We first include the stylesheets that are used by the two plugins, and then the jQuery library and the plugins. You might find line 5 interesting - this is a special set of CSS rules that we create with PHP to show a confirmation message, as you will see later.
Now lets take a look at our script.js.
script.js
$(document).ready(function(){ /* after the page has finished loading */ $('#contact-form').jqTransform(); /* transform the form using the jqtransform plugin */ $("button").click(function(){ $(".formError").hide(); /* hide all the error tooltips */ }); var use_ajax=true; $.validationEngine.settings={}; /* initialize the settings object for the formValidation plugin */ $("#contact-form").validationEngine({ /* create the form validation */ inlineValidation: false, promptPosition: "centerRight", success : function(){use_ajax=true}, /* if everything is OK enable AJAX */ failure : function(){use_ajax=false} /* in case of validation failure disable AJAX */ }) $("#contact-form").submit(function(e){ if(!$('#subject').val().length) { $.validationEngine.buildPrompt(".jqTransformSelectWrapper","* This field is required","error") /* a custom validation tooltip, using the buildPrompt method */ return false; } if(use_ajax) { $('#loading').css('visibility','visible'); /* show the rotating gif */ $.post('submit.php',$(this).serialize()+'&ajax=1', /* using jQuery's post method to send data */ function(data){ if(parseInt(data)==-1) $.validationEngine.buildPrompt("#captcha","* Wrong verification number!","error"); /* if there is an error, build a custom error tooltip for the captcha */ else { $("#contact-form").hide('slow').after('<h1>Thank you!</h1>'); /* show the confirmation message */ } $('#loading').css('visibility','hidden'); /* hide the rotating gif */ }); } e.preventDefault(); /* stop the default form submit */ }) });
This entire script block is executed within the $(document).ready method, which guarantees that it is run after the page has finished loading.
Next we use the jqTransform() method that is defined by the jqtransform plugin. It converts and styles all of the form's elements (input fields, text areas, buttons).
The select element is actually replaced by a set of divs and anchors. It surely looks great, but opens up some problems with the validation plugin which make us handle our own tooltip for the select drop-down.
After this on line 7 we bind every click on the buttons at the bottom of the page with a line of code that hides all currently displayed error tooltips by the validation plugin. This insures they are properly refreshed and do not stay on screen if the user enters valid data.
Later we initialize the formValidation plugin with the validationEngine() method and on line 24 define the form's onsubmit event. A few things worth mentioning here - the custom tooltip on line 28, and the additional ajax=1 parameter on line 39. This parameter is used by submit.php to distinguish whether the request has been made through ajax or directly with the form submit.
Also notice that we use a special variable use_ajax, to prevent ajax interactions if the form fails to validate.
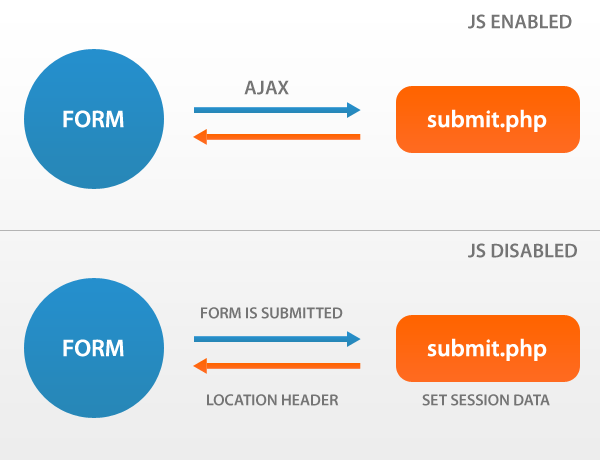
Step 3 - CSS
All of our CSS rules are defined in demo.css
demo.css
body,h1,h2,h3,p,quote,small,form,input,ul,li,ol,label{ /* reset some of the page elements */ margin:0px; padding:0px; } body{ color:#555555; font-size:13px; background: url(img/dark_wood_texture.jpg) #282828; font-family:Arial, Helvetica, sans-serif; } .clear{ clear:both; } #main-container{ width:400px; margin:30px auto; } #form-container{ background-color:#f5f5f5; padding:15px; /* rounded corners */ -moz-border-radius:12px; -khtml-border-radius: 12px; -webkit-border-radius: 12px; border-radius:12px; } td{ /* prevent multiline text */ white-space:nowrap; } a, a:visited { color:#00BBFF; text-decoration:none; outline:none; } a:hover{ text-decoration:underline; } h1{ color:#777777; font-size:22px; font-weight:normal; text-transform:uppercase; margin-bottom:5px; } h2{ font-weight:normal; font-size:10px; text-transform:uppercase; color:#aaaaaa; margin-bottom:15px; border-bottom:1px solid #eeeeee; margin-bottom:15px; padding-bottom:10px; } label{ text-transform:uppercase; font-size:10px; font-family:Tahoma,Arial,Sans-serif; } textarea{ color:#404040; font-family:Arial,Helvetica,sans-serif; font-size:12px; } td > button{ /* A special CSS selector that targets non-IE6 browsers */ text-indent:8px; } .error{ /* this class is used if JS is disabled */ background-color:#AB0000; color:white; font-size:10px; font-weight:bold; margin-top:10px; padding:10px; text-transform:uppercase; width:300px; } #loading{ /* the loading gif is hidden on page load */ position:relative; bottom:9px; visibility:hidden; } .tutorial-info{ color:white; text-align:center; padding:10px; margin-top:10px; }
Nothing out of this world here. Notice line 85. This makes the buttons at the bottom of the form wider, but unfortunately they look buggy in IE6. That is why I used a special CSS selector that is ignored in IE6, to target the rest of the browsers.
Now all that is left is the PHP code.
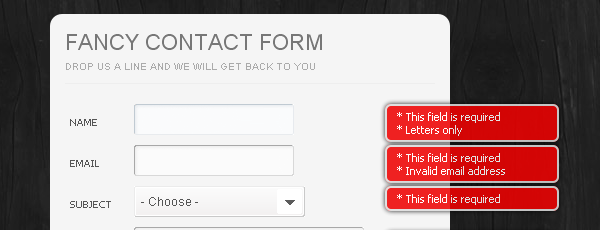
Step 4 - PHP
First lets take a look at the code in the beginning of demo.php.
demo.php
session_name("fancyform"); session_start(); $_SESSION['n1'] = rand(1,20); /* generate the first number */ $_SESSION['n2'] = rand(1,20); /* then the second */ $_SESSION['expect'] = $_SESSION['n1']+$_SESSION['n2']; /* the expected result */ /* the code below is used if JS has been disabled by the user */ $str=''; if($_SESSION['errStr']) /* if submit.php returns an error string in the session array */ { $str='<div class="error">'.$_SESSION['errStr'].'</div>'; unset($_SESSION['errStr']); /* will be shown only once */ } $success=''; if($_SESSION['sent']) { $success='<h1>Thank you!</h1>'; /* the success message */ $css='<style type="text/css">#contact-form{display:none;}</style>'; /* a special CSS rule that hides our form */ unset($_SESSION['sent']); }
As you can see, we use the $_SESSION array to store the two random numbers and the expected result. This is later used in submit.php to confirm the captcha has been solved.
Another interesting moment is line 21 where we define a custom CSS class. This hides the form, so that the only thing shown is the success message in case the visitor has disabled JS.
submit.php
require "phpmailer/class.phpmailer.php"; session_name("fancyform"); /* starting the session */ session_start(); foreach($_POST as $k=>$v) { /* if magic_quotes is enabled, strip the post array */ if(ini_get('magic_quotes_gpc')) $_POST[$k]=stripslashes($_POST[$k]); $_POST[$k]=htmlspecialchars(strip_tags($_POST[$k])); /* escape the special chars */ } $err = array(); /* some error checks */ if(!checkLen('name')) $err[]='The name field is too short or empty!'; if(!checkLen('email')) $err[]='The email field is too short or empty!'; else if(!checkEmail($_POST['email'])) $err[]='Your email is not valid!'; if(!checkLen('subject')) $err[]='You have not selected a subject!'; if(!checkLen('message')) $err[]='The message field is too short or empty!'; /* compare the received captcha code to the one in the session array */ if((int)$_POST['captcha'] != $_SESSION['expect']) $err[]='The captcha code is wrong!'; /* if there are errors */ if(count($err)) { /* if the form was submitted via AJAX */ if($_POST['ajax']) { echo '-1'; } /* else fill the SESSION array and redirect back to the form */ else if($_SERVER['HTTP_REFERER']) { $_SESSION['errStr'] = implode('<br />',$err); $_SESSION['post']=$_POST; header('Location: '.$_SERVER['HTTP_REFERER']); } exit; } /* the email body */ $msg= 'Name: '.$_POST['name'].'<br /> Email: '.$_POST['email'].'<br /> IP: '.$_SERVER['REMOTE_ADDR'].'<br /><br /> Message:<br /><br /> '.nl2br($_POST['message']).' '; $mail = new PHPMailer(); /* using PHPMailer */ $mail->IsMail(); $mail->AddReplyTo($_POST['email'], $_POST['name']); $mail->AddAddress($emailAddress); $mail->SetFrom($_POST['email'], $_POST['name']); $mail->Subject = "A new ".mb_strtolower($_POST['subject'])." from ".$_POST['name']." | contact form feedback"; $mail->MsgHTML($msg); $mail->Send(); unset($_SESSION['post']); /* unsetting */ /* the form was successfully sent */ if($_POST['ajax']) { echo '1'; } else { $_SESSION['sent']=1; if($_SERVER['HTTP_REFERER']) header('Location: '.$_SERVER['HTTP_REFERER']); exit; } /* some helpful functions */ function checkLen($str,$len=2) { return isset($_POST[$str]) && mb_strlen(strip_tags($_POST[$str]),"utf-8") > $len; } function checkEmail($str) { return preg_match("/^[\.A-z0-9_\-\+]+[@][A-z0-9_\-]+([.][A-z0-9_\-]+)+[A-z]{1,4}$/", $str); }
Note how we check whether the $_POST['ajax'] variable has been set and act accordingly. As you remember, we set it back in script.js to indicate we were using AJAX to fetch the data.
The two $_SESSION variables errStr and post are used to share data between the form and submit.php in case JS is disabled. Here post contains the $_POST array we sent and is used to populate the fields of the form, once the user is redirected back.
With this our fancy contact form is finished!
Conclusion
Today we used two great jQuery plugins in conjunction to create a fancy looking contact form. The best thing is that it works in any browser and thanks to graceful degradation you don't even need to have javascript enabled.
You are free to download and modify the code. The only thing needed to run this demo on your own is to put in the email address you want to receive the emails to in submit.php.
*Edit: If you modify the code, try opening submit.php directly in your browser - this will show any possible errors that would remain hidden otherwise. If you run into trouble you can take a look at the comment section - there might be the answer to your problem.
Bootstrap Studio
The revolutionary web design tool for creating responsive websites and apps.
Learn more
Looks pretty and solid. Thanks for sharing.
This looks fantastic! I can definitely use this for my portfolio redesign.
Well Very Fantastic! Thank's
I really liked your blog and bookmarked it
Really cool tutorial, simply excellent!
This is great! Would it be possible to tweak the PHP to have an auto email sent out if you wanted to create an auto email/password form?
here's a dumb question, where would I put the email if I run the demo?
Thanks for the comments.
@a
You can put your email in submit.php. There is an $emailAddress variable on line 5.
@mystro2b
Not quite sure what you mean. But if you want to send an email to the visitor filling in the form, in addition to the one sent to you, you can tweak submit.php. Just duplicate the code between lines 76-86. Will have to change the parameters you provide - e.g. email of the receiver, subject, body etc.
I can't make it send the mail to me. I added: $emailAddress = '[email protected]'; to submit.php
Also the textboxes are just white (no borders in the boxes, just looks like the background. I can write in them though)
-Thanks
continuing the previous comment:
The downloaded demo has the same problems.
and still continuing:
The part of the form is styled with jQuery, only the textfields (name, email, message) are "invisible".
The Captcha seems to not work. It keeps telling me the answer is an invalid number.
Sorry, the specific error is "Wrong verification number!".
Nice form though.
I seem to see a bug when using this demo... if the email address does not end with a .com etc. then the CAPTCHA error pops up stating that the answer is incorrect. but if I add the .com in the email line... then it sends just fine.
The tutorial is pretty good, only one form table ... that's what bothers me, sorry
managed to make it send email, but the textboxes are still not working..
Thanks for the comments.
@ Ryan
I found a bug in the validatorPlugin - it uses a wrong regex to check whether the email is correct. I usually tend not to modify plugins, but in this case I changed the regex to a working one. You can re-download the demo and replace the /formValidator/jquery.validationEngine.js - it will fix your problem.
@ Antti
Looks to me as if the page is unable to load the needed js files.
Are you sure don't apply any htaccess rewrites on your domain?
You could use Firebug to check whether the files are properly loaded and you can change the scripts' src attributes if this is the case.
I just didn't like the use of table to style the HTML. Everything else is perfect.
Very nice work. Easy to implement (AND modify if necessary).
This is great as is or as a starting point for something else. Many thanks to you for sharing this!
Nice form but I can’t send the mail to me.
I added: $emailAddress = ‘[email protected]’; to submit.php and it don't work. I tried an email with dot.com and it still dont work
Anyone can help??
Your blog is awesome man!
awesome !! it's great to see this kind of detailed and useful tutorials
thanks for share as always, i'm gonna check it later... my first impression is really good !
adeux
The same problem((( Where to insert my Email adress?
Thanks for all your comments!
@ Tutorial City
In this case, the form is basically tabular data:
| Field label | Textfield | Error field |
For me, the best way to output tabular data is with a table - that is what it is meant for. You could alternatively use DIVs but you will just end up recreating the row - column structure. This will result in unnecessary markup and will clutter your CSS.
Of course, it is also a matter of personal preference - use the technique you find most convenient.
@ Laury
Is email enabled on your server? You might want to test it by sending an email with PHP's mail() function.
@ Max
You can put your email in submit.php. There is an $emailAddress variable on line 5.
Code for send a copy to the user (You need paste in the file submit.php in the line 88):
$mail = new PHPMailer();
$mail->IsMail();
$mail->AddReplyTo($_POST['email'], $_POST['name']);
$mail->AddAddress($_POST['email']);
$mail->SetFrom($_POST['email'], $_POST['name']);
$mail->Subject = "uno nuevo ".mb_strtolower($_POST['subject'])." from ".$_POST['name']." | contact form feedback";
$mail->MsgHTML($msg);
$mail->Send();
** Spanish ***
Hola amigos, si quereis que el usuario reciba una copia del email, pegar en la linea 88 del archivo submit.php este codigo:
$mail = new PHPMailer();
$mail->IsMail();
$mail->AddReplyTo($_POST['email'], $_POST['name']);
$mail->AddAddress($_POST['email']);
$mail->SetFrom($_POST['email'], $_POST['name']);
$mail->Subject = "uno nuevo ".mb_strtolower($_POST['subject'])." from ".$_POST['name']." | contact form feedback";
$mail->MsgHTML($msg);
$mail->Send();
Hello Martin!
I really like your blog. I'm really new to all this jQuery hype but it looks like it will be a part to all future oages since it adds that little extra spice to make a website go from "cool" to "wow" =)
I have a question though. I want to embed this Fancy Form of yours into my existing page. It seems that the CSS gets a little messed up on the way problably because there are two CSS styles competing for the same tag. One thing is I get an odd strike trough my header (in Firefox), and second thing is that the error popups just adds as plain text at the very bottom of the page and last thing is that I can't seem to mail with it. However if I just upload the demo folder and access it alone it works great.
You can check it out at http://www.cederman.se/mail
Thanks in advance!
Marten
Please I need a code to insert a "CHEKBOXES" and "RADIO BUTTON"
I have one code, but the form talk me "Wrong verification number!"
this is my wrong code (you need also, modify the submit.php):
It just wont mail
Anyway to debug this?
pls help
How do I add a "cc" or "BCC" e-mail to send?
Thx,
Linces Marques from Brazil.
@ Mårten
You can solve the problem by including the contact form's CSS file after that of your site`s main style sheet file.
After this you'll have to modify the form's style sheet to explicitly tell it to disable the unwanted styles. Something like adding text-decoration:none; to prevent strike-through.
From the link you sent me I see that you rewrite the URL so that the form is looking for submit.php in a /mail/ directory on your server, which does not actually exist. There are two solutions, depending on the rewrite rules you use. You could create a real /mail/ directory and put all the form files and images there, or you could put a forward slash '/' before the file paths that are used and put the plugins and folders in your root directory.
@ Doctor Muerte
To put additional form elements, you would have to assign them a class, like the ones in the tutorial above - class="validate[required]". For more info you'll have to consult with the formValidator plugin's homepage.
@ Lunar Wizard
You might have an issue with the file paths, like in Mårten's case. If it is not the situation, you'd have to provide more info, or a URL.
@ Linces Marques
You could just use an additional $mail->AddAddress(); to send the contact form email to more than one recipient.
This looks very nice but why doesn't the "reset" reset the selector, only text fields? Anyone got a solution for that?
cheers, Jan
Thanks Martin, nice job...
Very nice job indeed! It's resources like this that make jQuery more accessible to the javascript newbies like me. :-)
Here is the link
http://totalcoach.com/questions/demoajax/
does not look like a path problem
Hey on my site ive tried intergrating it but the text box jumps right out of the white box :(
Ok fixed that
Now when it closes its like
THANK YOU!!
THANK YOU!!
twice
o.o thank you I will use ><
@ Lunar Wizard
I had the same issue and realized that my host had PHP 4 enabled by default. When this is the case if you go directly to your submit.php in the browser you'll get an error stating that there is an unexpected '}' on line 35 or something like that.
My host gave me the option to switch to PHP v5 and once that finished I refreshed the submit.php page and the error disappeared. I went back to the contact page and tried again and everything worked fine and I received the email.
Try that and see if it works.
Very nice form. I downloaded the source files, added my own email address to line 5 and uploaded it to my server. I can not get it to send email. The addition question always accepts the answer whether right or wrong... Please help, I am not a coder. :)
Hello, Manuel.
Can you provide a URL to the form?
Wow, thanks for sharing this. I like it the way oyu've integrated AJAX into the form - pretty hand! One tiny little problem though - when I supplied my email with dashes in the domain name (just like this one I used for this comment), it doesn't validate.
Other than that, awesome! It's very bookmark worthy indeed :)
Hello, Teddy.
Modified the formValidator plugin again. The problem with your email comes from the two dashes in the domain part - the regex I used does not support that.
Modified it and it is fine now. You can re-download the zip.
Same problem for me...the email address doesnt seem to work..
Hi Martin,
Thanks so much for looking into this. You form is awesome! Hope to get it working :P
http://projectgreenbag.com/demo/demo.php
(oops wrong url) Here is the correct one: http://projectgreenbag.com/contact/
Hi Martin,
Great script and fantastic blog - its now bookmarked. I'm just having a bit of a problem getting my form to process properly. When i click the submit button the form just shows that its loading, but nothing else happens and the form is never sent. I'd really appreciate any help with this. Its seems like it may be a php issue, but i'm not sure.
Thanks,
Andrew
@ Manuel
You've done a good job at styling the form. Will look great once it is up and running. Also I believe I found the possible cause of your problems - several versions of jQuery are included in your page at once. Would it be possible to leave only the one included with the form?
@ Andrew
Hello Andrew. Can you give an URL? There can be many possible causes for your problem.
Martin
I have everything setup correct but not getting the e-mail. I have php experience and can't get this form to my e-mail. I have the email set in submit.php as well. Please help, sites for a friend.
HI
I am building a site in drupal.. I want to integrate this form because it rules..
However I am having trouble. JQuery plugins are a part of the drupal core. All my links are correct in my .tpl.php file specific to /node/4. The form works beautifully in its own dir @ root.
I'm stumped. I'm willing to post markup if you will help Martin.
Thanks,
Patrick
Hi Martin,
Your right! That was the problem. Your amazing! I can fill out the form now, however the math doesn't check to see if the answer is correct nor am I getting emails.
http://projectgreenbag.com/contact/
I'm a beginner in php but after downloading the demo and putting it on my iis, it doesn't seem to work. The captcha doesn't generates, the textboxes are filled with php script and it doesn't resets. Though when having js enabled it makes the validation but the submit doesn't work. HELP me with some tips, and please tell me what am I doing wrong?!
Thanks!
@ Manuel
Found your problem - you are running PHP 4, and the class is for PHP 5. Your host should have given you an option to switch the PHP version that your site uses somewhere in your control panel. Alternatively you can download and include a version of PHPMailer for PHP 4 in place of the current one.
If I knew there would be so much compatibility problems I would have done the form differently. A lesson for future tutorials.
@ Patrick B
Sorry Patrick, I haven't worked with Drupal. You might try in some Drupal support forum.
@ Madalina
It looks to me as if IIS is misinterpreting the short PHP tags that I've used in the form:
<?=$var_name?>
Try replacing them with full tags:
<?php echo $var_name; ?>
Fix the problem! Thanks! Very helpful Now when getting to the next step when submitting the form nothing happens (js disabled), it redirects the page to submit.php and doesn't verifies the form like in the demo posted on your blog. Please give me a hint about what I should do about that.
Thank you very much, I must say I wasn't expecting a response so soon!
Martin, I made the switch to php 5, works like a charm. Wow your amazing! Thanks SO much! :D
Only just recently discovered this site. It's awesome! Plenty of geeky tutorials to fill my spare hours. Fantastic.
Here is my php version : PHP Version 5.2.8
http://totalcoach.com/questions/demoajax/submit.php
No error in the submit page.
No mailing either
'.$_SESSION['errStr'].''; unset($_SESSION['errStr']); } $success=''; if($_SESSION['sent']) { $success='
Thank you!
'; $css=''; unset($_SESSION['sent']); } ?>
i see these above the form.. there's no form... i just download the demo files..please help me ;)
@ Lunar Wizard
I checked your installation - you do not have the mb library installed with PHP. This means you'll have to either install it, or replace the mb_strlen() function with strlen() and removing the second "utf-8" parameter. This should do the trick.
@ monika
Can you give me an URL?
I love this form, looks great.
i specified my email address but when i filled the form i didn't received the email. Could you help please.
URL: jmysona.lap.pl/demo/demo.php
@ Jakub
Thanks for the comment! You are probably running PHP 4 - you'll have to switch your site to PHP 5 for the form to work (there should be an option for this in your hosting control panel).
many thanks Martin
Tnx for this nice script but I have problem to run it. Tested with WampServer Version 2.0 and this is a list of changes which I made, I hope that this would help peoples who use WAMP like me.
Demo.php
Line 13. if(isset($_SESSION['errStr'])) { ...
Line 20. if(isset($_SESSION['sent'])) { ...
Line 63. value=""
Line 68. value=""
Line 84.
Line 88. +
Sorry .
Line 13. if(isset($_SESSION['errStr'])) { …
Line 20. if(isset($_SESSION['sent'])) { …
Line 63. if(isset($_SESSION['post']['name'])) {$_SESSION['post']['name']; } else { ""; }
Line 68. if(isset($_SESSION['post']['email'])) {$_SESSION['post']['email']; } else { ""; }
Line 84. if(isset($_SESSION['post']['message'])) {$_SESSION['post']['message']; } else { ""; }
Line 88. echo $_SESSION['n1']; echo $_SESSION['n2'];
This is by far the best form I've come across in terms of usability and customisation, especially for a designer like me. I have the form working OK but there is one tiny little issue that I can't seem to fix.
The selected subject field option appears in a default styling. I realise that its probably being affected by another css selector from my other css file but I can't figure out what's causing it to appear that way. Any light you could shed on this would be greatly appreciated!
test.simontitchmarsh.co.uk/rhoco/contact.php
I've uploaded the form and tested everything and it was working great, but when I added a "phone" field, it says the email was sent but I don't get an email. Any ideas? I just copied the code from the name field and changed the name and id's and also added the phone to the message of the email. URL: http://www.opticsugarmedia.com/form/demo.php
My IE6 Installation works fine on the demo page except for one important issue: The text fields cannot be filled -- no character typing possible ... Sure, IE6 is somewhat old, I was only curious because I'm happy when the modern Javascripts degrade nicely.
Any one having any luck getting this to work with Godaddy?
Hey
i love this script, looks great!
Only thing is i have a problem adding a checkbox for terms and conditions, when i do and the checkbox is not clicked, the error display is at the top of the form, not next to the input element? I am sure its an easy fix but i have tried and am a bit stumped
Cheers
Davros
Cool. I made this to work on my website. But on IE, when i click the dropdown menu, the input box underneath it overlaps with the dropdown. Where do I fix this? I tried fixing the z-index on jqtransform.css but nothing happens.
@ Dana
Checked the link you provided but everything looks OK. Even a proper status code is returned from submit.php.
You might want to double-check the PHPMailer code you've added in order to send the additional fields.
@ archim
As far as I've tested it, the form works fine in IE6. There might be some CSS that is interfering with the form. Does it work with JS disabed?
@ Andrew
The demo is hosted at Godaddy, so you shouldn't run into any problems.
If you do, however, it might be that you are not running PHP 5. Try opening submit.php directly into your browser to see if there are any errors. If this is the case, you might want to change the PHP version used. This is done from the hosting control panel.
@ Davros
Can you share an URL?
@ Martin
You are fantastic, not only have you created an awesome form but you are also one of the few that likes to backup their work by helping others with their problems!
Excellent work mate! My hat goes off to you!
I always seem to make things difficult. This is a very simple and easy tutorial, but it took me 2 hours to complete. Remember kids follow the directions.
This is probably a 5 minute tutorial if followed properly.
Thanks for this Tutorial
This is just what I was looking for and I am using this on my website now.
Thanks a lot for this excellent tutorial!
hi and thanks for the great tutorial!
when i upload the demo version on my host, the form itself is loading (demo.php) and everything seems fine. But when it comes to captcha validation and recieving the e-mail, the form fails for me.
I get no e-mail and the script seems to ignore the captcha validation.
When i call the submit.php , i get the following message:
Parse error: syntax error, unexpected T_STRING, expecting T_OLD_FUNCTION or T_FUNCTION or T_VAR or '}' in /homepages/16/d209550691/htdocs/demo/phpmailer/class.phpmailer.php on line 53
Hope u can help me out! thanks in advance!
Its me again! I already fixed it, sorry for the comment!
And huge thanks for this nice tutorial :)
Hi Martin,
Awesome form, I'm currently using it at http://lookingforleases.com. After the form sends is there a way to change the URL from index.php to "index.php?src=complete" or have the form send the user to a thank you page. I need to have this in place to track a completed form conversion.
Thank you for your time,
Matt
Hi,
This form is great, and I'd love to implement it on our site, which is running PHP5. Unfortunately, the demo seems to get stuck on a loop when one hits submit: http://creative.promosis.com/newpromosys/demo/demo.php . Not seeing any errors from submit.php.
Any help would be very much appreciate.
@ Jeff
I used firebug and it showed that submit.php fails with a 500 Internal Server Error. I would suggest you try re-uploading it.
@ Matt
Yes, you could add the following code to line 49 of script.js:
window.location='index.php?src=complete';
And it will redirect your visitors to the page specified.
How can I remove the "subject" field?
if I try to desable it in demo.php and submit.php then it doesn't works
Please help
i'm getting the following:
Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent
Hello. Can this be use in wordpress? You see I have a wordpress blog and also I have your jquery plugin on every page of my website and of course I want to put also your jquery form in the footer of my blog as i did in the rest of my pages, But When I do, the only thing that is working is the demo.css because it seems to style the 'name', 'email', 'subject' texts before the input text but everything else fail: it doesn't send, the styles on the text inputs, dropdown, textarea and buttons.
I'm thinking I have some errors on my url but what I did was I uploaded every file, like demo.css, the other .css and the .js on my wp-content/wp-themes where i put all my php files (i.e. index.php, sidebar.php, footer.php) and have all the urls in my header.php like what i did in my main pages on my website.
I was thinking if you can also show us how to make this work on wordpress cuz the wordpress contact form plugin really sucks and it needed to be put on another page and not where I want it to (my footer).
THanks, hope you can reply.
-mark
Hi Martin,
I've re-uploaded it, and checked it with Firebug. Please have another look when you get a chance.
Many thanks.
@ DarioDN
After your remove the subject field from demo.php, you can add the following line to submit.php instead of modifying anything:
$_POST['subject'] = 'message';
Put it at the top of the file.
@ ajey
Looks like something gets outputted before the session_start. If you've included it into another PHP file, make sure the session lines are at the top of the file. If this is not the case please provide a link to your form.
@ Mark
If you are using URL rewriting, like I do here, then the form searches for the rest of the files in a directory that does not exist. For example for this post it would try to reach submit.php in
http://tutorialzine.com/2009/09/fancy-contact-form/submit.php
which does not exist. To make it work you'll have to put all the example files in a folder and put it in your root. Something like this:
http://tutorialzine.com/fancyform/
After this you'll have to edit script.js to search for submit.php in that directory. For example line 39 would become:
$.post('/fancyform/submit.php',$(this).serialize()+'&ajax=1',
The same is true for the contact form's action attribute - should be preceded by /fancyform/.
When this is done and you've changed the include paths of all the CSS & JS files in your blog theme, it should work fine.
@ Jeff
I see the same problem with the 500 error. Do you use any URL rewriting on your site?
Hi,
I have the same problem than Hansen(25/ october 2009) with the error message Parse error: syntax error, unexpected T_STRING, expecting T_OLD_FUNCTION or T_FUNCTION or T_VAR or '}' in /web/.../www/.../media/formJquery/phpmailer/class.phpmailer.php on line 53
He said he solved the issue but didn't tell how.
How can I solve this issue ?
Great script !!
Thanks
Thanks so much for the reply Martin, appreciate it. Will try this.
@ patrick42
This is caused by running the demo on PHP 4. You'll have to switch your site to PHP 5 - there should be an option in your hosting control panel.
Thanks Martin,
I have moved on test the script on Hostgator servers which runs Php5 and the problem has disappeared. But now I have the same problem that Jeff describes : a loop what I hit the submit button.
I have no htaccess file to rewrite urls.
Thank you
Great website. Keep up the good work!
Beautiful Contact form - love ajax , this is so smooth and just easy on the eyes . Great job :)
M.
BTW Martin : I see that youre not only a ROCKIN SUPER CODER ! but you also try to help out your users w/ any problems they may encounter. This is not only admirable , but commendable as well , not many Dev's out there are willing to take the time & make the effort to answer their users questions. You def ROCK ! :)
Nice form, have been playing around with it.
One thing I came across; the error box for the message field does not align. All the others do. Been trying to get it aligned to the message box but no luck so far.
Anyways I am making it more fancy as we speak, building a light box around it so it hides behind a contact button. Makes it a bit less obtrusive. I'll sent you a light box package when I figure out the alignment.
Thanks for your work!
All the best from Amsterdam.
Hi another quick question. How do you remove or add fields without the sent.php breaking.
For example say I wanted to remove the subject field?
$_POST['subject'] = ‘message’; does not work.
http://www.opticsugarmedia.com/403/form/demo.php
I had the form working, it sent an email and everything was good. I changed one of the field names hit refresh and now it always says my verification code is wrong. I un-did all of the previous changes and uploaded them…same problem. Any ideas? Thanks!
great, great. maybe i could use it in the future.
HI, Martin. Great tutorial, and great site as well.
I am trying to implement this on my new website.
Got a question: since I will implement this in a WordPress theme, where the Header stuff is in an include file (header.php), am I supposed to put the PHP chunk of code before the DOCTYPE in there? it will then be there for each and every page. Is that an optimal use of it, or there are other possibilities to include that code?
(I don't know if you're familiar with WordPress, but the principle is the same with nay other CMS).
Thanks.
@ patrick42
It sounds like there is a problem with the paths. Try the solution I suggested to Mark in the comment above.
@ Mia
Thanks for the support! I try to do my best :)
@ gideon
The error boxes align according to the TD with id="errOffset" (line 17 of demo.php in Step 1).
$_POST['subject'] = ‘message’; should work if you put it before the actual check whether this variable is set up (put it somewhere after session_start in submit.php).
@ Gianfranco
You can put the chunk of PHP code on the top of demo.php only on the page that holds your contact form.
Also don't forget to remove the two session_ lines both in demo.php and submit.php - wordpress handles its own sessions.
Thanks Martin!
By "You can put the chunk of PHP code on the top of demo.php only on the page that holds your contact form", do you mean "in the content" (which is like in the entry text, that comes after the header), or still BEFORE the Doctype?
Because, if it's a must fot it to be before the Doctype, since the header section is an include, than I need to create a conditional statement before the Doctype to check if that is the page that contains the form.
Mmm… Or can I put the code in the content, just before the form?
Thanks for your support!
Oh, and thanks for telling me about removing the two session_ lines. Even thought I master WP preatty well, I am not keen yet about WP and Forms.
So, again, thanks.
I've just downloaded and installed the form on a webpage. The form keeps telling me I have the "wrong verification code". I noticed earlier that someone else had this problem but they only had to download a new .js file. I just re-downloaded all of the files from this site yesterday. Any ideas?
http://www.opticsugarmedia.com/403/form/demo.php
Hi Martin,
Beside the above question, I got this issue I'd like to sort out (and from the comments above, I am sure others are interested too).
I'd like to add and implement some checkboxes to the form.
You can find an example at http://stylozero.com/fancyform/demo.php.
Now, I'd like the user to be able to check multiple checkboxes, and obviously I want the choices to appear into the sent email.
So, I customized the code in "submit.php", like this:
/ the email body /
$msg=
'Name: '.$_POST['name'].'
Company: '.$_POST['company'].'
Email: '.$_POST['email'].'
Chosen services:
Consultancy: '.$_POST['consultancy'].'
Management: '.$_POST['management'].'
Design: '.$_POST['design'].'
Coding: '.$_POST['coding'].'
IP: '.$_SERVER['REMOTE_ADDR'].'
Message:
'.nl2br($_POST['message']).'
';
Unfortunately, when I receive the email, I just get the label names for ALL the checkboxes, but nothing that tells that THIS or THAT was selected.
Actually, I can't figure out how to say: "If this checkbox is checked, then write it under "Chosen services", if not, don't write it."
In demo.php for each checkbox I got this (ex. only for Consultancy):
Consultancy
<input type="checkbox" name="consultancy" id="consultancy" value="" />
Something tells me that what I am trying to achive can be done very easily, but it's just out of my range for at this moment.
Can you help me out with this?
Thanks in advance.
;-)
Oops, the PHP was stripped out from the last code. Will this work?
Consultancy
<input type="checkbox" name="consultancy" id="consultancy" value="" />
Hi Martin...
great form!!!
I try to use your form, but this don't send the email... when I send a email from the form, I don't receive the email in my inbox...
I modified the line 5 in submit.php and my server have php5
you can help me??
sorry my english... I only speak spanish :)
Thanks!
Hey, using this as my band's contact form! Is there any way of changing the Captcha to be something a little more, I don't know, difficult? Hah. Awesome tutorial and great cform. Thanks!
This form is BEAUTIFUL! and I love how you pulled the subject from a dropdown into the email.
I also noticed the issue with the CAPTCHA being invalid. This only happened when I typed a ? or a period in the message box. when I type in a real word, like a visitor would, it works every time.
Maybe its just a gmail thing, but the emails end up in my spam folder. Telling gmail its not spam doesnt fix it. Maybe this is the issue others are having when theyre not receiving the emails??? any ideas on how to work around this?
anyway. I love this form! Thank you for sharing!!! I will use it every time with a link back!
Seems lile I am not able to post anymore…
Thanks for this.
The issue I am having is with the verification. While it worked once, regardless of how many times you enter in the correct answer it states it is incorrect.
Is there a suggestion on how to get this working?
Thank you.
@ Dana
I checked the URL you provided. It seems that everything on the form side is OK but something is wrong with the PHP - it always returns '-1' for an error. Maybe there is a problem in your error checks in submit.php?
@ Gianfranco
Yes, you can put the PHP code right before the form itself, in the same file that holds it. No need to modify the header.
As for the second issue - you have to assign a value to the checkboxes. For example:
And in PHP you would:
@ Martin
Can you share a URL?
@ Trisha
I can not replicate the problems you are facing - seems to work fine in the demo here. Maybe you could share a URL?
As for the gmail part, one solution would be to have the form send emails from "[email protected]". It currently sends them from the email of the person that filled the form and gmail might think they are spam because the alleged source of the mail and the real one don't match.
@ Tony
Can you post the URL where you've installed the form?
Hey Martin, thanks for your work.
I only noticed a compatibility problem between jqTransform and validationEngine with radio groups : the bubble goes at the top of the website (y-position not set?).
I don't know how to fix this in the jqTransform plugin so I applyed jqTransform only on ".rowElem" and not on the radio group :
$('.rowElem').jqTransform();
May be it could help someone ;)
PS : moreover, I have 3 radio boxes into my group (all 3 with the same name), and the 3 bubbles display one over the others. It's not a big deal but the opacity "seems" to be different now. I didn't try to fix it yet.
EDIT : seems your script doesn't work anymore with validationEngine v1.6 :(
Great form Martin!
However, I am having very similar problems to what Boba was having on 10/12.
I keep getting errors returned on like 13, 20, 41, 62, 67, and 83.
The session variables seem to be the big issue here.
Hi Martin,
I really admire what you have done here.
I am experiencing the same problem as Greg, Jqtransform and the validation messge don't work well together, validation messages for radio group and combobox don't line up with the input field.
I would also like to know whether it is possilble to add a smtp setting in the php file to overrride the default smtp setting configured on php.ini.
Thank you very much,
Hi grat work and thanks for helping everybody, i have a little problem, when somebody submits an email with underscores it sends me the message "Invalid email address", i saw the regex u used and they are there, do you know what might be causing this?
hi, nice form but what about an upload of a picture added in to this form ?
can you add on it ?
thank you
Hey Martin,
The form works fine on my server, but I transferred it over to the clients server and it acts like it sends ok, but the email never arrives. The tech guy from the server company says "mail server is secured up the ying-yang, and if your script is not using it as the outgoing SMTP server, then incoming mail could very likely wind up getting blocked due to SPF verification failure." Any ideas how to solve this problem? He is also suggested this: "But you could also point it at port 25
on "mail.chqmate.com", which resolves to 192.168.47.42, which is the
mail server that's authoritative for this domain. The mail server has
instructions to also accept mail (not put through spam filtering) if
it comes from 192.168.47.41, which is the IP address of the web
server." If this will work, what PHP code do I change?
Thanks in advance!
Hi Martin, great script its just what im looking for. However, im having a small problem. I have three inputs that i dont want to validate, ie so the form would submit with these being blank. Ive tried removing the input class completely and then adding the class optional: Special as said on the formvalidation site but the form just wont submit. Ive actually got error messages floated in random boxes now :/. Take a look here http://gfxpixeldesigns.com/gfxpixeldesignsnewdesign09/contact.php
And thanks for the great work.
Hello
I have it setup on my website http://www.perthstreetcars.net
It is a Godaddy hosted website running PHP5
There is no errors running submit.php but when you try to submit the contact form it just sits on the loading graphic
Any ideas?
Hi again Martin,
Ive since removed those optional: Special tags and added the class validate[required] back again. Although i would still like to have 3 fields that dont validate, not quite sure how i do this ?
Also after removing the subject field the form no longer submits, ive added $_POST['subject'] = ‘message’; after session_start in submit.php) as you mentioned above however this doesnt seem to work.
Ive uploaded the contents of my contact.php and submit.php here and would greatly appreciate you taking a look (ive removed my email since i dont wnat everyone to see it).
contact.php contents = http://gfxpixeldesigns.com/gfxpixeldesignsnewdesign09/contact.txt
submit.php contents = http://gfxpixeldesigns.com/gfxpixeldesignsnewdesign09/submit.txt
Thanks
Hey Martin, I just managed to fix the issue with removing the subject input. The issue being if you removed the subject input the form wouldnt submit, to resolve this just open the script.js file and remove
[code]if(!$('#subject').val().length)
{
$.validationEngine.buildPrompt(".jqTransformSelectWrapper","* This field is required","error")
return false;
}
[/code]
around line 22. once youve remvoed that you can remove all references off subject from contact.php and submit.php.
I've disabled the contact form until I get this resolved, but here's the link: http://www.chqmate.com/contact.php
Hey,
i have a problem with letters like ö ä ü Ö Ä Ü ß.
You cant use it as name letters. And if used in the message... they look like:
öäüßÖÄÜ
What can i do?
....and I also noticed the issue with the CAPTCHA being invalid.
It happens when i use (for example) an ü in the Name.
And than 20+4= 24 is wrong! I clear the ü and than (sometimes) 24 is ok.... or not!
Hi Martin,
i dont know why you clear my questiones??? Perhaps my english is too bad? :(
I try to explain my problem again!
I want to use european umlauts like öäü.
When i try to fix the jquery.validationEngine.js… with:
"regex":"/^[a-zA-Züöä\ \']+$/",
it dont work! Why?
Iam not a good coder. Perhaps you can help me.
Thanks
Hi... a little step towards solving my problems here:
if I use:"regex":"/^[w-äöüa-zA-Z\ \']+$/",
its possible to use äöü.
But in my mail they look always like: ä ü ö
What can i do?
…and thanks that you havent clear my questions:)
Please anyone tell me what are the free hosting providers to support this method !!! Is 000webhost free hosting account doesn't support this ?
wow, great contact form!
where i can change the width of textarea-field? Name, email, captcha
thx
I tried to get the check boxes working with the comments and snippets and tested many different places to insert the PHP without luck.
Please post it if anyone else was able to get it working.
It was easy to get the check boxes in the HTML to display and also to send the info through but I don't know where to place the conditional statement with the description given.
Thanks for the form, it's great.
But it's bit hard to customize the style, a lot of the values are ruled by javascript (jquery.jqtransform.js)
For example:
I like to make the name field wider, I change
$wrapper.css("width", inputSize+10);
to
$wrapper.css("width", inputSize+100);
it works, but when I type a very long name it turns out ugly (on firefox) because it only shows half of it.
Could someone please enlighten me how to change the field width on CSS? or where is the JS value ('size' jquery.jqtransform.js line 106) taken from?
I'm not a javascript coder and i find it hard to understand why CSS need to be written on JS.
Cheers
I am also having trouble getting rid of the "Subject" part of the form.
I've taken the field out of the demo.php file, and I have also placed
$_POST['subject'] = ‘message’;
after the session_start in submit.php file.
The form will no longer function with that line of code placed in the submit.php file. When you click "Submit" the page just sits there.
Any advice?
Hi. Thanks for perfect tutorial.
In Opera 10 there is a big 'gap' between top border and text in input-box (in MSIE ok). Also all elements are not the same width which is not pretty I think.
My solution is to change jquery.jqtransform.js (but I am not a professional so it is not optimized):
In "text fields" section change 'width' and 'padding' definitions to:
and in "Select" section add after "var newWidth":
I tried to turn off the jqtransform and it causes problems if I write in an email adress that isnt in email format. (throws a server error message form line 98 in the submit.php) the mail is sent alright but the success message isnt displayed.
I know it's weird that the jqtransform does this, but it only happens when I remove it's java script and CSS link from the main php, or remove its folder form the server.
@ Gianfranco
Were you able to ever get this working in a Wordpress theme?
I've got everything styling/linking correctly from a visual standpoint, but the form won't submit in Wordpress for some reason. When I click submit the loading spinner starts, but nothing ever happens.
Martin, if you can add anything to this it'd be greatly appreciated.
Hi.Thank you for great form. But form doesn't work in Wordpress. Please teach us step by step what should we change in code. Thank you!
How to add e-mail address which will be used for mail sending?
Tnx
Hi there
Great script, and just what I'm looking for...I think. I downloaded the code and took a look, and my question is this:
I am creating a very simple contact form, and I would like to eliminate the labels, and pre-load the entry fields with descriptive text.
So for example, remove the "Name" label and put "name" in the entry box itself. When the user clicks the form, the label goes away.
However, I see that you seem to be using the value field for something else.
So would this be possible?
Do reset buttons still have any use on the web?
http://www.useit.com/alertbox/20000416.html
If you ask me.. no =P
works great, thanks.
Hello!
Any way to reset the select boxes? I am looking desperate for a method to reset the select boxes but with no result.
Tnx a lot
Cool, thx !!!
Really Nice Form, Great Work!!!!!
had this form working well until my host upgraded to php 5.3 from 5.2 and now my form wont work, works ok without js but not with, anyone else having the same problems?
thanks
Luke
WOW! This is an insanely great form!
Great Work! Its things like this that make me want to get stuck in to web-design!
Nice background wooden effect to!
Ill be using this on my project
Cheers
how to remove the captcha? what lines do i need to edit ?
i removed from the script.js file and demo.php file but it stops working ...
Hi Martin.
Nice tutorial. There is a problem with the name length verification. If your name is too short (Ed, for example) it'll return a captcha check error. It does this on your demo as well, not just the version I'm bashing together.
Hey i have been using this script for quite awhile now (and its great)
But when i moved the files onto my other server it starting displaying incorrectly.
The 1st server i had it hosted on it was worked perfectly
When i moved it to the 2nd server, this is what happened:
When the page loads the text-box's already have value inserted into them and also the numbers for the captcha aren't showing up.
Here is what it displays in the text-box's :
Name:
Email:
Message:
Does this mean that my new server hasn't got the right version of php to process this script? or does it mean something else.
Please help.
All suggestions much appreciated
Sorry the text didnt show up,
here it is
Name: <?=$_SESSION['post']['name']?>
Email: <?=$_SESSION['post']['email']?>
Message: <?=$_SESSION['post']['message']?>
Hi Martin,
I'm working on adding another fancy form to my site (I already have one working). This is a longer form, so I had to add more fields. However now the form is not sending once I click submit.
I changed the names of each text fields I added to match accordingly, ie: address, city, state, zip, etc.
Then in the submit.php file I added this code for each as well:
if(!checkLen('city'))
$err[]='City Required';
Can you please tell me why each filed is not being validated and why my form will not send when submit is clicked?
http://www.Projectgreenbag.com/sticker/
Many thanks
I've tried a test on my hosting... everything works fine... but i never receive any email from the form... any idea?
Thanks
I got the same error as Joe.. Try replacing out the PHP tags <? with
<?php . It worked for me..
Hello Martin,
I also noticed fancy form stops lightbox galleries from working. I narrowed it down to these 2 conflicting JS files below.
If I remove the fancy form JS, lightbox will work but fancy form will not:
If I remove the lightbox JS, fancy form will work but lightbox will not.
I got the lightbox JS from here:
http://www.huddletogether.com/projects/lightbox2/#overview
Please tell me how to get these two JS to play nice!
http://www.Projectgreenbag.com/sticker/
Thanks,
The fancy form JS that was giving me trouble didn't get posted in my last message. Here it is:
http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js
I don´t know how to get this work in Wordpress. I´ve added the php-part to the header.php, but it still doesn´t work. When I click on "submit" just the word "loading" appears, but I don´t get any mails. Can you help me?
@ Doctor Muerte
Did you ever get this working with radio buttons? I have come pretty close but my validation warnings are being forced to the top of the browser window...
Have you have any success in getting the radio buttons working?
if you have any luck please do share buddy!
Thx
it introduced me to great plugins..:) good work